e def scheduler_mark_completed(self, t): 1 ***Marks the task t as completed, and returns the additional 2 set of tasks that can be done (and that could not be 3 previously done) once t is completed.*** 4 # YOUR CODE HERE 5 6 DependencyScheduler.available_tasks = property (scheduler_available_tasks) 7 DependencyScheduler.mark_completed = scheduler_mark_completed 8 WN HO 00
e def scheduler_mark_completed(self, t): 1 ***Marks the task t as completed, and returns the additional 2 set of tasks that can be done (and that could not be 3 previously done) once t is completed.*** 4 # YOUR CODE HERE 5 6 DependencyScheduler.available_tasks = property (scheduler_available_tasks) 7 DependencyScheduler.mark_completed = scheduler_mark_completed 8 WN HO 00
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python help please.
![Question 1: implement available_tasks and mark_completed.
[ ]
1 ### Implementation of available_tasks and mark_completed".
2
3 def scheduler_available_tasks(self):
4
"""Returns the set of tasks that can be done in parallel.
5
6
A task can be done if all its predecessors have been completed.
And of course, we don't return any task that has already been
completed."*****
7
8
# YOUR CODE HERE
9
10 def scheduler_mark_completed(self, t):
11
"""Marks the task t as completed, and returns the additional
set of tasks that can be done (and that could not be
12
13
previously done) once is completed.""
14
# YOUR CODE HERE
15
16 DependencyScheduler.available_tasks = property (scheduler_available_tasks)
17 DependencyScheduler.mark_completed = scheduler_mark_completed
18
1 # Here is a place where you can test your code.
2
3 # YOUR CODE HERE
4
Let us check if this works.
[ ] 1 s = DependencyScheduler ()
2 s.add_task('a', ['b', 'c'])
3 s.add_task('b', ['c', 'e'])
4 s._check()
5 s.show()
6
[ ]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc06466e0-b6af-451e-ad3a-a7add978ace4%2F80f459fc-4a57-46ec-a340-ebf2f5e395de%2F8otzd6a_processed.png&w=3840&q=75)
Transcribed Image Text:Question 1: implement available_tasks and mark_completed.
[ ]
1 ### Implementation of available_tasks and mark_completed".
2
3 def scheduler_available_tasks(self):
4
"""Returns the set of tasks that can be done in parallel.
5
6
A task can be done if all its predecessors have been completed.
And of course, we don't return any task that has already been
completed."*****
7
8
# YOUR CODE HERE
9
10 def scheduler_mark_completed(self, t):
11
"""Marks the task t as completed, and returns the additional
set of tasks that can be done (and that could not be
12
13
previously done) once is completed.""
14
# YOUR CODE HERE
15
16 DependencyScheduler.available_tasks = property (scheduler_available_tasks)
17 DependencyScheduler.mark_completed = scheduler_mark_completed
18
1 # Here is a place where you can test your code.
2
3 # YOUR CODE HERE
4
Let us check if this works.
[ ] 1 s = DependencyScheduler ()
2 s.add_task('a', ['b', 'c'])
3 s.add_task('b', ['c', 'e'])
4 s._check()
5 s.show()
6
[ ]
![4
5 class
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
DependencyScheduler(object):
def __init__(self):
self.tasks = set()
# The successors of a task are the tasks that depend on it, and can
# only be done once the task is completed.
self.successors = defaultdict(set)
# The predecessors of a task have to be done before the task.
self.predecessors defaultdict (set)
self.completed_tasks = set() # completed tasks.
def add_task(self, t, dependencies):
"""Adds a task t with given dependencies.""
# Makes sure we know about all tasks mentioned.
assert t not in self.tasks or len(self.predecessors[t]) == 0, "The task was already pres
self.tasks.add(t)
self.tasks.update (dependencies)
# The predecessors are the tasks that need to be done before.
self.predecessors[t] = set (dependencies)
# The new task is a successor of its dependencies.
for u in dependencies:
self.successors[u].add(t)
def reset(self):
self.completed_tasks = set()
@property
def done(self):
return self.completed_tasks == self.tasks
def show(self):
"""We use the nx graph to display the graph.""
g = nx.DiGraph()
g.add_nodes_from(self.tasks)
g.add_edges_from([(u, v) for u in self.tasks for v in self.successors[u]])
node_colors = ''.join([('g' if v in self.completed_tasks else 'r').
for v in self.tasks])
nx.draw(g, with_labels=True, node_color=node_colors)
plt.show()
@property
def uncompleted (self):
"""Returns the tasks that have not been completed.
