For this assignment, create a new program that will be similar to Assignment #17. Make modifications so that your new program produces the following graph. It should match this graph exactly: by YOUR NAME GOES HERE 16628 15380 12710 10915 4920 4211 3861 Lenovo HP Inc. Dell Apple ASUS Acer Group Others Worldwide PC Vendor Unit Shipment Estimates for 4Q18 (in thousands of units shipped) Be sure to include comments at the top of your Python program with your name and other descriptive information
For this assignment, create a new program that will be similar to Assignment #17. Make modifications so that your new program produces the following graph. It should match this graph exactly: by YOUR NAME GOES HERE 16628 15380 12710 10915 4920 4211 3861 Lenovo HP Inc. Dell Apple ASUS Acer Group Others Worldwide PC Vendor Unit Shipment Estimates for 4Q18 (in thousands of units shipped) Be sure to include comments at the top of your Python program with your name and other descriptive information
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
First picture is assignment I need help with, second picture is my code from assignment 17. Using python
![This image contains a Python script using the `turtle` graphics library. The script appears to draw a bar chart representing the number of first-language speakers for five major languages. Here's a breakdown of the code:
```python
import turtle
heights = [856, 420, 360, 260, 205]
def main():
t = turtle.Turtle()
t.hideturtle()
for i in range(5):
drawFilledRectangle(t, -200+i*75, 0, 75, heights[i]/4, "black", "light blue")
displayText(t)
# Draws a filled rectangle with bottom left corner (x, y)
# With height h and width w, colorPen color, and colorFill color
def drawFilledRectangle(t, x, y, w, h, colorP, colorF):
t.pencolor(colorP)
t.fillcolor(colorF)
t.up()
t.goto(x, y)
t.down()
t.begin_fill()
t.goto(x+w, y)
t.goto(x+w, y+h)
t.goto(x, y+h)
t.goto(x, y)
t.end_fill()
def displayText(t):
languages = ["Mandarin", "Spanish", "English", "Hindi", "Bengali"]
t.pencolor("Blue")
t.up()
for i in range(5):
# Display numbers for each rectangle
t.goto(-200+38+(75*i), heights[i]/4)
t.write(str(heights[i]), align='center', font=("Arial", 10, 'normal'))
# Display languages for each rectangle
t.goto(-200+38+(75*i), 5)
t.write(languages[i], align='center', font=("Arial", 10, 'normal'))
# Display title below chart
t.goto(-200, -25)
t.write("Principal Languages of the World", align='left', font=("Arial", 10, 'normal'))
t.goto(-200, -40)
t.write("(in millions of 'first language' speakers)", align='left', font=("Arial", 10, 'normal'))
main()
```
### Explanation:
- **Imports and Variables**: The script imports the `turtle` module and sets the `heights` list, representing the number of speakers in millions](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F63588563-b3b2-4480-9fdb-3701254cc374%2F95ea5064-49eb-4515-b7fc-60af3212a873%2Fwu168g_processed.jpeg&w=3840&q=75)
Transcribed Image Text:This image contains a Python script using the `turtle` graphics library. The script appears to draw a bar chart representing the number of first-language speakers for five major languages. Here's a breakdown of the code:
```python
import turtle
heights = [856, 420, 360, 260, 205]
def main():
t = turtle.Turtle()
t.hideturtle()
for i in range(5):
drawFilledRectangle(t, -200+i*75, 0, 75, heights[i]/4, "black", "light blue")
displayText(t)
# Draws a filled rectangle with bottom left corner (x, y)
# With height h and width w, colorPen color, and colorFill color
def drawFilledRectangle(t, x, y, w, h, colorP, colorF):
t.pencolor(colorP)
t.fillcolor(colorF)
t.up()
t.goto(x, y)
t.down()
t.begin_fill()
t.goto(x+w, y)
t.goto(x+w, y+h)
t.goto(x, y+h)
t.goto(x, y)
t.end_fill()
def displayText(t):
languages = ["Mandarin", "Spanish", "English", "Hindi", "Bengali"]
t.pencolor("Blue")
t.up()
for i in range(5):
# Display numbers for each rectangle
t.goto(-200+38+(75*i), heights[i]/4)
t.write(str(heights[i]), align='center', font=("Arial", 10, 'normal'))
# Display languages for each rectangle
t.goto(-200+38+(75*i), 5)
t.write(languages[i], align='center', font=("Arial", 10, 'normal'))
# Display title below chart
t.goto(-200, -25)
t.write("Principal Languages of the World", align='left', font=("Arial", 10, 'normal'))
t.goto(-200, -40)
t.write("(in millions of 'first language' speakers)", align='left', font=("Arial", 10, 'normal'))
main()
```
### Explanation:
- **Imports and Variables**: The script imports the `turtle` module and sets the `heights` list, representing the number of speakers in millions

Transcribed Image Text:**Assignment Description for Educational Website**
---
**Title: Language and PC Vendor Data Visualization**
---
**Introduction:**
In this assignment, you will refine your skills in data visualization using Python. Building on the concepts learned in Assignment #17, you will create a program to generate specific graphs accurately.
