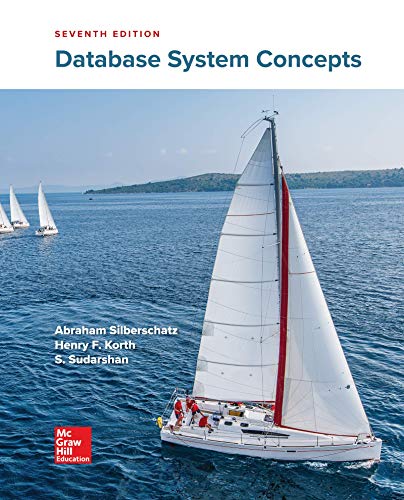
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Can anyone help me with these coding questions
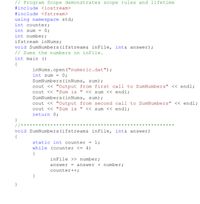
Transcribed Image Text:This C++ program demonstrates scope rules and lifetime through a simple function that processes data from a file. Below is a detailed breakdown of the code and its functionality:
### Program Description
The program reads numbers from a file named `numeric.dat` and calculates their sum. The process is repeated twice, with outputs displayed each time to illustrate the concept of variable scope and lifetime, specifically using a static variable within a function.
### Code
```cpp
// Program Scope demonstrates scope rules and lifetime
#include <iostream>
#include <fstream>
using namespace std;
int counter;
int sum = 0;
int number;
ifstream inNums;
void SumNumbers(ifstream& inFile, int& answer);
// Sums the numbers on inFile.
int main()
{
inNums.open("numeric.dat");
int sum = 0;
SumNumbers(inNums, sum);
cout << "Output from first call to SumNumbers" << endl;
cout << "Sum is " << sum << endl;
SumNumbers(inNums, sum);
cout << "Output from second call to SumNumbers" << endl;
cout << "Sum is " << sum << endl;
return 0;
}
//**********************************************
void SumNumbers(ifstream& inFile, int& answer)
{
static int counter = 1;
while (counter <= 4)
{
inFile >> number;
answer = answer + number;
counter++;
}
}
```
### Explanation
- **Global Variables**:
- `counter`, `sum`, `number`: Declared at the global scope, accessible throughout the program.
- **File Handling**:
- `ifstream inNums`: Used to handle operations related to the input file `numeric.dat`.
- **Function Declaration**:
- `void SumNumbers(ifstream& inFile, int& answer);`: Declares a function that processes numbers from a file and updates the sum.
- **Main Function**:
- Opens the file `numeric.dat`.
- Declares a local integer `sum`.
- Calls `SumNumbers` twice, demonstrating the effect of a static variable within the function.
- Outputs the calculated sum after each function call.
- **SumNumbers Function**:
- Contains a `static int counter = 1;` which retains its value between function calls.
- Reads numbers from the file and adds them to `answer` until `counter` exceeds 4.
###
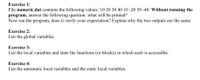
Transcribed Image Text:**Exercise 1:**
File *numeric.dat* contains the following values: 10 20 30 40 10 -20 30 -40. **Without running the program,** answer the following question: what will be printed?
Now run the program, does it verify your expectation? Explain why the two outputs are the same.
**Exercise 2:**
List the global variables.
**Exercise 3:**
List the local variables and state the functions (or blocks) in which each is accessible.
**Exercise 4:**
List the automatic local variables and the static local variables.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
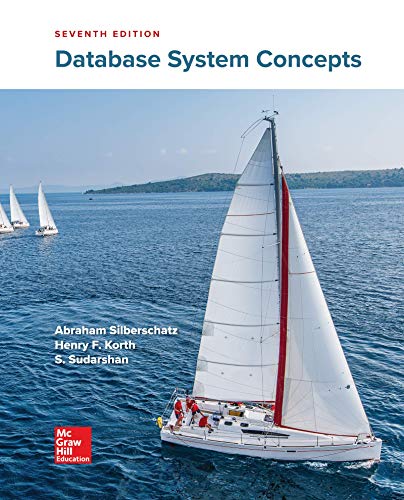
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
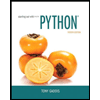
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
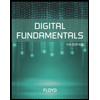
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
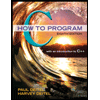
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
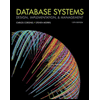
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
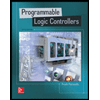
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education