What would a UML Diagram look like for the program written below. Please include the "Shape", "Sphere", "Cylinder", "Cone" and the "shapeArray" classes. I still do not fully understand and I am trying to learn (first time creating one). Please and thank you! Source Code: package application; //abstract class "Shape" public abstract class Shape { //Declare two abstract methods publicabstractdouble surfaceArea(); //Calculate surface area of shape publicabstractdouble volume(); //Calculate volume of shape } package application; //class named "Sphere" that extends the "Shape" class public class Sphere extends Shape { //Attribute radius privatedoubleradius; // Constructor to initialize the sphere with a given radius public Sphere(doubleradius) { this.radius = radius; } @Override publicdouble surfaceArea() { return 4 * Math.PI * Math.pow(radius, 2); } @Override publicdouble volume() { return (4 / 3.0) * Math.PI * Math.pow(radius, 3); } @Override public String toString() { return"Sphere - Surface Area: " + surfaceArea() + ", Volume: " + volume(); } } package application; //Class named "Cylinder" that extends the "Shape" class public class Cylinder extends Shape { //Attributes non-changeable privatefinaldoubleradius; privatefinaldoubleheight; privatestaticfinaldoublePI = Math.PI; // Constructor to initialize the radius and height attributes public Cylinder(doubleradius, doubleheight) { this.radius = radius; this.height = height; } // Overriding the surfaceArea() method to calculate the surface area of the cylinder. @Override publicdouble surfaceArea() { return (2 * PI * radius * height) + (2 * PI * Math.pow(radius, 2)); } // Overriding the volume() method to calculate the volume of the cylinder. @Override publicdouble volume() { returnPI * Math.pow(radius, 2) * height; } // toString() method returning the surface area and volume of the cylinder as a string @Override public String toString() { return"\nCylinder\n\tSurface Area: " + surfaceArea() + " sq.m\n\tVolume: " + volume() + " cubic m."; } } package application; //Class named "Cone" that extends the "Shape" class public class Cone extends Shape { //Attributes non-changeable privatefinaldoubleradius; privatefinaldoubleheight; privatestaticfinaldoublePI = Math.PI; // Constructor to initialize the radius and height attributes public Cone(doubleradius, doubleheight) { this.radius = radius; this.height = height; } // Overriding the surfaceArea() method to calculate the surface area of the cone. @Override publicdouble surfaceArea() { doubleslantHeight = Math.sqrt(Math.pow(radius, 2) + Math.pow(height, 2)); returnPI * radius * (radius + slantHeight); } // Overriding the volume() method to calculate the volume of the cone. @Override publicdouble volume() { return (1 / 3.0) * PI * Math.pow(radius, 2) * height; } // toString() method returning the surface area and volume of the cone as a string @Override public String toString() { return"\nCone\n\tSurface Area: " + surfaceArea() + " sq.m\n\tVolume: " + volume() + " cubic m."; } } package application; //Java GUI FX imports import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.stage.Stage; //Driver class ShapeArray public class ShapeArray extends Application { //Entry point for JavaFX application publicvoid start(Stage primaryStage) { // Creating instances for the different shapes: Sphere, Cylinder & Cone Sphere sphere = new Sphere(4); Cylinder cylinder = new Cylinder(2, 6); Cone cone = new Cone(5, 8); // Storing the shape instances in an array Shape[] shapeArray = {sphere, cylinder, cone}; // Creating a vertical box layout VBox root = new VBox(); root.setPadding(new Insets(10)); // Iterating/looping through the shape array and creating labels for each shape's calculated data for (Shape shape : shapeArray) { Label shapeLabel = new Label(shape.toString()); root.getChildren().add(shapeLabel); } // Creating a scene with the layout and setting it to the stage Scene scene = new Scene(root, 300, 200); primaryStage.setScene(scene); primaryStage.setTitle("Shapes Surface Area & Volume Calculator"); primaryStage.show(); } // Launching application publicstaticvoid main(String[] args) { launch(args); } }
What would a UML Diagram look like for the program written below. Please include the "Shape", "Sphere", "Cylinder", "Cone" and the "shapeArray" classes. I still do not fully understand and I am trying to learn (first time creating one). Please and thank you!
Source Code:
package application;
//abstract class "Shape"
public abstract class Shape {
//Declare two abstract methods
publicabstractdouble surfaceArea(); //Calculate surface area of shape
publicabstractdouble volume(); //Calculate volume of shape
}
package application;
//class named "Sphere" that extends the "Shape" class
public class Sphere extends Shape {
//Attribute radius
privatedoubleradius;
// Constructor to initialize the sphere with a given radius
public Sphere(doubleradius) {
this.radius = radius;
}
@Override
publicdouble surfaceArea() {
return 4 * Math.PI * Math.pow(radius, 2);
}
@Override
publicdouble volume() {
return (4 / 3.0) * Math.PI * Math.pow(radius, 3);
}
@Override
public String toString() {
return"Sphere - Surface Area: " + surfaceArea() + ", Volume: " + volume();
}
}
package application;
//Class named "Cylinder" that extends the "Shape" class
public class Cylinder extends Shape {
//Attributes non-changeable
privatefinaldoubleradius;
privatefinaldoubleheight;
privatestaticfinaldoublePI = Math.PI;
// Constructor to initialize the radius and height attributes
public Cylinder(doubleradius, doubleheight) {
this.radius = radius;
this.height = height;
}
// Overriding the surfaceArea() method to calculate the surface area of the cylinder.