**Graph 1: Principal Languages of the World**
- **Description:** This graph displays the number of first-language speakers (in millions) for five of the world's principal languages.
- **Languages and Values:**
- **Mandarin:** 856 million
- **Spanish:** 420 million
- **English:** 360 million
- **Hindi:** 260 million
- **Bengali:** 205 million
**Graph 2: Worldwide PC Vendor Unit Shipment Estimates for Q4 2018**
- **Description:** This bar graph illustrates the estimated number of units shipped (in thousands) by various PC vendors during the fourth quarter of 2018.
- **Vendors and Values:**
- **Lenovo:** 16,628 thousand units
- **HP Inc.:** 15,380 thousand units
- **Dell:** 10,915 thousand units
- **Apple:** 4,920 thousand units
- **ASUS:** 4,211 thousand units
- **Acer Group:** 3,861 thousand units
- **Others:** 12,710 thousand units
**Instructions:**
- Create a new Python program based on the guidelines of Assignment #17.
- Modify your code to generate a graph that matches the "Worldwide PC Vendor Unit Shipment Estimates" chart exactly.
- Ensure your program includes comments at the top with your name and other descriptive information.
- Double-check that the visual alignment, colors, and text details are precise.
---
**End of Assignment**
**Note:** Pay careful attention to graph styling and data accuracy for the best results.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
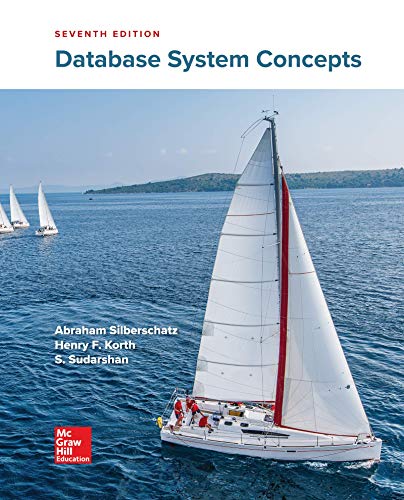
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
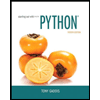
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
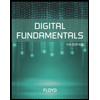
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
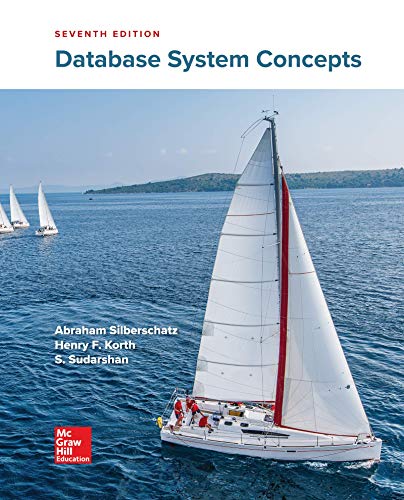
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
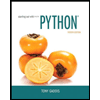
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
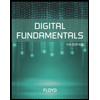
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
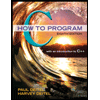
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
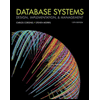
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
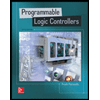
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education