Fix the error Code: import numpy as np from statistics import mean import matplotlib as plt import matplotlib.pyplot as plt from random import randrange def takeHalfTest(a,b,oper,order): asOrig=np.random.randint(1,7,a) if(order==1): as1=np.sort(asOrig) else: as1=-np.sort(-asOrig) as1=as1[0:b] out=0 if(oper==1): out=min(as1) elif(oper==2): out=max(as1) elif(oper==3): out=mean(as1) else: diffs=np.diff(as1) dMin=np.min(diffs) j=np.argmin(diffs) if(dMin<0): out=j-1 else: out=b return out results = [] n= 10 m= 300 for a1 in range(1,n-1): for b1 in range(1,a1): for oper1 in range(1,4): for order1 in range(1,2): for a2 in range(1,(n-a1)): for b2 in range(1,a2): for oper2 in range(1,4): for order2 in range(1,2): counter1=0 counter2=0 for i in range(1,300): res1= takeHalfTest(a1,b1, oper1,order1) res2= takeHalfTest(a2,b2, oper2,order2) if res1 < res2: counter1= counter1+1 if res1 == res2 : counter2= counter2+1 # results += [a1,b1,oper1,order1, a2,b2,oper2,order2,1, counter1/m] # results += [a1,b1,oper1,order1, a2,b2,oper2,order2,2, counter2/m] results += [counter1/m] results += [counter2/m] print(results) ps = results f,p = plt.hist(ps,100) f = f / np.trapz(p,f) plt.plot(p,f) grid('on') plt.xlabel('P(procedure)') plt.ylabel('freq.') plt.title('Probabilities of diff. procedures') The Output of this code is: This is error message while visualizing the data
Fix the error
Code:
import numpy as np
from statistics import mean
import matplotlib as plt
import matplotlib.pyplot as plt
from random import randrange
def takeHalfTest(a,b,oper,order):
asOrig=np.random.randint(1,7,a)
if(order==1):
as1=np.sort(asOrig)
else:
as1=-np.sort(-asOrig)
as1=as1[0:b]
out=0
if(oper==1):
out=min(as1)
elif(oper==2):
out=max(as1)
elif(oper==3):
out=mean(as1)
else:
diffs=np.diff(as1)
dMin=np.min(diffs)
j=np.argmin(diffs)
if(dMin<0):
out=j-1
else:
out=b
return out
results = []
n= 10
m= 300
for a1 in range(1,n-1):
for b1 in range(1,a1):
for oper1 in range(1,4):
for order1 in range(1,2):
for a2 in range(1,(n-a1)):
for b2 in range(1,a2):
for oper2 in range(1,4):
for order2 in range(1,2):
counter1=0
counter2=0
for i in range(1,300):
res1= takeHalfTest(a1,b1, oper1,order1)
res2= takeHalfTest(a2,b2, oper2,order2)
if res1 < res2:
counter1= counter1+1
if res1 == res2 :
counter2= counter2+1
# results += [a1,b1,oper1,order1, a2,b2,oper2,order2,1, counter1/m]
# results += [a1,b1,oper1,order1, a2,b2,oper2,order2,2, counter2/m]
results += [counter1/m]
results += [counter2/m]
print(results)
ps = results
f,p = plt.hist(ps,100)
f = f / np.trapz(p,f)
plt.plot(p,f)
grid('on')
plt.xlabel('P(procedure)')
plt.ylabel('freq.')
plt.title('Probabilities of diff. procedures')
The Output of this code is: This is error message while visualizing the data


Step by step
Solved in 3 steps with 1 images

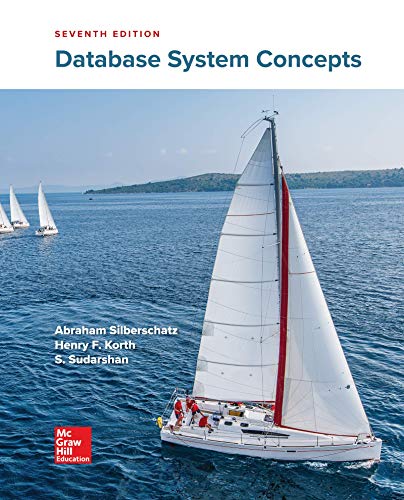
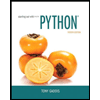
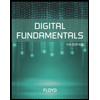
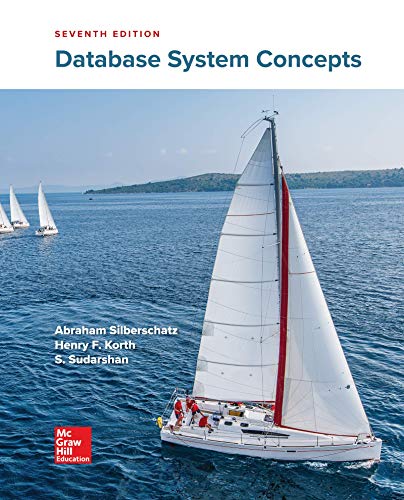
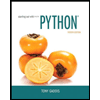
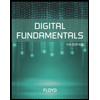
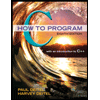
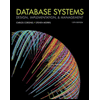
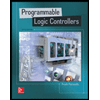