elf) method that will return a tuple or list with self.lat, self.lon. A CalcDistance(self, lat, lon) method that will figure out the distance between the object’s self.lat, self.lon and lat, lon parameters passed in. A CalcDistancePoint(self, point) method that takes in a point (both coordinates at once, a data sequence, or GeoPoint object) that will figure out the distance between the object’s self.lat, self.lon and the lat and lon from the point. A SetDescription(self, description) method that will set the objects self.description attribute (variable). A GetDescription(self) method that will return the objects self.description attribute. Add a property: Point = property(GetPoint,SetPoint). Make sure GetPoint and SetPoint are the names you used for the get and set methods you already wrote for
elf) method that will return a tuple or list with self.lat, self.lon. A CalcDistance(self, lat, lon) method that will figure out the distance between the object’s self.lat, self.lon and lat, lon parameters passed in. A CalcDistancePoint(self, point) method that takes in a point (both coordinates at once, a data sequence, or GeoPoint object) that will figure out the distance between the object’s self.lat, self.lon and the lat and lon from the point. A SetDescription(self, description) method that will set the objects self.description attribute (variable). A GetDescription(self) method that will return the objects self.description attribute. Add a property: Point = property(GetPoint,SetPoint). Make sure GetPoint and SetPoint are the names you used for the get and set methods you already wrote for
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter8: Arrays
Section: Chapter Questions
Problem 11E
Related questions
Question
I am creating a class using python, I am stuck with inserting the functions as I have run across some errors. I will attach my program and the instructions.
- In your program define a class called GeoPoint that will have the following:
- A constructor __init__(self, lat=0, lon=0,description=’TBD’) that will initialize the class variables called: self.lat, self.lon, and self.description.
- A SetPoint(self, point) method that takes an individual points coordinates as a single sequence and sets them to self.lat, self.long. E.g:
self.lat = point[0]
self.lon =point[1]
- A GetPoint(self) method that will return a tuple or list with self.lat, self.lon.
- A CalcDistance(self, lat, lon) method that will figure out the distance between the object’s self.lat, self.lon and lat, lon parameters passed in.
- A CalcDistancePoint(self, point) method that takes in a point (both coordinates at once, a data sequence, or GeoPoint object) that will figure out the distance between the object’s self.lat, self.lon and the lat and lon from the point.
- A SetDescription(self, description) method that will set the objects self.description attribute (variable).
- A GetDescription(self) method that will return the objects self.description attribute.
- Add a property: Point = property(GetPoint,SetPoint). Make sure GetPoint and SetPoint are the names you used for the get and set methods you already wrote for points.
- Add another property: Description = property(GetDescription, SetDescription). Make sure GetDescription and SetDescription are the names you used for the get and set methods you already wrote.
- In the main part of your program do the following:
- Include the class.
- Instantiate three points.
- Use the constructor to set point1 coordinates and description. It should look something like the following but with your own coordinates and description:
point1 = GeoPoint(12.3456,-123.4567,'Loc1')
- Use a constructor without any arguments to instantiate the second point and use its properties to set its values. It should look something like the following but with your own coordinates and description:
point2 = GeoPoint()
point2.Point = 23.4567, -213.456
point2.Description = 'Loc2'
- For point3 use the SetPoint and SetDescription methods to set the points location and description. Make sure they have different coordinates and different descriptions. It should look something like the following but with your own coordinates and description:
point3 = GeoPoint()
point3.SetPoint((25.25,52.52))
point3.SetDescription(“Loc3”)
- Inside a “Do another (y/n)?” loop do the following:
- Create a fourth point by asking the user for their location. You can ask for coordinates in three inputs or ask them for their coordinates in one input with each element separated by a coma.
