Explain each important segment of the code.
INSTRUCTIONS: Explain each important segment of the code.
import javax.swing.JOptionPane;
public class Quiz
{
/**
* isValid accapts a string and checks to see if it is A, B, or C
* and returns a boolean value of true or false if it matches
*/
private static boolean isValid(String a)
{
a = a.toLowerCase();
if(a.equals("a") || a.equals("b") || a.equals("c") || a.equals("d"))
{
return true;
}
else
{
JOptionPane.showMessageDialog(null, "Please pick A, B, or C");
return false;
}
}
/**
* askQuestion recieves an array containing the question and all the
* answers. Returning the user input as a String
*/
private static String askQuestion(String[] q)
{
String fmtQuestion = ""; // String to hold the formatted question
String answer = "";
for(int i = 0; i < q.length; i++)
fmtQuestion += q[i] + "\n";
do
{
answer = JOptionPane.showInputDialog(null, fmtQuestion);
if(answer == null)
{
int quit = JOptionPane.showConfirmDialog(null, "Would you like to quit?", "Quit", JOptionPane.YES_NO_OPTION);
if(quit == 0)
return "ABORT";
else
continue;
}
} while (answer == null || !(isValid(answer)));
return answer;
}
/**
* isCorrect recieves two values a (correct answer) and r (incorrect
* answer). Returning a boolean value if the answer is correct or not
*/
private static boolean isCorrect(String a, String r) // a = correct answer, r = user response
{
r = r.toUpperCase();
if(a.equals(r))
{
JOptionPane.showMessageDialog(null, "Correct!");
return true;
}
else
{
JOptionPane.showMessageDialog(null, "The correct answer is: \n" + a);
return false;
}
}
/**
* showGrade accepts two values c (total correct answers) and i (total
* incorrect answers). A message dialog is displayed stating the total
* coreect, incorrect, and the grade for the test
*/
public static void showGrade(int c, int i)
{
int numberQuestions = c + i;
String fmtGrade = "";
int pointsPerQuestion = 100 / numberQuestions;
int grade = c * pointsPerQuestion;
fmtGrade += "You answered " + c + " correctly and " + i + " incorrectly";
fmtGrade += "\nYour grade is: " + grade + "%";
JOptionPane.showMessageDialog(null, fmtGrade);
}
public static void main(String[] args)
{
int i = 0; // iterator to be used later for the askQuestion loop
int correct = 0; // number of correct answers
int incorrect = 0; // number of incorrect answers
String response = ""; // holds the answer the user supplied temporarily
/*
* I know multi-dimensional arrays are not till next chapter but
* I already knew about them and felt that it really was the best
* option for this assignment
*/
JOptionPane.showMessageDialog(null, "Are you a true Potterhead?");
String[][] question = new String[10][5]; // Array to store questions
String[] correctAnswer = new String[10]; // Array to store correct answers
question[0][0] = "Who is not in Gryffindor?";
question[0][1] = "A) Harry Potter";
question[0][2] = "B) Ron Weasley";
question[0][3] = "C) Draco Malfoy";
question[0][4] = "D) Ginny Weasley";
correctAnswer[0] = "C";
question[1][0] = "Which magical creatures did Gilderoy Lockhart set loose in class?";
