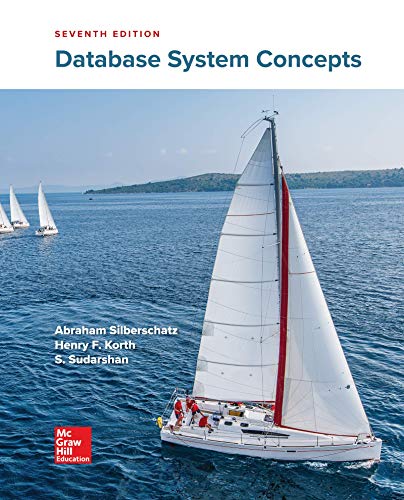
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![### Code Explanation for Educational Purposes
This C++ code snippet is designed to fill an array of strings with the letters of the alphabet from "a" to "z". Additionally, it includes a function to convert this array into a single string with each element delimited by commas and enclosed in square brackets.
#### Code Breakdown
1. **Header Files and Namespace**
```cpp
#include <iostream>
#include <string>
using namespace std;
```
- `#include <iostream>`: Includes the input/output stream standard library.
- `#include <string>`: Includes the string library for handling string operations.
- `using namespace std;`: Allows direct access to the standard namespace.
2. **Function Declaration**
```cpp
string to_string(string array[], int size);
```
- Declares the `to_string` function which converts the array to a formatted string.
3. **Main Function**
```cpp
int main()
{
const string alpha = "abcdefghijklmnopqrstuvwxyz";
const int SIZE = 26;
string letters[SIZE];
for (...) {
letters[...] = ...;
}
cout << to_string(letters, SIZE) << endl;
}
```
- Creates a constant string `alpha` representing the entire alphabet.
- Defines an integer `SIZE` with the value 26, the length of the alphabet.
- Initializes an array `letters` with 26 elements to store the alphabet.
- The for loop is intended to iterate over each letter of `alpha` and assign it to the `letters` array. The loop's body must be completed for functionality.
- Outputs the formatted string array using `cout`.
4. **String Conversion Function**
```cpp
string to_string(string a[], int size)
{
string result = "[";
if (size > 0)
{
result = result + "\"" + a[0] + "\"";
for (int i = 1; i < size; i++)
{
result = result + ", \"" + a[i] + "\"";
}
}
result = result + "]";
return result;
}
```
- Defines a function `to_string` that takes a string array and its size as parameters.
- Initializes `result` as `[` to prepare the opening bracket for the list.
- Checks if the array](https://content.bartleby.com/qna-images/question/c8525d30-f731-4576-a11c-639afeb912cb/61584cdc-887b-498e-9193-3e41f46bac77/tsdedil_thumbnail.jpeg)
Transcribed Image Text:### Code Explanation for Educational Purposes
This C++ code snippet is designed to fill an array of strings with the letters of the alphabet from "a" to "z". Additionally, it includes a function to convert this array into a single string with each element delimited by commas and enclosed in square brackets.
#### Code Breakdown
1. **Header Files and Namespace**
```cpp
#include <iostream>
#include <string>
using namespace std;
```
- `#include <iostream>`: Includes the input/output stream standard library.
- `#include <string>`: Includes the string library for handling string operations.
- `using namespace std;`: Allows direct access to the standard namespace.
2. **Function Declaration**
```cpp
string to_string(string array[], int size);
```
- Declares the `to_string` function which converts the array to a formatted string.
3. **Main Function**
```cpp
int main()
{
const string alpha = "abcdefghijklmnopqrstuvwxyz";
const int SIZE = 26;
string letters[SIZE];
for (...) {
letters[...] = ...;
}
cout << to_string(letters, SIZE) << endl;
}
```
- Creates a constant string `alpha` representing the entire alphabet.
- Defines an integer `SIZE` with the value 26, the length of the alphabet.
- Initializes an array `letters` with 26 elements to store the alphabet.
- The for loop is intended to iterate over each letter of `alpha` and assign it to the `letters` array. The loop's body must be completed for functionality.
- Outputs the formatted string array using `cout`.
4. **String Conversion Function**
```cpp
string to_string(string a[], int size)
{
string result = "[";
if (size > 0)
{
result = result + "\"" + a[0] + "\"";
for (int i = 1; i < size; i++)
{
result = result + ", \"" + a[i] + "\"";
}
}
result = result + "]";
return result;
}
```
- Defines a function `to_string` that takes a string array and its size as parameters.
- Initializes `result` as `[` to prepare the opening bracket for the list.
- Checks if the array
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
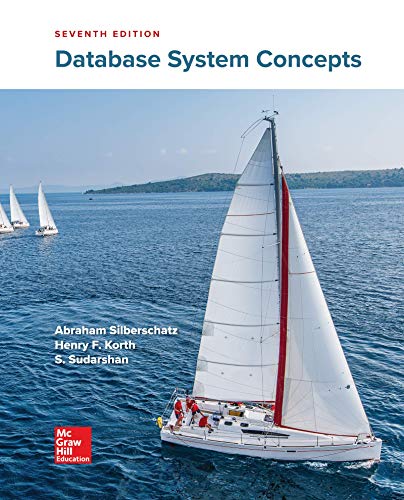
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
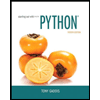
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
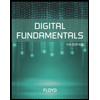
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
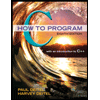
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
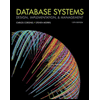
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
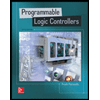
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education