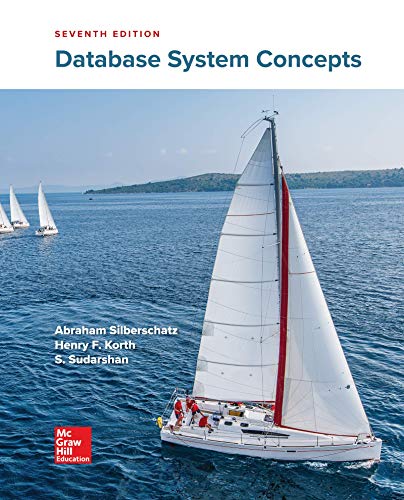
LAB 5.3 Working with the for Loop
Bring in
the Pre-lab Reading Assignment). This program has the user input a number n and
then finds the mean of the first n positive integers. The code is shown below:
// This program has the user input a number n and then finds the
// mean of the first n positive integers
// PLACE YOUR NAME HERE
#include <iostream>
using namespace std;
int main()
{
int value; // value is some positive number n
int total = 0; // total holds the sum of the first n positive numbers
int number; // the amount of numbers
float mean; // the average of the first n positive numbers
cout << "Please enter a positive integer" << endl;
cin >> value;
if (value > 0)
{
for (number = 1; number <= value; number++)
{
total = total + number;
} // curly braces are optional since there is only one statement
mean = static_cast<float>(total) / value; // note the use of the typecast
// operator here
cout << "The mean average of the first " << value
<< " positive integers is " << mean << endl;
continues
70 LESSON SET 5 Loops and Files
}
else
cout << "Invalid input - integer must be positive" << endl;
return 0;
}
Exercise 1: Why is the typecast operator needed to compute the mean in the
statement mean = static_cast(float)(total)/value;? What do you think
will happen if it is removed? Modify the code and try it. Record what happens.
Make sure that you try both even and odd cases. Now put static_cast<float>
total back in the program.
Exercise 2: What happens if you enter a float such as 2.99 instead of an integer
for value? Try it and record the results.
Exercise 3: Modify the code so that it computes the mean of the consecutive
positive integers n, n+1, n+2, . . . , m, where the user chooses n and m.
For example, if the user picks 3 and 9, then the program should find the
mean of 3, 4, 5, 6, 7, 8, and 9, which is 6.

Step by stepSolved in 5 steps with 8 images

- EXERCISE 19 Write a program using for loop that generates a multiplication table for any integer (given by the user) up to any multiple (also given by the user). Your output should be similar to what is given in the sample below. Sample Output >> exercise19 Enter the number for which you want to create the multiplication table: 91 Enter the number up to which multiplication table is required: 5 91 X 1- 91 91 X 2 = 182 91 X 3 - 273 91 X 4 - 364 91 X 5- 455 >> Electronic Engineering Technology---MATLAB Tutorialarrow_forwardA semicolon (;) is necessary at the end of which type of the loop? All loops do-while while forarrow_forwardProgram - Python Write a program asking the user to keep entering in strings (ie names) until they enter the string “leave”, at which point you’ll exit the loop and tell them how many times they entered the string “gold”. This one requires that you use a loop and also keep a tally of the number of times they enter the string gold.arrow_forward
- Write a FUNCTION that asks the user to input a string. Unless the user inputs "END" or "end", a loop will execute wherein the user is asked to input a string. Each time the loop runs and the user input a string, the function does the following: +Print the number of letters in the string that the user inputs; +Print the number of digits in the string that the user inputs. When the user enters "END" or "end" the loop stops and the function prints the aggregate numbers of letters and digits that the user has entered from the beginning. Example: 1st loop: a8K6H => 3 letters and 2 numbers 2nd loop: THGF*T425 => 5 letters and 3 numbers Aggragates: 8 letters and 5 numbersarrow_forwardin C#arrow_forwardIn Coral Language 3.22 LAB: Loops: Countdown until matching digits Write a program that takes in an integer in the range 20-98 as input. The output is a countdown starting from the integer, and stopping when both output digits are identical. Ex: If the input is: 93 the output is: 93 92 91 90 89 88 Ex: If the input is: 77 the output is: 77 Ex: If the input is: 9 or any number not between 20 and 98 (inclusive), the output is: Input must be 20-98 For coding simplicity, follow each output number by a space, even the last one. Use a while loop. Compare the digits; do not write a large if-else for all possible same-digit numbers (11, 22, 33, …, 88), as that approach would be cumbersome for large ranges.arrow_forward
- Can this be completed using a while loop at least once or twicearrow_forwardHow do I write the while loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop? Also including the calculations to compute the average rating.arrow_forwardWrite a complete program to generate a username based on a user's first and last name. Part One: Get a Valid First and Last Name ask the user for and read in their first and last name use a loop to validate the first name: it must be at least one character long and it must start with a letter use a loop to validate the last name: it must not contain any spaces Hint: use a nested loop. The outer loop will iterate until there is valid input. The inner loop will check each character in the String to see if any of them are whitespace. Part Two: Generate a Username generate a two-digit random number (i.e., a random number between 10 and 99, inclusive) create a username that is the following data concatenated: the first letter of their first name in lower case the first five characters of their last name in lower case; if the name is shorter than five characters, use the whole last name the two-digit number output the username to the user Additional Coding Requirements code…arrow_forward
- 12. Write a loop of the specified type that sums up the even integers between 1 and 21. a. a while loop b. a for looparrow_forwardIn this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. The source code file already contains the necessary assignment and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie.arrow_forwardBoolean Function - Pythona) Define a function, isInRange() with an integer parameter, x. This function returns true if the integer number x is in the range (-100, 100). Otherwise, return false.b) Define a main() function, in which a loop will be created to do the following ten times: - Get a random integer, n, in the range (-500, 500).- Call isInRange(x) function to determine and print out if the number n is in the range.c) Call main() function to initiate the tasks to be performed.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
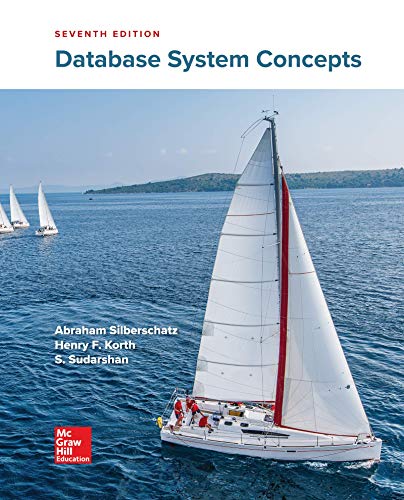
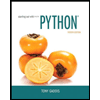
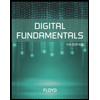
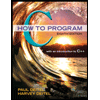
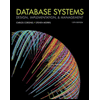
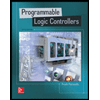