- Use the CalcDistance method to find the distance between point1 and a new latitude and longitude of your choosing. Then tell the user the coordinates for point1, the new latitude and longitude that was entered, and the distance between those coordinates. For example:
distanceToPoint1 = point1.CalcDistance(lat, lon)
- Use point2 and point3’s CalcDistancePoint method to find the distance from each point to the user’s location. Notice we are passing in the users point rather than the separate coordinates:
distanceToTwo = point2.CalcDistancePoint(userPoint)
distanceToThree = point3.CalcDistancePoint(userPoint)
- Tell the user which point they are closest to in this format:
You are closest to <description> which is located at <point’s lat and lon coordinates>
- Ask “Do another (y/n)?” and loop if they respond with ‘y’
![IDLE File Edit Format Run Options
D2L Homepa
ue:
lon =
map(
and longit
culate the
values (la
anceToOne
ance ToTwo
listanceToo
print (f"Yo
locate
Beautify
GeoPoint (1
GeoPoint ()
zing value
([23
int-
lon)
scription
from math import cos, asin,sqrt, pi
class GeoPoint:
5
45
46 #Function helps to get the point
def GetPoint (self):
47
48
GeoPoint (1
GeoPoint() #Distance calculation method between two points
zing value
def Distance (self, toPoint):
int' =>
([23 49
s (lat, lo 50
escription 51
52
5
# Parametrizzed and default constructor
def _init__(self, lat=0, lon=0, description = 'TBD'):
Window Help
self.__lat= lat
self.__long = lon
self.__description = description
# Latitude and longitude setup programme
def SetPoint (self, coords):
self._
lat = coords [0]
self.__lon = coords [1]
YazzieTP6.py - /Users/tishenayazzie/Documents/YazzieTP6.py (3.7.9)
return (self.__lat,self.__lon)
p = pi/180
a = 0.5 cos((toPoint [0]-(self.Point) [0])*p)/2 + cos((self
.Point) [0]*p) * cos(toPoint [0]*p) * (1-cos((toPoint [1]
-(self.Point) [1]) *p))/2
return 12742 * asin(sqrt(a))
#Function defining to set the self description
def Set Description(self, description):
self.__description = description
return self.__description
#Function that returns the self description
def GetDescription(self, description):
5 #Property of Point to access and set point values
Point = property (GetPoint, SetPoint)
#To set and access the point's description, use their description attribute
Description = property (GetDescription, Set Description)
#Giving a value to the function Object (), i.e constructor to initialize the object
point1= GeoPoint (12.3456, -123, 'Chicago')
point2 = GeoPoint ()
#initializing values of longitude and lattitude
point2.Point = ([23.4567, -213.4567])
point2. Description = 'Loc2'
while True:
lat, lon = map (float, input ("Enter the location points of latitude and longitude: ").split(","))
#Calculate the distance between two places using a list of values (lat, lon)
distancetoone = point1.Distance ([lat, lon])
distancetotwo = point2.Distance ([lat, lon])
1800
blaaari
if distancetoone < distancetotwo:
else:
print (f"You are closest to {point1.GetDescription()} which is located at ", tuple (point1.Point))
print (f"You are closest to {point2.GetDescription()} which is located at tunle(noint2. Point))
Ni
NOV 1
2
tv
Your program will have the following functions
Ln: 28
A
Col: 22
borate
U
((.
80
DOCX
CL
Format
Et
Wed Nov 2 5:27 PM
Document
Nothing selected.
Select an object or text to format.
at Document
Update
More
10.5 pt
A
ΑΞ
1.2
0 pt
8 pt](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F75caf353-1d6b-4c64-a099-344a34c0daad%2Ff9d6e95c-e172-4e27-ab9e-a2c4d419c83d%2F48x9vqv_processed.png&w=3840&q=75)
Transcribed Image Text:IDLE File Edit Format Run Options
D2L Homepa
ue:
lon =
map(
and longit
culate the
values (la
anceToOne
ance ToTwo
listanceToo
print (f"Yo
locate
Beautify
GeoPoint (1
GeoPoint ()
zing value
([23
int-
lon)
scription
from math import cos, asin,sqrt, pi
class GeoPoint:
5
45
46 #Function helps to get the point
def GetPoint (self):
47
48
GeoPoint (1
GeoPoint() #Distance calculation method between two points
zing value
def Distance (self, toPoint):
int' =>
([23 49
s (lat, lo 50
escription 51
52
5
# Parametrizzed and default constructor
def _init__(self, lat=0, lon=0, description = 'TBD'):
Window Help
self.__lat= lat
self.__long = lon
self.__description = description
# Latitude and longitude setup programme
def SetPoint (self, coords):
self._
lat = coords [0]
self.__lon = coords [1]
YazzieTP6.py - /Users/tishenayazzie/Documents/YazzieTP6.py (3.7.9)
return (self.__lat,self.__lon)
p = pi/180
a = 0.5 cos((toPoint [0]-(self.Point) [0])*p)/2 + cos((self
.Point) [0]*p) * cos(toPoint [0]*p) * (1-cos((toPoint [1]
-(self.Point) [1]) *p))/2
return 12742 * asin(sqrt(a))
#Function defining to set the self description
def Set Description(self, description):
self.__description = description
return self.__description
#Function that returns the self description
def GetDescription(self, description):
5 #Property of Point to access and set point values
Point = property (GetPoint, SetPoint)
#To set and access the point's description, use their description attribute
Description = property (GetDescription, Set Description)
#Giving a value to the function Object (), i.e constructor to initialize the object
point1= GeoPoint (12.3456, -123, 'Chicago')
point2 = GeoPoint ()
#initializing values of longitude and lattitude
point2.Point = ([23.4567, -213.4567])
point2. Description = 'Loc2'
while True:
lat, lon = map (float, input ("Enter the location points of latitude and longitude: ").split(","))
#Calculate the distance between two places using a list of values (lat, lon)
distancetoone = point1.Distance ([lat, lon])
distancetotwo = point2.Distance ([lat, lon])
1800
blaaari
if distancetoone < distancetotwo:
else:
print (f"You are closest to {point1.GetDescription()} which is located at ", tuple (point1.Point))
print (f"You are closest to {point2.GetDescription()} which is located at tunle(noint2. Point))
Ni
NOV 1
2
tv
Your program will have the following functions
Ln: 28
A
Col: 22
borate
U
((.
80
DOCX
CL
Format
Et
Wed Nov 2 5:27 PM
Document
Nothing selected.
Select an object or text to format.
at Document
Update
More
10.5 pt
A
ΑΞ
1.2
0 pt
8 pt
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
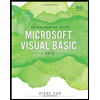
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
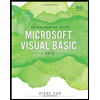
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning