For this assignment, you will be coding a MinHeap that is backed by an array of contiguous elements. Here is a tree and array representation of the same MinHeap: IMPORTANT: • You will be given 5 attempts on this assignment, with a 30 minute cooldown between submissions. • Please run your code before each submission to ensure that there are no formatting errors! If there are formatting errors in your code, your code will not be graded and a submission attempt will be logged. For more information, please review the Vocareum overview below. Properties A MinHeap is a type of binary tree with two main properties: Shape Property: The tree must be complete. All levels of the tree must be full except the bottom-most level. If the bottom-most level is not full, it must be filled from left to right with no gaps. • Order Property: Each node's data is smaller than the data in its children. There is no explicit relationship between sibling nodes. These properties guarantee that the smallest element in the heap will be at the root of the heap. Implementation Details Although heaps are usually classified as a type of tree, they are commonly implemented using an array due to their completeness. In your implementation, you should leave index 0 empty and begin your heap at index 1. This will make the arithmetic for finding parent and children indices simpler. When resizing your backingArray, double the current capacity. Therefore, if the initial capacity is 13, the first resize should bring the total backingArray capacity to 26. Note that this includes the oth index! You may assume that your implementation does not need to handle duplicate elements. That is, the add method will never be passed duplicates and the remove method will never have to deal with the heap having duplicates. To be clear, your implementation would most likely work even if we were to test for duplicates; however, this will help remove ambiguity surrounding grading and testing your implementation. Unlike your BST homework, you are not required to use recursion in this assignment. Use whatever you find most intuitive - recursion, iteration, or both. However, regardless of the technique you use, make sure to meet the efficiency requirements as discussed in this course.
For this assignment, you will be coding a MinHeap that is backed by an array of contiguous elements. Here is a tree and array representation of the same MinHeap: IMPORTANT: • You will be given 5 attempts on this assignment, with a 30 minute cooldown between submissions. • Please run your code before each submission to ensure that there are no formatting errors! If there are formatting errors in your code, your code will not be graded and a submission attempt will be logged. For more information, please review the Vocareum overview below. Properties A MinHeap is a type of binary tree with two main properties: Shape Property: The tree must be complete. All levels of the tree must be full except the bottom-most level. If the bottom-most level is not full, it must be filled from left to right with no gaps. • Order Property: Each node's data is smaller than the data in its children. There is no explicit relationship between sibling nodes. These properties guarantee that the smallest element in the heap will be at the root of the heap. Implementation Details Although heaps are usually classified as a type of tree, they are commonly implemented using an array due to their completeness. In your implementation, you should leave index 0 empty and begin your heap at index 1. This will make the arithmetic for finding parent and children indices simpler. When resizing your backingArray, double the current capacity. Therefore, if the initial capacity is 13, the first resize should bring the total backingArray capacity to 26. Note that this includes the oth index! You may assume that your implementation does not need to handle duplicate elements. That is, the add method will never be passed duplicates and the remove method will never have to deal with the heap having duplicates. To be clear, your implementation would most likely work even if we were to test for duplicates; however, this will help remove ambiguity surrounding grading and testing your implementation. Unlike your BST homework, you are not required to use recursion in this assignment. Use whatever you find most intuitive - recursion, iteration, or both. However, regardless of the technique you use, make sure to meet the efficiency requirements as discussed in this course.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:For this assignment, you will be coding a MinHeap that is backed by an array of contiguous elements. Here is a tree and
array representation of the same MinHeap:
IMPORTANT:
• You will be given 5 attempts on this assignment, with a 30 minute cooldown between submissions.
• Please run your code before each submission to ensure that there are no formatting errors! If there are
formatting errors in your code, your code will not be graded and a submission attempt will be logged. For
more information, please review the Vocareum overview below.
Properties
A MinHeap is a type of binary tree with two main properties:
• Shape Property: The tree must be complete. All levels of the tree must be full except the bottom-most level.
If the bottom-most level is not full, it must be filled from left to right with no gaps.
• Order Property: Each node's data is smaller than the data in its children. There is no explicit relationship
between sibling nodes.
These properties guarantee that the smallest element in the heap will be at the root of the heap.
Implementation Details
Although heaps are usually classified as a type of tree, they are commonly implemented using an array due to
their completeness. In your implementation, you should leave index 0 empty and begin your heap at index 1.
This will make the arithmetic for finding parent and children indices simpler.
When resizing your backingArray, double the current capacity. Therefore, if the initial capacity is 13, the first
resize should bring the total backingArray capacity to 26. Note that this includes the Oth index!
You may assume that your implementation does not need to handle duplicate elements. That is, the add
method will never be passed duplicates and the remove method will never have to deal with the heap having
duplicates. To be clear, your implementation would most likely work even if we were to test for duplicates;
however, this will help remove ambiguity surrounding grading and testing your implementation.
Unlike your BST homework, you are not required to use recursion in this assignment. Use whatever you find
most intuitive - recursion, iteration, or both. However, regardless of the technique you use, make sure to
meet the efficiency requirements as discussed in this course.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
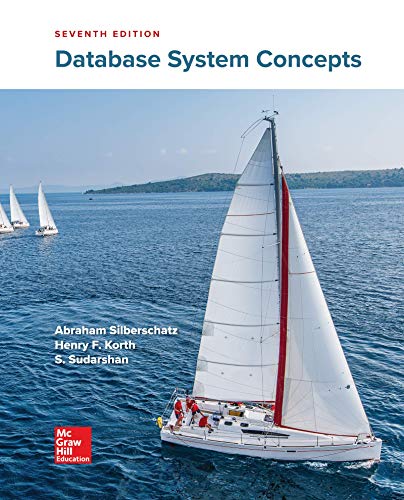
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
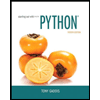
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
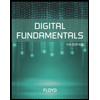
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
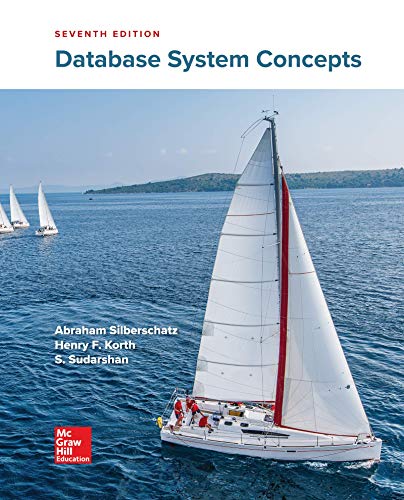
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
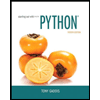
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
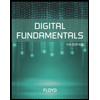
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
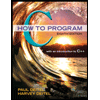
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
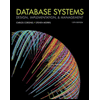
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
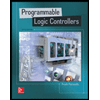
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education