This is a property, so you can say scheduler.uncompleted rather than
scheduler.uncompleted ()"""*
return self.tasks self.completed_tasks
def _check(self):
"""We check that if t is a successor of u, then u is a predecessor
of t.***
for u in self.tasks:
for t in self.successors[u]:
assert u in self.predecessors[t]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc06466e0-b6af-451e-ad3a-a7add978ace4%2F80f459fc-4a57-46ec-a340-ebf2f5e395de%2Ffkf768q_processed.png&w=3840&q=75)
Transcribed Image Text:4
5 class
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
DependencyScheduler(object):
def __init__(self):
self.tasks = set()
# The successors of a task are the tasks that depend on it, and can
# only be done once the task is completed.
self.successors = defaultdict(set)
# The predecessors of a task have to be done before the task.
self.predecessors defaultdict (set)
self.completed_tasks = set() # completed tasks.
def add_task(self, t, dependencies):
"""Adds a task t with given dependencies.""
# Makes sure we know about all tasks mentioned.
assert t not in self.tasks or len(self.predecessors[t]) == 0, "The task was already pres
self.tasks.add(t)
self.tasks.update (dependencies)
# The predecessors are the tasks that need to be done before.
self.predecessors[t] = set (dependencies)
# The new task is a successor of its dependencies.
for u in dependencies:
self.successors[u].add(t)
def reset(self):
self.completed_tasks = set()
@property
def done(self):
return self.completed_tasks == self.tasks
def show(self):
"""We use the nx graph to display the graph.""
g = nx.DiGraph()
g.add_nodes_from(self.tasks)
g.add_edges_from([(u, v) for u in self.tasks for v in self.successors[u]])
node_colors = ''.join([('g' if v in self.completed_tasks else 'r').
for v in self.tasks])
nx.draw(g, with_labels=True, node_color=node_colors)
plt.show()
@property
def uncompleted (self):
"""Returns the tasks that have not been completed.
This is a property, so you can say scheduler.uncompleted rather than
scheduler.uncompleted ()"""*
return self.tasks self.completed_tasks
def _check(self):
"""We check that if t is a successor of u, then u is a predecessor
of t.***
for u in self.tasks:
for t in self.successors[u]:
assert u in self.predecessors[t]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
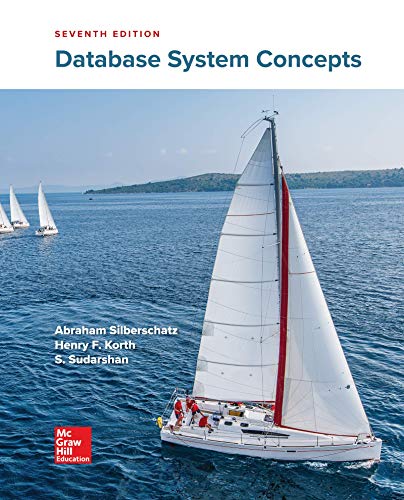
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
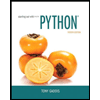
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
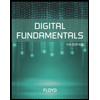
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
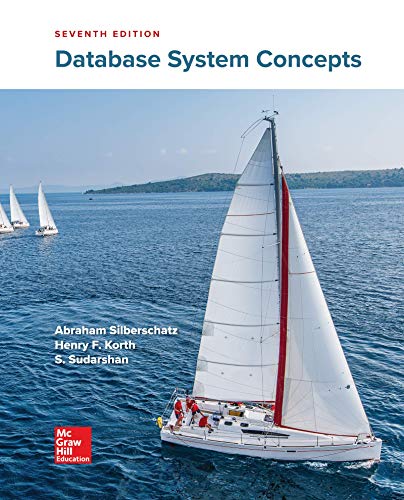
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
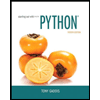
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
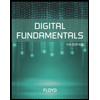
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
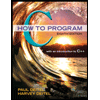
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
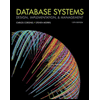
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
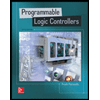
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education