For this assignment, you will use the Visual Studio Code (VS Code) IDE to compile and run a C# program. Your application will demonstrate the use of inheritance by creating software classes that represent modes of transportation. Incorporate the following requirements into your application: For all classes, provide the following Documentation at the top of the class file that includes Your name Date of development Description of the class Class properties for each attribute listed with the class with public get and set accessors Override of the ToString method to provide formatted string representation of the class properties The main application must do the following Print a line that states "Your Name - Week 1 Inheritance Performance Assessment" Create an instance of each class using parameters of your choosing Print each class's properties to the console using the ToString method Print the following for your instance of the Car class using the get accessors (in this order): Color Number of Seats Engine Size Whether or not it has an Automatic transmission Whether or not it has a Sun Roof Storage Capacity size Class Vehicle will be the overall base class and will have the properties and methods described below Wheels - type integer, the number of wheels the vehicle has Color - type string, the color of the vehicle Moving - type Boolean, indicator of whether or not the vehicle is currently moving Seats - type integer, the number of seats available in the vehicle Class Motorized is derived from Vehicle and will have the properties described below Engine - type string, description of the engine (e.g. 2.6L V6) Automatic - type Boolean, indicator of automatic or manual transmission In the ToString override, DO NOT use the base class's ToString method - use the base class's property accessors to output the base class's data Class Bicycle is derived from Vehicle and will have the properties described below Gears - type integer, the number of gears the bicycle has Seat Height - type double, the height of the bicycle Tire Size - type double, the size of the bicycle's tires in the ToString override, use the base class's ToString method to output the base class's data Class Car is derived from Motorized and will have the properties described below Sun Roof - type Boolean, indicator of whether or not the car has a sunroof Storage - type string, describes the storage area the car has (e.g. None, Small Trunk, etc.) Class Truck is derived from Motorized and will have the properties described below Load - type string, describes the capacity the truck can carry (e.g. 2 ton, long bed) Towing - type Boolean, indicator of whether or not the truck is capable of towing
For this assignment, you will use the Visual Studio Code (VS Code) IDE to compile and run a C# program. Your application will demonstrate the use of inheritance by creating software classes that represent modes of transportation. Incorporate the following requirements into your application: For all classes, provide the following Documentation at the top of the class file that includes Your name Date of development Description of the class Class properties for each attribute listed with the class with public get and set accessors Override of the ToString method to provide formatted string representation of the class properties The main application must do the following Print a line that states "Your Name - Week 1 Inheritance Performance Assessment" Create an instance of each class using parameters of your choosing Print each class's properties to the console using the ToString method Print the following for your instance of the Car class using the get accessors (in this order): Color Number of Seats Engine Size Whether or not it has an Automatic transmission Whether or not it has a Sun Roof Storage Capacity size Class Vehicle will be the overall base class and will have the properties and methods described below Wheels - type integer, the number of wheels the vehicle has Color - type string, the color of the vehicle Moving - type Boolean, indicator of whether or not the vehicle is currently moving Seats - type integer, the number of seats available in the vehicle Class Motorized is derived from Vehicle and will have the properties described below Engine - type string, description of the engine (e.g. 2.6L V6) Automatic - type Boolean, indicator of automatic or manual transmission In the ToString override, DO NOT use the base class's ToString method - use the base class's property accessors to output the base class's data Class Bicycle is derived from Vehicle and will have the properties described below Gears - type integer, the number of gears the bicycle has Seat Height - type double, the height of the bicycle Tire Size - type double, the size of the bicycle's tires in the ToString override, use the base class's ToString method to output the base class's data Class Car is derived from Motorized and will have the properties described below Sun Roof - type Boolean, indicator of whether or not the car has a sunroof Storage - type string, describes the storage area the car has (e.g. None, Small Trunk, etc.) Class Truck is derived from Motorized and will have the properties described below Load - type string, describes the capacity the truck can carry (e.g. 2 ton, long bed) Towing - type Boolean, indicator of whether or not the truck is capable of towing
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
For this assignment, you will use the Visual Studio Code (VS Code) IDE to compile and run a C# program.
