find the error in this code to display the required output from collections import deque def shortest_path(graph, start, goal): # keep track of explored nodes explored = [] # keep track of all the paths to be checked queue = deque([[start]]) if start == goal: print("Start and goal are the same") return # returns path if found while queue: # gets the first path from the queue path = queue.popleft() # gets the last node from the path node = path[-1] if node not in explored: neighbours = graph[node] # goes through all neighbour nodes, constructing a new path and # pushing it into the queue for neighbour in neighbours: new_path = list(path) new_path.append(neighbour) queue.append(new_path) # returns path if neighbour is goal if neighbour == goal: print("Shortest path found: ", *new_path) return new_path # marks node as explored explored.append(node) # returns None if goal not reached print("So sorry, but a connecting path does not exist :(") return # define the graph graph = { 1: [2,3], 2: [1,3], 3: [1,2,5, 4], 4: [6,11,3,5], 5: [7,4,3], 6: [8,4], 7: [5,9], 8: [6], 9: [7,10], 10: [9,12], 11: [12,4], 12: [10,11] } # modify the dfs function to return the charge when it reaches the charging station def dfs(graph, start, visited, charge): # current node as visited visited.add(start) # Check the Roomba needs to enter the charging station if charge <= 6: print(f" {start} (Charging, {charge} Wh) -> ") charge = 12 return charge else: print(f"{start} (Cleaning, {charge} Wh) ->") charge -= 1 # Take the list of adjacent nodes adj_nodes = graph[start] # Visit the unvisited adjacent nodes for node in adj_nodes: if node not in visited: charge = dfs(graph, node, visited, charge) return charge # ask the user to enter the starting node start_node = int(input("Enter starting node: ")) # perform depth-first search starting from the starting node visited = set() charge = dfs(graph, start_node, visited, 12) # keep looping until the Roomba has enough charge to reach the charging station while charge <= 6: # find the shortest path from the current node to the charging station shortest_path(graph, start_node, 12) # update the start node to be the current node start_node = shortest # Sample output Enter starting node: 1 1 (Cleaning, 12 Wh) -> 2 (Cleaning, 11 Wh) -> 3 (Cleaning, 10 Wh) -> 4 (Cleaning, 9 Wh)-> 6 (Cleaning, 8 Wh)-> 8 (Cleaning, 7 Wh)-> 6 (Charging, 6 Wh)-> 4 (Charging, 5 Wh)-> 11 (Charging, 4 Wh)-> 12 (Cleaning, 12 Wh)-> 10 (Cleaning, 11 Wh)-> 9 (Cleaning, 10 Wh)-> 7 (Cleaning, 9 Wh)-> 5 (Cleaning, 8 Wh)
find the error in this code to display the required output from collections import deque def shortest_path(graph, start, goal): # keep track of explored nodes explored = [] # keep track of all the paths to be checked queue = deque([[start]]) if start == goal: print("Start and goal are the same") return # returns path if found while queue: # gets the first path from the queue path = queue.popleft() # gets the last node from the path node = path[-1] if node not in explored: neighbours = graph[node] # goes through all neighbour nodes, constructing a new path and # pushing it into the queue for neighbour in neighbours: new_path = list(path) new_path.append(neighbour) queue.append(new_path) # returns path if neighbour is goal if neighbour == goal: print("Shortest path found: ", *new_path) return new_path # marks node as explored explored.append(node) # returns None if goal not reached print("So sorry, but a connecting path does not exist :(") return # define the graph graph = { 1: [2,3], 2: [1,3], 3: [1,2,5, 4], 4: [6,11,3,5], 5: [7,4,3], 6: [8,4], 7: [5,9], 8: [6], 9: [7,10], 10: [9,12], 11: [12,4], 12: [10,11] } # modify the dfs function to return the charge when it reaches the charging station def dfs(graph, start, visited, charge): # current node as visited visited.add(start) # Check the Roomba needs to enter the charging station if charge <= 6: print(f" {start} (Charging, {charge} Wh) -> ") charge = 12 return charge else: print(f"{start} (Cleaning, {charge} Wh) ->") charge -= 1 # Take the list of adjacent nodes adj_nodes = graph[start] # Visit the unvisited adjacent nodes for node in adj_nodes: if node not in visited: charge = dfs(graph, node, visited, charge) return charge # ask the user to enter the starting node start_node = int(input("Enter starting node: ")) # perform depth-first search starting from the starting node visited = set() charge = dfs(graph, start_node, visited, 