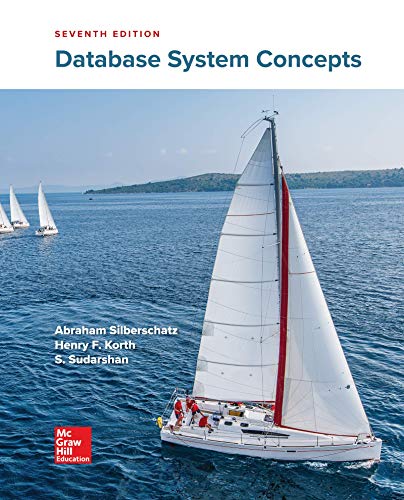
Concept explainers
Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles.
Output each floating-point value with two digits after the decimal point, which can be achieved as follows:
print('%0.2f' % your_value)
Ex: If the input is:
20.0 3.1599
the output is:
1.58 7.90 63.20
Your program must define and call the following driving_cost() function. Given input parameters driven_miles, miles_per_gallon, and dollars_per_gallon, the function returns the dollar cost to drive those miles.
Ex: If the function is called with:
50 20.0 3.1599
the function returns:
7.89975
def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon)
Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400 miles.
Note: This is a lab from a previous chapter that now requires the use of a function.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write a program that asks the user to enter an amount of money in the format of dollars and remaining cents. The program should calculate and print the minimum number of coins (quarters, dimes, nickels and pennies) that are equivalent to the given amount.Hint: In order to find the minimum number of coins, first find the maximum number of quarters that fit in the given amount of money, then find the maximum number of dimes that fit in the remaining amount, and so on. Write a program that prompts for weight in pounds and height in inches, converts the values to metric, and then calculates the BMI.Note: 1 pound is 0.453592 kilograms and 1 inch is 0.0254 meters.arrow_forwardGiven four values representing counts of quarters, dimes, nickels and pennies, output the total amount as dollars and cents. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print(f'Amount: ${dollars:.2f}') Ex: If the input is: 4 3 2 1. where 4 is the number of quarters, 3 is the number of dimes, 2 is the number of nickels, and 1 is the number of pennies, the output is: Amount: $1. 41arrow_forwardDriving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both doubles) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: System.out.printf("%.2f", yourValue); Ex: If the input is: the output is: Note: Real per-mile cost would also include maintenance and depreciation.arrow_forward
- Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print('{:.2f}'.format(your_value)) Ex: If the input is: 20.0 3.1599 the output is: 1.58 7.90 63.20 Your program must define and call the following driving_cost() function. Given input parameters driven_miles, miles_per_gallon, and dollars_per_gallon, the function returns the dollar cost to drive those miles. Ex: If the function is called with: 50 20.0 3.1599 the function returns: 7.89975 def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon) Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400 miles. Instructor note: The program has 2 inputs: miles_per_gallon and dollars_per_gallon. The function has 3 parameters: driven_miles,…arrow_forwardCalories = ( (Age x 0.2757) + (Weight x 0.03295) + (Heart Rate x 1.0781) — 75.4991 ) x Time / 8.368 Write a program using inputs age (years), weight (pounds), heart rate (beats per minute), and time (minutes), respectively. Output the average calories burned for a person. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:System.out.printf("%.2f", yourValue); Ex: If the input is: 49 155 148 60 the output is: Calories: 736.21 caloriesarrow_forwardDriving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print('{:.2f}'.format(your_value)) Ex: If the input is: 20.0 3.1599 the output is: 1.58 7.90 63.20 Your program must define and call the following driving_cost() function. Given input parameters driven_miles, miles_per_gallon, and dollars_per_gallon, the function returns the dollar cost to drive those miles. Ex: If the function is called with: 50 20.0 3.1599 the function returns: 7.89975 def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon) Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400 milesarrow_forward
- The following equation estimates the average calories burned for a person when exercising, which is based on a scientific journal article (source): Calories = ( (Age x 0.2757) + (Weight x 0.03295) + (Heart Rate x 1.0781) — 75.4991 ) x Time / 8.368 Write a program using inputs age (years), weight (pounds), heart rate (beats per minute), and time (minutes), respectively. Output the average calories burned for a person. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print(f'calories: {calories:.2f} calories') Ex: If the input is: 49 155 148 60 then the output is: Calories: 736.21 caloriesarrow_forwardDesign a program that computes pay for employees. Allow a user to continuously input employees’ names until an appropriate sentinel value is entered. Also input each employee’s hourly wage and hours worked. Compute each employee’s gross pay (hours times rate), withholding tax percentage (based on Table 6-4), withholding tax amount, and net pay (gross pay minus withholding tax). Display all the results for each employee. After the last employee has been entered, display the sum of all the hours worked, the total gross payroll, the total withholding for all employees, and the total net payroll. You will need to submit a flowchart and pseudocode for this assignment.arrow_forwardThe following equation estimates the average calories burned for a person when exercising, which is based on a scientific journal article (source): Calories = ( (Age x 0.2757) + (Weight x 0.03295) + (Heart Rate x 1.0781) — 75.4991 ) x Time / 8.368 Write a program using inputs age (years), weight (pounds), heart rate (beats per minute), and time (minutes), respectively. Output the average calories burned for a person. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print(f'Calories: {calories:.2f} calories')arrow_forward
- Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print('{:.2f}'.format(your_value)) Ex: If the input is: 20.0 3.1599 the output is: 1.58 7.90 63.20 Your program must define and call the following driving_cost() function. Given input parameters driven_miles, miles_per_gallon, and dollars_per_gallon, the function returns the dollar cost to drive those miles. Ex: If the function is called with: 50 20.0 3.1599 the function returns: 7.89975 def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon) Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400 miles.arrow_forwardCode in Pythonarrow_forwardMad Libs are activities that have a person provide various words, which are then used to complete a short story in unexpected (and hopefully funny) ways. Complete the program to read the needed values from input, that the existing output statement(s) can use to output a short story. Ex: If the input is: Eric Chipotle 12 cars the output is: Eric went to Chipotle to buy 12 different types of cars. 367012.2549490.qx3zqy7 LAB 2.14.1: LAB: Mad Lib 0/10 ACTIVITY LabProgram.java Load default template... 1 import java.util.Scanner; 2 3 public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String firstName; String genericLocation; int wholeNumber; String pluralNoun; 4 6 7 8 9 10 11 /* Type your code here. */ 12 System.out.println(firstName + } 13 went to + genericlocation + to buy + wholeNumber + differei 14 15arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
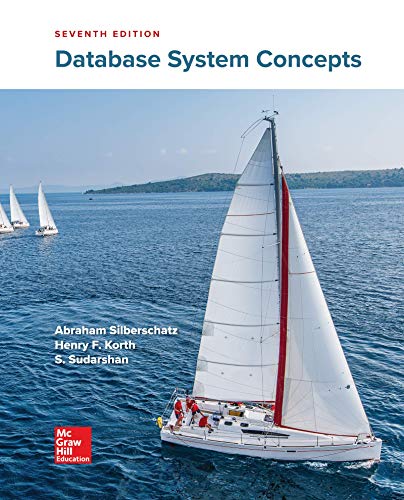
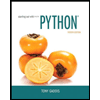
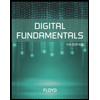
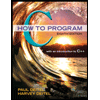
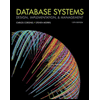
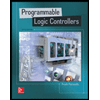