Your application will demonstrate the use of inheritance by creating software classes that represent modes of transportation. Incorporate the following requirements into your application:
- For all classes, provide the following
- Documentation at the top of the class file that includes
- Your name
- Date of development
- Description of the class
- Class properties for each attribute listed with the class with public get and set accessors
- Override of the ToString method to provide formatted string representation of the class properties
- Documentation at the top of the class file that includes
- The main application must do the following
- Print a line that states "Your Name - Week 1 Inheritance Performance Assessment"
- Create an instance of each class using parameters of your choosing
- Print each class's properties to the console using the ToString method
- Print the following for your instance of the Car class using the get accessors (in this order):
- Color
- Number of Seats
- Engine Size
- Whether or not it has an Automatic transmission
- Whether or not it has a Sun Roof
- Storage Capacity size
- Class Vehicle will be the overall base class and will have the properties and methods described below
- Wheels - type integer, the number of wheels the vehicle has
- Color - type string, the color of the vehicle
- Moving - type Boolean, indicator of whether or not the vehicle is currently moving
- Seats - type integer, the number of seats available in the vehicle
- Class Motorized is derived from Vehicle and will have the properties described below
- Engine - type string, description of the engine (e.g. 2.6L V6)
- Automatic - type Boolean, indicator of automatic or manual transmission
- In the ToString override, DO NOT use the base class's ToString method - use the base class's property accessors to output the base class's data
- Class Bicycle is derived from Vehicle and will have the properties described below
- Gears - type integer, the number of gears the bicycle has
- Seat Height - type double, the height of the bicycle
- Tire Size - type double, the size of the bicycle's tires
- in the ToString override, use the base class's ToString method to output the base class's data
- Class Car is derived from Motorized and will have the properties described below
- Sun Roof - type Boolean, indicator of whether or not the car has a sunroof
- Storage - type string, describes the storage area the car has (e.g. None, Small Trunk, etc.)
- Class Truck is derived from Motorized and will have the properties described below
- Load - type string, describes the capacity the truck can carry (e.g. 2 ton, long bed)
- Towing - type Boolean, indicator of whether or not the truck is capable of towing

Transcribed Image Text:Your Name - Week 1 Inheritance Performance Assessment
Color: Red
Wheel Count: 3
Seat Count: 2
Currently Moving: False
Color: Blue
Wheel Count: 2
Seat Count: 1
Currently Moving: True
Number of Gears: 12
Bicycle Height: 36
Color: Orange
Wheel Count: 4
Seat Count: 2
Currently Moving: False
Engine: 3.6L V6
Automatic Transmission? True
Color: Maroon
Wheel Count: 4
Seat Count: 5
Currently Moving: False
Engine: 2.4L 4-CYL
Automatic Transmission? False
Storage: None
Has Sunroof? True
Color: Black
Wheel Count: 6
Seat Count: 6
Currently Moving: True
Engine: V8 Hemi
Automatic Transmission? False
Color: Maroon
# of Seats: 5
Engine Size: 2.4L 4-CYL
Automatic Transmission? False
Has Sunroof? True
Storage: None

Transcribed Image Text:UML Diagram
Bicycle
+Gears: integer
+SeatHeight: double
+TireSize: double
Vehicle
+Wheels: integer
+Color: string
+Moving: boolean
+Seats: integer
Car
Inheritance
+SunRoof: boolean
+Storage: string
Week1 PA Inheritance Diagram
Motorized
+Engine: string
+Automatic: boolean
Truck
+Load: string
+TowingEnabled: boolean
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
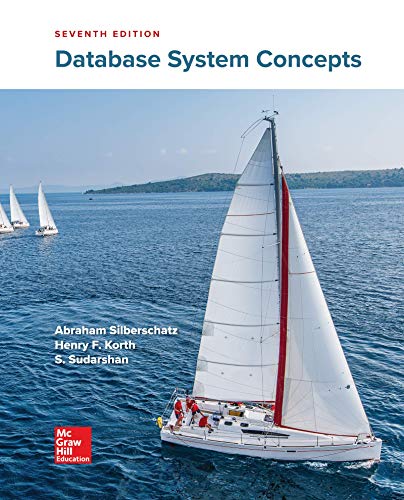
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
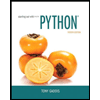
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
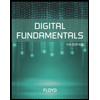
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
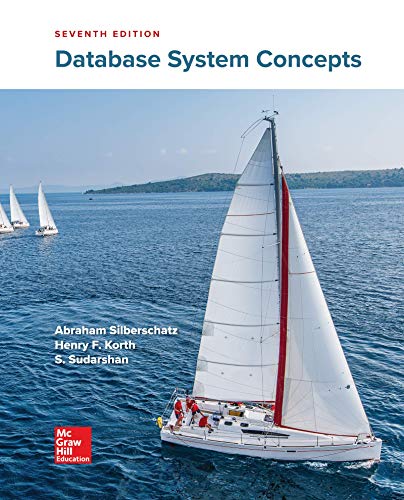
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
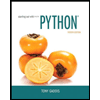
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
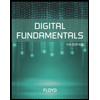
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
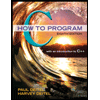
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
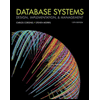
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
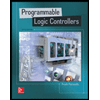
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education