12) # keep looping until the Roomba has enough charge to reach the charging station while charge <= 6: # find the shortest path from the current node to the charging station shortest_path(graph, start_node, 12) # update the start node to be the current node start_node = shortest # Sample output Enter starting node: 1 1 (Cleaning, 12 Wh) -> 2 (Cleaning, 11 Wh) -> 3 (Cleaning, 10 Wh) -> 4 (Cleaning, 9 Wh)-> 6 (Cleaning, 8 Wh)-> 8 (Cleaning, 7 Wh)-> 6 (Charging, 6 Wh)-> 4 (Charging, 5 Wh)-> 11 (Charging, 4 Wh)-> 12 (Cleaning, 12 Wh)-> 10 (Cleaning, 11 Wh)-> 9 (Cleaning, 10 Wh)-> 7 (Cleaning, 9 Wh)-> 5 (Cleaning, 8 Wh)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
find the error in this code to display the required output
from collections import deque
def shortest_path(graph, start, goal):
# keep track of explored nodes
explored = []
# keep track of all the paths to be checked
queue = deque([[start]])
if start == goal:
print("Start and goal are the same")
return
# returns path if found
while queue:
# gets the first path from the queue
path = queue.popleft()
# gets the last node from the path
node = path[-1]
if node not in explored:
neighbours = graph[node]
# goes through all neighbour nodes, constructing a new path and
# pushing it into the queue
for neighbour in neighbours:
new_path = list(path)
new_path.append(neighbour)
queue.append(new_path)
# returns path if neighbour is goal
if neighbour == goal:
print("Shortest path found: ", *new_path)
return new_path
# marks node as explored
explored.append(node)
# returns None if goal not reached
print("So sorry, but a connecting path does not exist :(")
return
# define the graph
graph = {
1: [2,3],
2: [1,3],
3: [1,2,5, 4],
4: [6,11,3,5],
5: [7,4,3],
6: [8,4],
7: [5,9],
8: [6],
9: [7,10],
10: [9,12],
11: [12,4],
12: [10,11]
}
# modify the dfs function to return the charge when it reaches the charging station
def dfs(graph, start, visited, charge):
# current node as visited
visited.add(start)
# Check the Roomba needs to enter the charging station
if charge <= 6:
print(f" {start} (Charging, {charge} Wh) -> ")
charge = 12
return charge
else:
print(f"{start} (Cleaning, {charge} Wh) ->")
charge -= 1
# Take the list of adjacent nodes
adj_nodes = graph[start]
# Visit the unvisited adjacent nodes
for node in adj_nodes:
if node not in visited:
charge = dfs(graph, node, visited, charge)
return charge
# ask the user to enter the starting node
start_node = int(input("Enter starting node: "))
# perform depth-first search starting from the starting node
visited = set()
charge = dfs(graph, start_node, visited, 12)
# keep looping until the Roomba has enough charge to reach the charging station
while charge <= 6:
# find the shortest path from the current node to the charging station
shortest_path(graph, start_node, 12)
# update the start node to be the current node
start_node = shortest
# Sample output
Enter starting node: 1
1 (Cleaning, 12 Wh) ->
2 (Cleaning, 11 Wh) ->
3 (Cleaning, 10 Wh) ->
4 (Cleaning, 9 Wh)->
6 (Cleaning, 8 Wh)->
8 (Cleaning, 7 Wh)->
6 (Charging, 6 Wh)->
4 (Charging, 5 Wh)->
11 (Charging, 4 Wh)->
12 (Cleaning, 12 Wh)->
10 (Cleaning, 11 Wh)->
9 (Cleaning, 10 Wh)->
7 (Cleaning, 9 Wh)->
5 (Cleaning, 8 Wh)
Enter starting node: 1
1 (Cleaning, 12 Wh) ->
2 (Cleaning, 11 Wh) ->
3 (Cleaning, 10 Wh) ->
4 (Cleaning, 9 Wh)->
6 (Cleaning, 8 Wh)->
8 (Cleaning, 7 Wh)->
6 (Charging, 6 Wh)->
4 (Charging, 5 Wh)->
11 (Charging, 4 Wh)->
12 (Cleaning, 12 Wh)->
10 (Cleaning, 11 Wh)->
9 (Cleaning, 10 Wh)->
7 (Cleaning, 9 Wh)->
5 (Cleaning, 8 Wh)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
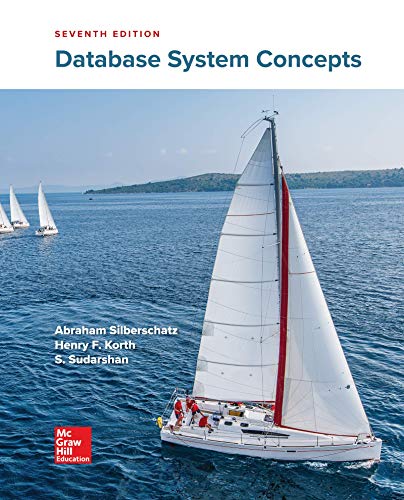
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
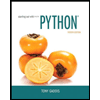
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
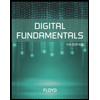
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
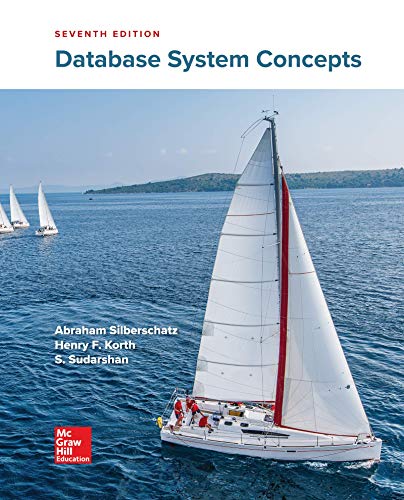
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
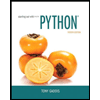
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
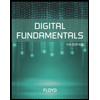
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
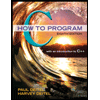
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
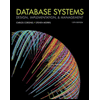
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
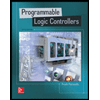
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education