question[1][1] = "A) Bowtruckles";
question[1][2] = "B) Cornish Pixies";
question[1][3] = "C) Occamy";
question[1][4] = "D) Kneazle";
correctAnswer[1] = "B";
question[2][0] = "What killed Moaning Myrtle?";
question[2][1] = "A) Nagini";
question[2][2] = "B) Basilisk";
question[2][3] = "C) Occamy";
question[2][4] = "D) Boa Constrictor";
correctAnswer[2] = "B";
question[3][0] = "What Harry did to get him temporarily expelled from Hogwarts?";
question[3][1] = "A) He inflates Aunt Marge";
question[3][2] = "B) He turns Vernon into a ferret";
question[3][3] = "C) He disapparates";
question[3][4] = "D) He chases away demetors";
correctAnswer[3] = "D";
question[4][0] = "What is Slytherin's house ghost called?";
question[4][1] = "A) The Bloody Baron";
question[4][2] = "B) The Fat Friar";
question[4][3] = "C) Nearly-Headless Nick";
question[4][4] = "D) The Grey Lady";
correctAnswer[4] = "A";
(continuation of the codes in the photo below)
(continuation of the codes in the photo below)
![question[50] = "What is the new password for entering into the Gryffindor common
room?";
question(5)1] = "A) Pig Snout":
question[52] = "B) Caput Draconis":
question[53) = "C) Wattlebird":
question(5)4) = "D) Abstinence":
carrectAnswer(5) = "C":
question[60] = "Who sent Harry his Firebolt?":
question[6(1] = "A) Professor McGonagall":
question[62) - "B) Sirius Black":
questione3) = "C) Remus Lupin";
question[614] = "D) Professor Dumbledare";
correciAnswerf6] = B:
question70] = Which of these is Harry's boggart?":
question(71] = "A) Giant Spider";
question(72) = "B) Professor Snape"
question[73] = "C) The moon";
question(614) - "D) A dementor":
carrectAnswer[7] = "D";
question[B0] = "Which department does Mr. Arthur Weasley work in?";
question[B1] = "A) The Misuse of Muggle Atifacts Office":
question[B2] = "B) Department of Magical Accidents and Catastrophes":
question[B3] = "C) Department of International Magical Cooperation";
question[814] = "D) Minister of Magic and Support Staf";
carrectAnswer[8] = "A";
question[90] = "Who does Hermione go with to Slughom'sChristmas party?":
question[91] = "A) Neville Longbottom";
question[92) = "B) Carmac Mcdaggen":
question[93] = "C) Viktor Krum":
question[94] = "D) Dean Thomas":
carrectAnswer(9) = "B":
N loop through the question array asking each one
op
response - askOuestion(question):
if(response.equals("ABORT")
return;
iflisCorrect(correctAnswerfj, response)
carrect += 1;
else
incorrect += 1;
} while(i < questionlength);
showGrade(correct, incorrect):](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F536c9f3c-7f76-4204-ae42-d14690a1c64b%2F45fdc51d-2b6c-4fcc-8aa2-9bcbf007937c%2Fovgqgqf_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

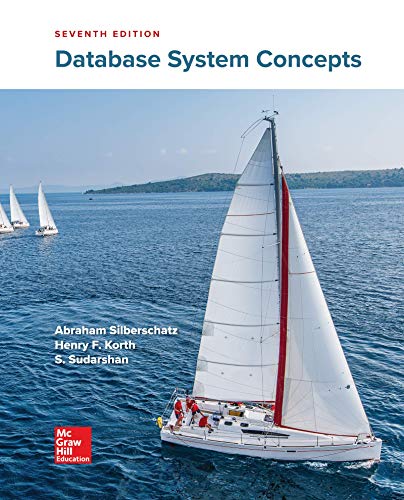
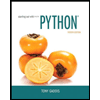
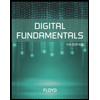
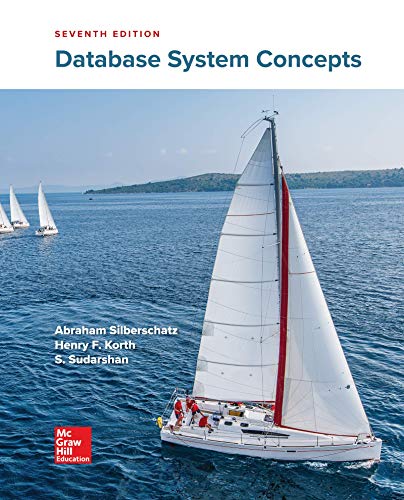
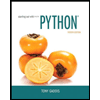
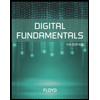
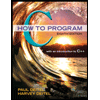
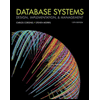
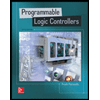