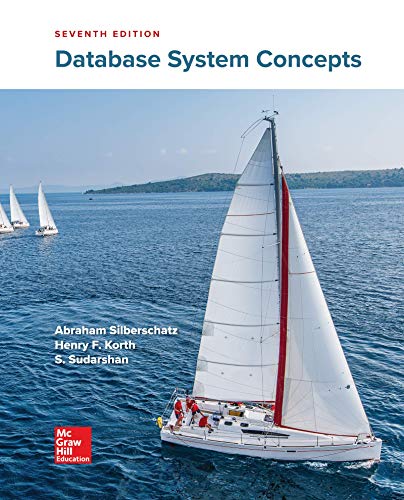
Concept explainers
Exception in thread "main" java.lang.NumberFormatException: For input string: "x" for Java code
public class Finder {
//Write two recursive functions, both of which will parse any length string that consists of digits and numbers. Both functions
//should be in the same class and have the following signatures.
//use the if/else statement , Find the base case and -1 till you get to base case
//recursive function that adds up the digits in the String
publicstaticint sumIt(String s)
{
//if String length is less or equal to 1 retrun 1.
if (s.length()<= 1){
return Integer.parseInt(s);
}else{
//use Integer.praseInt(s) to convert string to Integer
//returns the interger values
//else if the CharAt(value in index at 0 = 1) is not equal to the last vaule in the string else {//return the numeric values of a char value + call the SumIt method with a substring = 1
return Character.getNumericValue(s.charAt(0) ) + sumIt(s.substring(1));
}
}
//write a recursion function that will find the largest interger in the string.
publicstaticint findMax(String s,intmax)
{//check the characters of the string is greater than 0
if(s.length() == 0) {
returnmax;
}
if(s.substring(0, 1).matches("[0-9]")) {
intend = 1;
while(end < s.length() && s.substring(end, end+1).matches("[0-9]")) {
end++;
}//create varabile to convert to string with ( 0,end)
inta = Integer.parseInt(s.substring(0, end));
if(a > max) {// call findMax(use s.substring(end),a)
returnfindMax(s.substring(end), a);
}//call again
elsereturnfindMax(s.substring(end), max);
}//call again
elsereturnfindMax(s.substring(1), max);
}
//input string result
//"1d2d3d" 6
//"55" 10
//"xx" 0
//input string result
//"12x8" 12
//"012x88" 88
//"012x88ttttt9xe33ppp100" 100
//Testing
publicstaticvoid main(String[] args) {
String a = "12X8";
String b = "55";
String c = "xx";
String d = "12x8";
String e = "012x88";
String f = "012x88ttttt9xe33ppp100";
System.out.println(sumIt(a));
System.out.println (sumIt(b));
System.out.println (sumIt(c));
System.out.println (findMax(d, 0));
System.out.println (findMax(e,0));
System.out.println (findMax(f,0));
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

For the SumIt function - the print statement is suppose to print only the numeric total for the string
input string | result
//"1d2d3d" | 6
//"55" | 10
//"xx" |0
How do I fix the formatting ?
For the SumIt function - the print statement is suppose to print only the numeric total for the string
input string | result
//"1d2d3d" | 6
//"55" | 10
//"xx" |0
How do I fix the formatting ?
- The provided file has syntax and/or logical errors. Determine the problem and fix the program.arrow_forwardLab Goal : This lab was designed to teach you more about recursion. Lab Description : luckyThrees will return a count of the 3s in the number unless the 3 is at the start. A 3 at the start of the number does not count. /* luckyThrees will return the count of 3s in the number* unless the 3 is at the front and then it does not count* 3 would return 0* 31332 would return 2* 134523 would return 2* 3113 would return 1* 13331 would return 3* 777337777 would return 2* the solution to this problem must use recursion*/public static int luckyThrees( long number ){} Sample Data : 331332134523311313331777337777 Sample Output : 022132arrow_forward}/*** Adds letterGuessed to the current String updateLetterBoard* @param usedLetterBoard* @param letterGuessed* @param first* @return updateLetterBoard with letterGuess appended. */public static String updateLetterBoard(String usedLetterBoard, char letterGuessed, boolean first) {return "";}arrow_forward
- Simple try-catch Program This lab is a simple program that demonstrates how try-catch works. You will notice the output when you enter incorrect input (for example, enter a string or double instead of an integer). Type up the code, execute and submit the results ONLY. Do at least 2 valid inputs and 1 invalid. NOTE: The program stops executing after it encounters an error! CODE: import java.util.Scanner; public class TryCatchExampleSimple { public static void main(String[] args) { Scanner input = new Scanner(System.in); int num = 0; System.out.println("Even number tester.\n"); System.out.println("Enter your name: "); String name input.nextLine(); } } while (true) { try { System.out.println("Enter an integer : "); num= input.nextInt (); } int mod = num%2; if (mod == 0) " System.out.println(name + else " System.out.println(name + num = 0; } catch (Exception e) { break; > System.out.println("ERROR: The number you entered is illegal!"); System.out.println("Exception error: "+e.toString());…arrow_forwardC#Write a method MyMethod which takes an int parameter named x. This method calculates the sum of integers from 1 to x and returns the sum. Use a for loop.arrow_forwardWrite the code in java and understand what the question says and give me the code and don't copy or plagiarize pleasearrow_forward
