For simple usage of GUIs, why would the source codes (shown below) not work? The input/output objects have not been properly handled. The data type of the methods has been defined in a wrong way. The necessary parameters in the methods have not been assigned properly. The flow of execution has been applied in a wrong way. import javax.swing.JOptionPane; public class Palindrome { public static void main(String[] args) //main method { long num1, num2; //variables to store the numbers String inputStr, outputStr; // strings to receive from the user // and display inputStr=JOptionPane.showInputDialog("Enter the number: "); num1 = Long.parseLong(inputStr); // change the string to num2 = num1; // numbers if (num1 <= 0) { num1 = - num1; //if the numbers are negative, change the sign inputStr = inputStr.valueOf(num1);//change the inputStr value } if (isPalindrome(inputStr)) outputStr = num2 + " is a palindrome"; else outputStr = num2 + " is not a palindrome"; JOptionPane.showMessageDialog(null, outputStr,"Palindrome Program",JOptionPane.INFORMATION_MESSAGE); System.exit(0); } public static void isPalindrome(String str) //call this method { int len = str.length(); int i, j; j = len - 1; for (i = 0; i <= (len - 1) / 2; i++) { if (str.charAt(i) != str.charAt(j)) return 0; j--; } return 1; } } Which of following is not true about using Java applets? A Java applet is a program that is intended to be transported over the Web and executed using a web browser. While a Java application is a stand-alone program with a main method, an Java applet doesn't have a main method. The paint method is manually executed regardless of the Java applet’s contents. The paint method also accepts a parameter that is an object of the Graphics class. To draw an oval object like this (shown below), how should we specify the method parameters (like coordinates and sizes)? 11 drawOval (50, 80, 175, 20); drawOval (20, 175, 80, 50); drawOval (175, 20, 50, 80); drawOval (175, 50, 80, 20); Which of the following is not true about GUI containers? A GUI container is a component that is used to hold and organize other components. A frame is a container displayed as a separate window with a title bar. A panel is a container that cannot be displayed on its own but is used to organize other components. A frame cannot be repositioned and resized on the screen as needed. Which of the following is not true about GUI events? An event in Java is an object that represents some activity to which we may want to respond. Even a movement of a mouse can be considered as an event. Since Java does not strictly define classes that represent typical events, users should define their own events. We set up a listener object to respond to an event when it occurs.
- For simple usage of GUIs, why would the source codes (shown below) not work?
- The input/output objects have not been properly handled.
- The data type of the methods has been defined in a wrong way.
- The necessary parameters in the methods have not been assigned properly.
- The flow of execution has been applied in a wrong way.
import javax.swing.JOptionPane;
public class Palindrome
{
public static void main(String[] args) //main method
{
long num1, num2; //variables to store the numbers
String inputStr, outputStr; // strings to receive from the user
// and display
inputStr=JOptionPane.showInputDialog("Enter the number: ");
num1 = Long.parseLong(inputStr); // change the string to
num2 = num1; // numbers
if (num1 <= 0)
{
num1 = - num1; //if the numbers are negative, change the sign
inputStr = inputStr.valueOf(num1);//change the inputStr value
}
if (isPalindrome(inputStr))
outputStr = num2 + " is a palindrome";
else
outputStr = num2 + " is not a palindrome";
JOptionPane.showMessageDialog(null, outputStr,"Palindrome Program",JOptionPane.INFORMATION_MESSAGE);
System.exit(0);
}
public static void isPalindrome(String str) //call this method
{
int len = str.length();
int i, j;
j = len - 1;
for (i = 0; i <= (len - 1) / 2; i++)
{
if (str.charAt(i) != str.charAt(j))
return 0;
j--;
}
return 1;
}
}
- Which of following is not true about using Java applets?
- A Java applet is a program that is intended to be transported over the Web and executed using a web browser.
- While a Java application is a stand-alone program with a main method, an Java applet doesn't have a main method.
- The paint method is manually executed regardless of the Java applet’s contents.
- The paint method also accepts a parameter that is an object of the Graphics class.
- To draw an oval object like this (shown below), how should we specify the method parameters (like coordinates and sizes)?
11
- drawOval (50, 80, 175, 20);
- drawOval (20, 175, 80, 50);
- drawOval (175, 20, 50, 80);
- drawOval (175, 50, 80, 20);
- Which of the following is not true about GUI containers?
- A GUI container is a component that is used to hold and organize other components.
- A frame is a container displayed as a separate window with a title bar.
- A panel is a container that cannot be displayed on its own but is used to organize other components.
- A frame cannot be repositioned and resized on the screen as needed.
- Which of the following is not true about GUI events?
- An event in Java is an object that represents some activity to which we may want to respond.
- Even a movement of a mouse can be considered as an event.
- Since Java does not strictly define classes that represent typical events, users should define their own events.
- We set up a listener object to respond to an event when it occurs.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

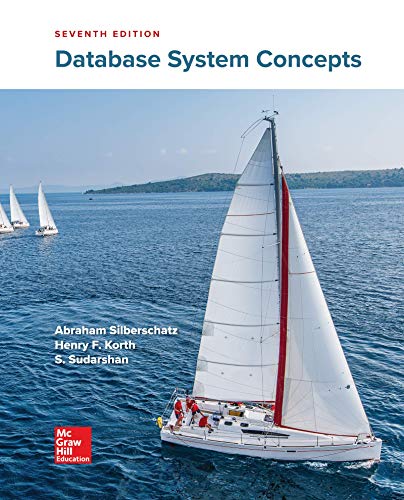
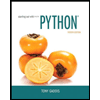
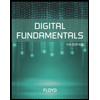
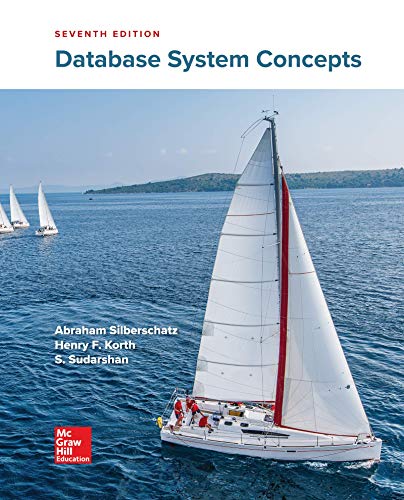
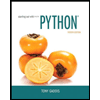
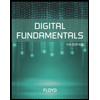
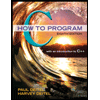
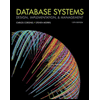
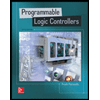