@Override
publicdouble surfaceArea() {
return (2 * PI * radius * height) + (2 * PI * Math.pow(radius, 2));
}
// Overriding the volume() method to calculate the volume of the cylinder.
@Override
publicdouble volume() {
returnPI * Math.pow(radius, 2) * height;
}
// toString() method returning the surface area and volume of the cylinder as a string
@Override
public String toString() {
return"\nCylinder\n\tSurface Area: " + surfaceArea() + " sq.m\n\tVolume: " + volume() + " cubic m.";
}
}
package application;
//Class named "Cone" that extends the "Shape" class
public class Cone extends Shape {
//Attributes non-changeable
privatefinaldoubleradius;
privatefinaldoubleheight;
privatestaticfinaldoublePI = Math.PI;
// Constructor to initialize the radius and height attributes
public Cone(doubleradius, doubleheight) {
this.radius = radius;
this.height = height;
}
// Overriding the surfaceArea() method to calculate the surface area of the cone.
@Override
publicdouble surfaceArea() {
doubleslantHeight = Math.sqrt(Math.pow(radius, 2) + Math.pow(height, 2));
returnPI * radius * (radius + slantHeight);
}
// Overriding the volume() method to calculate the volume of the cone.
@Override
publicdouble volume() {
return (1 / 3.0) * PI * Math.pow(radius, 2) * height;
}
// toString() method returning the surface area and volume of the cone as a string
@Override
public String toString() {
return"\nCone\n\tSurface Area: " + surfaceArea() + " sq.m\n\tVolume: " + volume() + " cubic m.";
}
}
package application;
//Java GUI FX imports
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
//Driver class ShapeArray
public class ShapeArray extends Application { //Entry point for JavaFX application
publicvoid start(Stage primaryStage) {
// Creating instances for the different shapes: Sphere, Cylinder & Cone
Sphere sphere = new Sphere(4);
Cylinder cylinder = new Cylinder(2, 6);
Cone cone = new Cone(5, 8);
// Storing the shape instances in an array
Shape[] shapeArray = {sphere, cylinder, cone};
// Creating a vertical box layout
VBox root = new VBox();
root.setPadding(new Insets(10));
// Iterating/looping through the shape array and creating labels for each shape's calculated data
for (Shape shape : shapeArray) {
Label shapeLabel = new Label(shape.toString());
root.getChildren().add(shapeLabel);
}
// Creating a scene with the layout and setting it to the stage
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Shapes Surface Area & Volume Calculator");
primaryStage.show();
}
// Launching application
publicstaticvoid main(String[] args) {
launch(args);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

For the diagram, are the classes "Sphere", "Cone", and "Cylinder" under the class "Shape"? And then the class "shapeArray" is under those classes? Is that how they all relate to one another? I have attached a rough UML diagram for clarity as to what I am trying to describe.
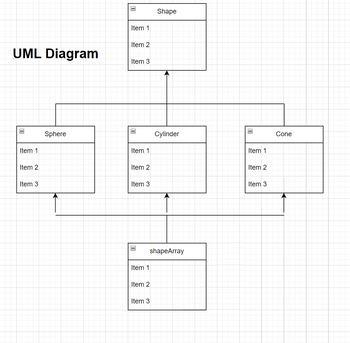
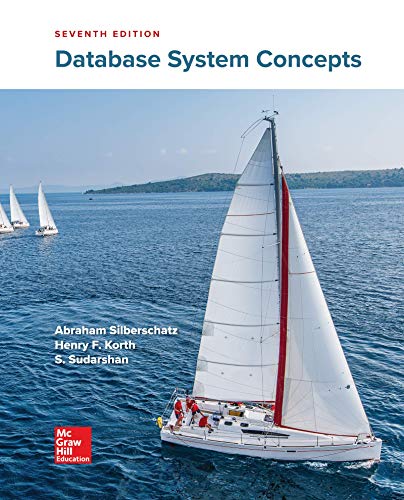
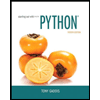
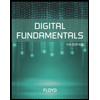
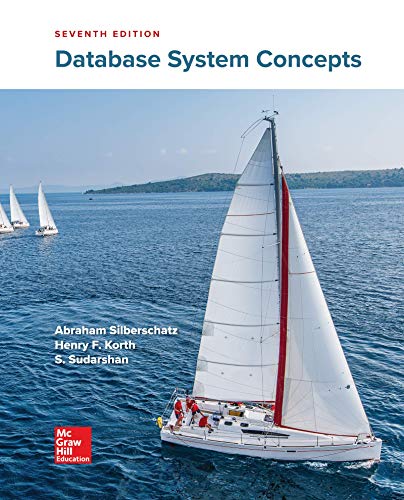
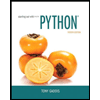
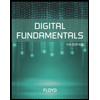
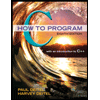
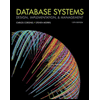
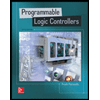