- JAVA PROGRAM Homework #2. Chapter 7. PC #13. Name Search (page 492) Read these instructions for additional requirements carefully. Write a program that reads the contents of the two files into two separate arrays. The user should be able to enter a name the application will display messages indicating whether the names were among the most popular. 1. GirlNames.txt and BoyNames.txt contain a list of the 200 most popular names given to girls/boys born in the United States for the years 2000 through 2009. 2. Your application should use an array to hold the names. 3. The program should continue interacting with the user indefinitely unless the user chooses to quit by entering "QUIT" (should be case insensitive). 4. The user should enter a single name and the program will search the name in both lists. The user SHOULD NOT specify whether the search is for girls name or boys name. The program is responsible for finding either or both and telling the user where it found it. 5.…arrow_forwardJAVA PROGRAM Homework #2. Chapter 7. PC #13. Name Search (page 492) Read these instructions for additional requirements carefully. Write a program that reads the contents of the two files into two separate arrays. The user should be able to enter a name the application will display messages indicating whether the names were among the most popular. 1. GirlNames.txt and BoyNames.txt contain a list of the 200 most popular names given to girls/boys born in the United States for the years 2000 through 2009. 2. Your application should use an array to hold the names. 3. The program should continue interacting with the user indefinitely unless the user chooses to quit by entering "QUIT" (should be case insensitive). 4. The user should enter a single name and the program will search the name in both lists. The user SHOULD NOT specify whether the search is for girls name or boys name. The program is responsible for finding either or both and telling the user where it found it. 5.…arrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forward
- Using jGRASP please de-bug this /**This program demonstrates an array of String objects.It should print out the days and the number of hours spent at work each day.It should print out the day of the week with the most hours workedIt should print out the average number of hours worked each dayThis is what should display when the program runs as it shouldSunday has 12 hours worked.Monday has 9 hours worked.Tuesday has 8 hours worked.Wednesday has 13 hours worked.Thursday has 6 hours worked.Friday has 4 hours worked.Saturday has 0 hours worked.Highest Day is Wednesday with 13 hours workedAverage hours worked in the week is 7.43 hours*/{public class WorkDays{public static void main(String[] args){String[] days = { "Sunday", "Monday", "Tuesday","Wednesday", "Thursday", "Friday", "Saturday"}; int[] hours = { 12, 9, 8, 13, 6, 4}; int average = 0.0;int highest = 0;String highestDay = " ";int sum = 0; for (int index = 0; index < days.length; index++){System.out.println(days[index] + " has "…arrow_forwardIN C++ Write code that: creates 3 integer values - one can be set to 0, the other two should NOT multiples of one another (for example 3 and 6, or 2 and 8) take you largest value and perform a modulus operation using the smaller (non zero) value as the divisor print out the result. create an alias for the string type call the alias "name" then create an instance of the "name" class and assign it a value using an appropriate cout statement - print out the value Please screenshot your input and output, as the format tends to get messed up. Thank you!arrow_forwardusing System; class TicTacToe { Console.WriteLine("Do you want be X or O: "); Console.Write("Press 1 for 'X' or Press 2 for 'O'\n"); choice = int.Parse(Console.ReadLine()); if(choice==1) staticint player = 1 staticint choice; { staticvoid Print(char[] board) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } staticvoid Main(string[] args) { char[] board = newchar[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); } } } How can this program be fixed to ask the user "It is your turn to place an X. Where would you like to place it?"arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
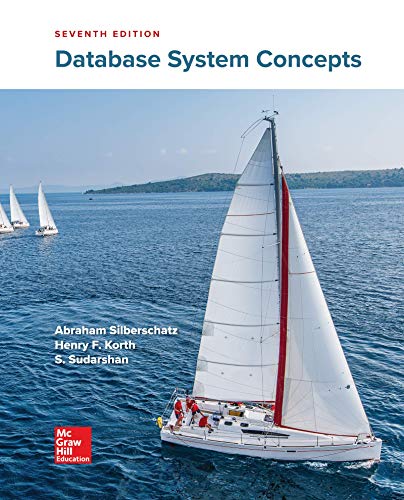
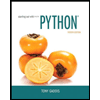
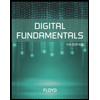
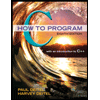
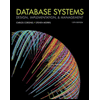
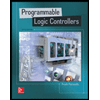