For the Circle class, create the following: • A private double instance variable m_radius. • A constructor that takes two parameters (in the order of color, radius). It should use the parameter for radius to set the m_radius instance variable and then explicitly call the parent class constructor using the super keyword and pass in the color parameter. • A method that overrides the calcArea method of the superclass and calculates the area of the circle. The area of a circle can be calculated as 3.14 xr² (where r is the radius). • A method that overrides the calcPerimeter method of the superclass and calculates the perimeter of the circle. The perimeter of a circle can be calculated as 2 x 3.14 xr (where r is the radius). For the Rectangle class, create the following: • Two private double instance variables m_width and m_length. • A constructor that takes three parameters (in the order of color, width, length). It should use the parameters for width and length to set the instance variables and then explicitly call the parent class constructor using the super keyword and pass in the color parameter. • A method that overrides the calcArea method of the superclass and calculates the area of the rectangle. The area of a rectangle can be calculated as width x height.
For the Circle class, create the following: • A private double instance variable m_radius. • A constructor that takes two parameters (in the order of color, radius). It should use the parameter for radius to set the m_radius instance variable and then explicitly call the parent class constructor using the super keyword and pass in the color parameter. • A method that overrides the calcArea method of the superclass and calculates the area of the circle. The area of a circle can be calculated as 3.14 xr² (where r is the radius). • A method that overrides the calcPerimeter method of the superclass and calculates the perimeter of the circle. The perimeter of a circle can be calculated as 2 x 3.14 xr (where r is the radius). For the Rectangle class, create the following: • Two private double instance variables m_width and m_length. • A constructor that takes three parameters (in the order of color, width, length). It should use the parameters for width and length to set the instance variables and then explicitly call the parent class constructor using the super keyword and pass in the color parameter. • A method that overrides the calcArea method of the superclass and calculates the area of the rectangle. The area of a rectangle can be calculated as width x height.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:P1.
In this question, you are provided a completed superclass – Shape (Do not make
any changes to this class!). This class represents generic shapes and has several methods
that you will have to override in the Circle and Rectangle subclasses, whose code skeletons
are provided as well. You are required to complete these two classes so that they can correctly
describe and calculate their attributes.
For the Circle class, create the following:
A private double instance variable m radius.
A constructor that takes two parameters (in the order of color, radius). It should use the
parameter for radius to set the m_radius instance variable and then explicitly call the
parent class constructor using the super keyword and pass in the color parameter.
A method that overrides the calcArea method of the superclass and calculates the area
of the circle. The area of a circle can be calculated as 3.14 x r² (where r is the radius).
A method that overrides the calcPerimeter method of the superclass and calculates the
perimeter of the circle. The perimeter of a circle can be calculated as 2 x 3.14 xr (wherer
is the radius).
For the Rectangle class, create the following:
Two private double instance variables m width and m_length.
A constructor that takes three parameters (in the order of color, width, length). It should use
the parameters for width and length to set the instance variables and then explicitly call the
parent class constructor using the super keyword and pass in the color parameter.
A method that overrides the calcArea method of the superclass and calculates the area
of the rectangle. The area of a rectangle can be calculated as width x height.
A method that overrides the calcPerimeter method of the superclass and calculates the
perimeter of the rectangle. The perimeter of a rectangle can be calculated as 2 × (width +
height).

Transcribed Image Text:P2.
In this question, you are provided with a Date class and an incomplete
EnhancedDate class. The EnhancedDate class inherits from the Date class with two more
methods:
addDays (int numDays)
This method adds a certain number of days to the date
represented by the current Date object. The changes should be left on the current object.
This task is done by first creating an object of the EnhancedDate class in the test driver with
an initial date and then calling the addDays method on this object. For example, if the current
EnhancedDate object, say currentDate, contains the date 1/1/2016, then after the
addDays (20) method is called on currentDate, the date in it would be 1/21/2016 because
20 days has been added to the original date. This is not a simple addition because you need to
take care of different months as well as leap years.
This method computes the number of days elapsed from
daysFrom (Date startDate)
startDate, and returns an integer. If the startDate is earlier than the current day, the return
should be positive, whereas if it is later than the current date, then this method should return a
negative number. Hint: you can call the compareTo method provided in the Date class to
check which of the two dates is older so that you can always count the days from the older date
to the newer date. You may choose to use the above addDays method to simplify this
implementation
date.
- you can keep adding a day to the older date until you bring it up to the newer
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 15 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
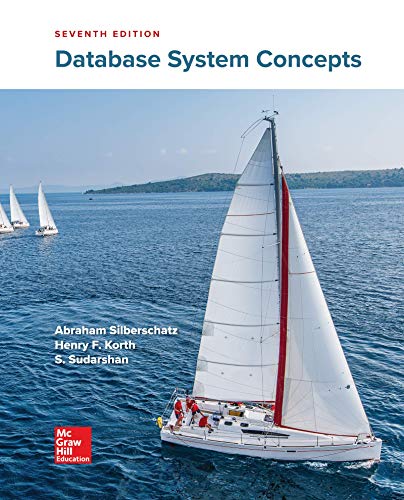
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
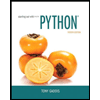
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
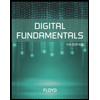
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
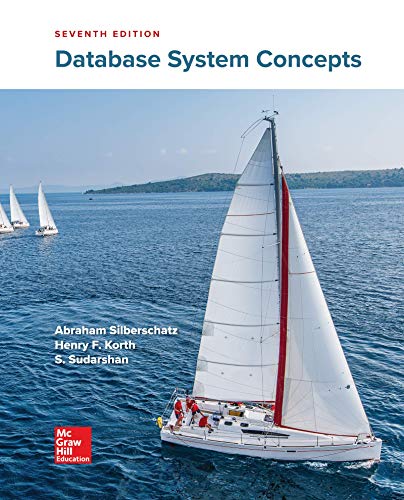
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
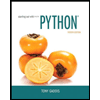
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
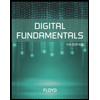
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
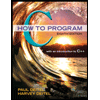
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
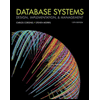
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
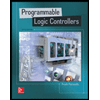
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education