Exercise Arithmetic (Command-line arguments): Write a program called Arithmetic that takes three command-line arguments: two integers followed by an arithmetic operator (+, -, * or /). The program shall perform the corresponding operation on the two integers and print the result. For example: java Arithmetic 3 2 + 3+2=5 java Arithmetic 3 2 - 3-2=1 java Arithmetic 3 2 / 3/2=1 Hints: The method main(String[] args) takes an argument: "an array of String", which is often (but not necessary) named args. This parameter captures the command-line arguments supplied by the user when the program is invoked. For example, if a user invokes: java Arithmetic 12345 4567 + The three command-line arguments "12345", "4567" and "+" will be captured in a String array {"12345", "4567", "+"} and passed into the main() method as the argument args. That is, args is: {"12345", "4567", "+"} // args is a String array args.length is: 3 // length of the array args[0] is: "12345" // 1st element of the String array args[1] is: "4567" // 2nd element of the String array args[2] is: "+" // 3rd element of the String array args[0].length() is: 5 // length of 1st String element args[1].length() is: 4 // length of the 2nd String element args[2].length() is: 1 // length of the 3rd String element public class Arithmetic { public static void main (String[] args) { int operand1, operand2; char theOperator; // Check if there are 3 command-line arguments in the // String array args[] by using length variable of array. if (args.length != 3) { System.err.println("Usage: java Arithmetic int1 int2 op"); return; } // Convert the 3 Strings args[0], args[1], args[2] to int and char. // Use the Integer.parseInt(aStr) to convert a String to an int. operand1 = Integer.parseInt(args[0]); operand2 = ...... // Get the operator, assumed to be the first character of // the 3rd string. Use method charAt() of String. theOperator = args[2].charAt(0); System.out.print(args[0] + args[2] + args[1] + "="); switch(theOperator) { case ('-'): System.out.println(operand1 - operand2); break; case ('+'): ...... case ('*'): ...... case ('/'): ...... default: System.err.println("Error: invalid operator!"); } } } Notes: To provide command-line arguments, use the "cmd" or "terminal" to run your program in the form "java ClassName arg1 arg2 ....". To provide command-line arguments in Eclipse, right click the source code ⇒ "Run As" ⇒ "Run Configurations..." ⇒ Select "Main" and choose the proper main class ⇒ Select "Arguments" ⇒ Enter the command-line arguments, e.g., "3 2 +" in "Program Arguments". To provide command-line arguments in NetBeans, right click the "Project" name ⇒ "Set Configuration" ⇒ "Customize..." ⇒ Select categories "Run" ⇒ Enter the command-line arguments, e.g., "3 2 +" in the "Arguments" box (but make sure you select the proper Main class). Question: Try "java Arithmetic 2 4 *" (in CMD shell and Eclipse/NetBeans) and explain the result obtained. How to resolve this problem? In Windows' CMD shell, * is known as a wildcard character, that expands to give the list of file in the directory (called Shell Expansion). For example, "dir *.java" lists all the file with extension of ".java". You could double-quote the * to prevent shell expansion. Eclipse has a bug in handling this, even * is double-quoted. NetBeans?? Exercise SumDigits (Command-line arguments): Write a program called SumDigits to sum up the individual digits of a positive integer, given in the command line. The output shall look like: java SumDigits 12345 The sum of digits = 1 + 2 + 3 + 4 + 5 = 15
Exercise Arithmetic (Command-line arguments): Write a
java Arithmetic 3 2 +
3+2=5
java Arithmetic 3 2 -
3-2=1
java Arithmetic 3 2 /
3/2=1
Hints:
The method main(String[] args) takes an argument: "an array of String", which is often (but not necessary) named args. This parameter captures the command-line arguments supplied by the user when the program is invoked. For example, if a user invokes:
java Arithmetic 12345 4567 +
The three command-line arguments "12345", "4567" and "+" will be captured in a String array {"12345", "4567", "+"} and passed into the main() method as the argument args. That is,
args is: {"12345", "4567", "+"} // args is a String array
args.length is: 3 // length of the array
args[0] is: "12345" // 1st element of the String array
args[1] is: "4567" // 2nd element of the String array
args[2] is: "+" // 3rd element of the String array
args[0].length() is: 5 // length of 1st String element
args[1].length() is: 4 // length of the 2nd String element
args[2].length() is: 1 // length of the 3rd String element
public class Arithmetic {
public static void main (String[] args) {
int operand1, operand2;
char theOperator;
// Check if there are 3 command-line arguments in the
// String array args[] by using length variable of array.
if (args.length != 3) {
System.err.println("Usage: java Arithmetic int1 int2 op");
return;
}
// Convert the 3 Strings args[0], args[1], args[2] to int and char.
