for the help, but I'm not finished yet. Here are my instructions for my C++ program: Write a program that uses the function isPalindrome given in Example 6-6 (Palindrome). Test your program on the following strings: madam, abba, 22, 67876, 444244, trymeuemyrt Modify the function isPalindrome of Example 6-6 so that when determining whether a string is a palindrome, cases are ignored, that is, uppercase and lowercase letters are considered the same.
Code of the following but incorrect attach sa. Not to remove any thing elaww.
Thanks for the help, but I'm not finished yet. Here are my instructions for my C++ program:
Write a program that uses the
function isPalindrome given in
Example 6-6 (Palindrome). Test
your program on the following
strings:
madam, abba, 22, 67876, 444244, trymeuemyrt
Modify the function isPalindrome
of Example 6-6 so that when
determining whether a string is a
palindrome, cases are ignored, that
is, uppercase and lowercase letters
are considered the same.
The isPalindrome function from
Example 6-6 has been included
below for your convenience.
bool isPalindrome(string str)
{
int length = str.length();
for (int i = 0; i < length / 2; i++) {
if (str[i] != str[length – 1 – i]) {
return false;
} // if
} // for loop
return true;
}// isPalindrome
Your program should print a
message indicating if a string is a
palindrome:
madam is a palindrome
Here's what I have so far:
//Include the neccessary header files.
#include <iostream>
#include <string.h>
#include <algorithm>
//Declare the neccessary namespace statements.
using namespace std;
//Define the function
bool isPalindrome(string str)
{
//Compute the string length
int len = str.length();
//Iterate till the middle of the string
for (int m = 0; m < len / 2; m++)
{
//Check the condition
if (str[m]) != str[len - 1 - m])
{
//if for loop returns false
return false;
}
}
//if for loop returns true
return true;
}
//Define the main function
int main()
{
//Declare the variable
string str;
//Prompt the user to enter the string
cout <<"Enter the string: ";
//Get the input string
cin >> str;
//Iterate through the characters in a string
for_each (str.begin(), str.end(), [] (char & ch) {
//Convert the letter into lower-case
ch = ::tolower(ch);
});
//Assign the value returned from the function call
bool s = isPalindrome(str);
//Check if the value is true
if (s == true)
//Print the message
cout << str <<" is a palindrome";
//Otherwise
else
//Print the message
cout << str <<" is not a palindrome";
return 0;
}
As you can see from my screenshot I have 1 error in my program and my exercise is incomplete. According to the complier my only error is on line 18. So in need help fixing any sytax errors.
Also, according to the lab environment (see 6-1a.Capture.PNG), on line 48, the bool expression doesn't cover any of the string values. The lab values still require that I have the following string patterns in my lab:
.+*isPalindrome\(\"Madam\"\).+*
.+*isPalindrome\(\"abBa\"\).+*
.+*isPalindrome\(\"22\"\).+*
.+*isPalindrome\(\"67876\"\).+*
.+*isPalindrome\(\"444244\"\).+*
.+*isPalindrome\(\"trYmeuemyRT\"\).+*
Finally, the program doesn't display whether any of the string values are palindromes or not. Can anyone help?.... L

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

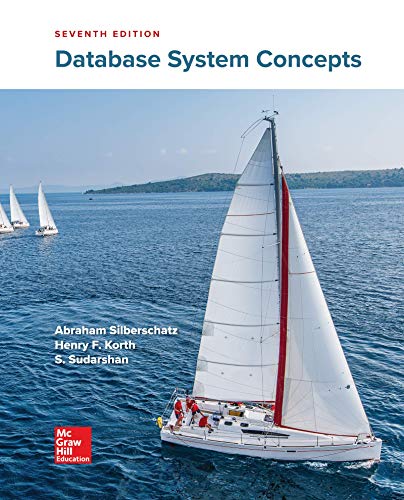
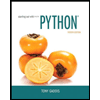
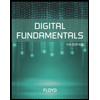
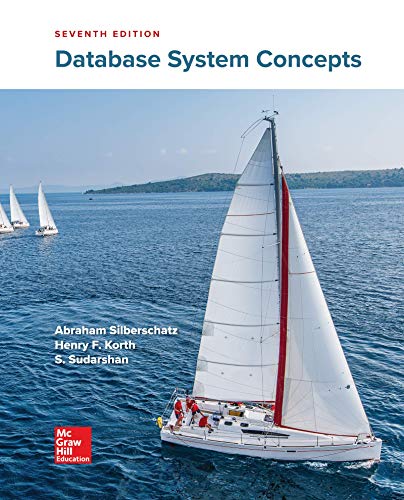
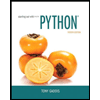
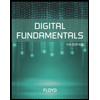
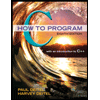
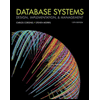
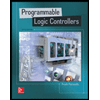