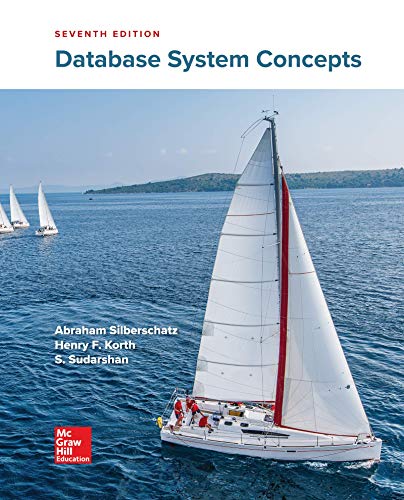
Concept explainers
Extend the
(b) #include<stdio.h>
#include<stdlib.h>
char c;
char name[10][50];
int roll[10];
float marks[10];
int main (int argc, char **argv)
{
FILE* f;
if (argc != 2)
{
printf("No filename in the argument");
return 1;
}
f = fopen(argv[1], "r");
if (f == NULL) {
printf("The file cannot be opened successfully: %s\n",argv[1]);
return 1;
}
printf("The file has been opened successfully\n\n");
int lines = 0;
while( c != EOF)
{
c = fgetc(f);
if(c == '\n')
{
lines++;
}
if(c ==EOF)
{
lines++;
}
else
{
printf("%c",c);
}
}
printf("\n\nFile has Total %d Lines.",lines);
//EXTENDED PART
f = fopen(argv[1], "r");
lines=0;
while( fscanf(f, "%s %d %f\n", name[lines], &roll[lines], &marks[lines]) != EOF )
{
lines++;
}
fclose(f);
char filename[] = "output.csv";
f=fopen(filename,"w");
int i=0;
while(i<lines)
{
fprintf(f,"%s,%d,%f\n",name[i],roll[i],marks[i]);
i++;
}
fclose(f);
return 0;
}

Step by stepSolved in 3 steps with 1 images

- Create an array called sales to monitor one week's worth of sales over the course of two months. Assign sales data to the array's elements. Then, in a loop, compute the average sales for the one week of each of the two months that are recorded in the array.arrow_forwardTicTacToeCreate the board display the board Set token to ‘x’Loop //at most 9 times, but end loop when someone wins{ Ask player where to place token display the board Checks if won Change token (if it was ‘x’ change to ‘o’ and vice versa)}Either congratulate someone on winning or say it was a draw. Create a 17x17 array of characters and put ‘ ‘ in each element.2. Write a loop to make the first horizontal line, and another to do the second horizontal line3. Write a loop to make the first vertical line, and another to create the second vertical line4. Put in the numbers (this is so the player can choose where to put the token). You will need 9 separate assignment statements.arrow_forwardThe program should allow the user to enter the age of the child and the number of days per week the child will be at the day care center. The program should output the appropriate weekly rate. The file provided for this lab contains all of the necessary variable declarations, except the two-dimensional array. You need to write the input statements and the code that initializes the two-dimensional array, determines the weekly rate, and prints the weekly rate. Comments in the code tell you where to write your statements. 1. Open the source code file named DayCare.cpp using Notepad or the text editor of your choice. 2. Declare and initialize the two-dimensional array. 3. Write the C++ statements that retrieve the age of the child and the number of days the child will be at the day care center. 4. Determine and print the weekly rate. 5. Save this source code file in a directory of your choice, and then make that directory your working directory. 6. Compile the source code…arrow_forward
- The subscript identifies the of a particular element in the array. O range value O location namearrow_forwardA four-row, three-column array has seven elements. Group of answer choices True Falsearrow_forwardThere are 2 arrays, the first contains item numbers in inventory and the second parallel array contains the price of the items. The user will enter the item number they want to purchase, the program checks to see if it is a valid entry. If it is valid, it will tell the user the price of the item. If it is an invalid item, a message will display telling the user they entered an invalid item. Type up the code below, execute the program a few times.arrow_forward
- Using C# in Microsoft Visual Studio create an application that grades the written portion of the driver’s license exam. The exam has 20 multiple-choice questions. (The correct answers are provided in the screenshot.) the program should store these correct answers in an array. The program should read the student’s answers for each of the 20 questions from a text file and store the answers in another array. (Create your own text file to test the application.) After the student’s answers have been read from the file, the program should display a message indicating whether the student passed or failed the exam. (A student must correctly answer 15 of the 20 questions to pass the exam.) It should then display the total number of correctly answered questions, the total number of incorrectly answered questions, and a list showing the question numbers of the incorrectly answered questions.arrow_forwardThe position number contained within square brackets is called the datatype of the array Select one: True Falsearrow_forwardBelow is an application in which the number of days for each month in the year is stored in an array. (Forexample, January has 31 days, February has 28, and so on. Assume that the year is not a leap year.) Display 12sentences in the same format for each month; for example, the sentence displayed for January is Month 1 has 31days. Given the following pseudocode, fill in the missing lines of code (see bold portions):class MonthDaysmain() //Declarations num month num SIZE = 12 num DAYS finish declaring arraymonth = 0while month < SIZE output complete output statement month = ____________endwhilereturnendClassarrow_forward
- A four-row, three-column array has seven elements. Group of answer choices True Falsearrow_forwardWhich of the following will repeat the loop instructions for each row in atwo-dimensional array named intNum?a. For intRow As Integer = 0 To intNum.GetUpperBound(0)b. For intRow As Integer = 0 To intNum.GetUpperBound(1)c. intRow = 0Do While intRow < intNum.GetUpperBound(0)d. both b and carrow_forwardThe intNums array is declared as follows: Dim intNums() As Integer = {10, 5, 7, 2}. Which of the following blocks of code correctly calculates the average value stored in the array? The intTotal, intSub, and dblAvg variables contain the number 0 before the loop is processed. a. Do While intSub < 4 intNums(intSub) = intTotal + intTotal intSub += 1 Loop dblAvg = intTotal / intSub b. Do While intSub < 4 intTotal += intNums(intSub) intSub = intSub + 1 Loop dblAvg = intTotal / intSub c. Do While intSub < 4 intTotal += intNums(intSub) intSub += 1 Loop dblAvg = intTotal / intSub – 1 d. Do While intSub < 4 intTotal = intTotal + intNums(intSub) intSub = intSub + 1 Loop dblAvg = intTotal / (intSub – 1)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
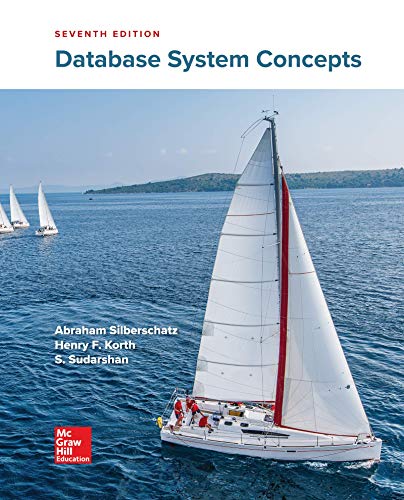
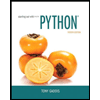
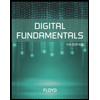
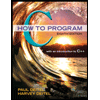
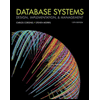
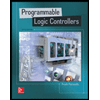