// Use the Integer.parseInt(aStr) to convert a String to an int.
operand1 = Integer.parseInt(args[0]);
operand2 = ......
// Get the operator, assumed to be the first character of
// the 3rd string. Use method charAt() of String.
theOperator = args[2].charAt(0);
System.out.print(args[0] + args[2] + args[1] + "=");
switch(theOperator) {
case ('-'): System.out.println(operand1 - operand2); break;
case ('+'): ......
case ('*'): ......
case ('/'): ......
default:
System.err.println("Error: invalid operator!");
}
}
}
Notes:
- To provide command-line arguments, use the "cmd" or "terminal" to run your program in the form "java ClassName arg1 arg2 ....".
- To provide command-line arguments in Eclipse, right click the source code ⇒ "Run As" ⇒ "Run Configurations..." ⇒ Select "Main" and choose the proper main class ⇒ Select "Arguments" ⇒ Enter the command-line arguments, e.g., "3 2 +" in "Program Arguments".
- To provide command-line arguments in NetBeans, right click the "Project" name ⇒ "Set Configuration" ⇒ "Customize..." ⇒ Select categories "Run" ⇒ Enter the command-line arguments, e.g., "3 2 +" in the "Arguments" box (but make sure you select the proper Main class).
Question: Try "java Arithmetic 2 4 *" (in CMD shell and Eclipse/NetBeans) and explain the result obtained. How to resolve this problem?
In Windows' CMD shell, * is known as a wildcard character, that expands to give the list of file in the directory (called Shell Expansion). For example, "dir *.java" lists all the file with extension of ".java". You could double-quote the * to prevent shell expansion. Eclipse has a bug in handling this, even * is double-quoted. NetBeans??
Exercise SumDigits (Command-line arguments): Write a program called SumDigits to sum up the individual digits of a positive integer, given in the command line. The output shall look like:
java SumDigits 12345
The sum of digits = 1 + 2 + 3 + 4 + 5 = 15

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 10 images

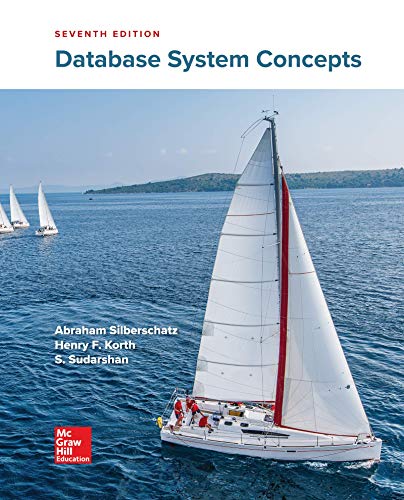
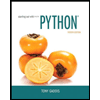
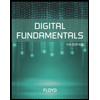
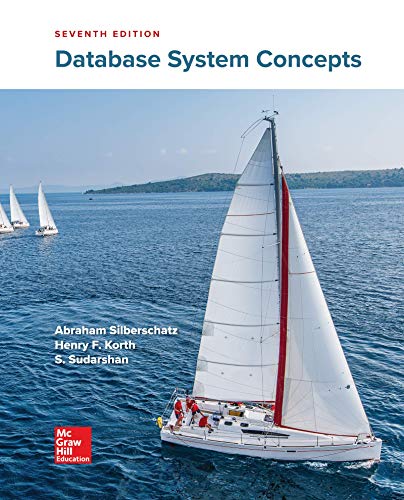
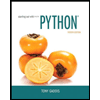
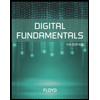
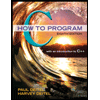
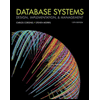
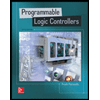