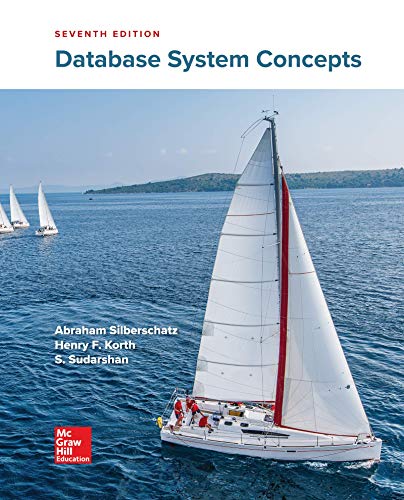
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Change Summation Integers problem to Finding the minimum
problem. Make sure you properly wrote/updated all text messages, method names,
and math calculations.
Hint: You can use java.lang.Math.min() method.
![Below is a transcription and explanation suitable for an educational website regarding the provided Java code for summing integers:
---
### Java Program: Sum of Integers
This code snippet demonstrates how to write a basic Java program to sum either two or three integers based on user input.
#### Code
```java
import java.util.Scanner;
public class ReportSum4 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Prompt the user to enter the number of integers
System.out.print("Enter the number of integers you will provide: ");
int num = sc.nextInt();
// Prompt the user to enter the first integer
System.out.print("Enter the value for 'a' and press Enter: ");
int a = sc.nextInt();
// Prompt the user to enter the second integer
System.out.print("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
if (num == 3) {
// Prompt the user to enter the third integer
System.out.print("Enter the value for 'c' and press Enter: ");
int c = sc.nextInt();
// Display the result
System.out.printf("Provided Integers: %d and %d and %d, the total is %d\n", a, b, c, sum(a, b, c));
} else {
// Display the result
System.out.printf("Provided Integers: %d and %d, the total is %d\n", a, b, sum(a, b));
}
}
public static int sum(int num1, int num2) {
return num1 + num2;
}
public static int sum(int num1, int num2, int num3) {
return num1 + num2 + num3;
}
public static int sum(int num1, int num2, int num3, int num4) {
return num1 + num2 + num3 + num4;
}
}
```
#### Explanation
1. **Imports and Class Declaration**:
- The program imports `java.util.Scanner` to read user input.
- The class `ReportSum4` contains all methods for handling user interactions and calculations.
2. **Main Method**:
- A `Scanner` object `sc` is created to take user input.
-](https://content.bartleby.com/qna-images/question/e4e72d86-d216-4977-8ee6-fe536869a207/8ef4dbb2-23cd-425f-b546-c47180978c32/2jx7gvs_thumbnail.jpeg)
Transcribed Image Text:Below is a transcription and explanation suitable for an educational website regarding the provided Java code for summing integers:
---
### Java Program: Sum of Integers
This code snippet demonstrates how to write a basic Java program to sum either two or three integers based on user input.
#### Code
```java
import java.util.Scanner;
public class ReportSum4 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Prompt the user to enter the number of integers
System.out.print("Enter the number of integers you will provide: ");
int num = sc.nextInt();
// Prompt the user to enter the first integer
System.out.print("Enter the value for 'a' and press Enter: ");
int a = sc.nextInt();
// Prompt the user to enter the second integer
System.out.print("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
if (num == 3) {
// Prompt the user to enter the third integer
System.out.print("Enter the value for 'c' and press Enter: ");
int c = sc.nextInt();
// Display the result
System.out.printf("Provided Integers: %d and %d and %d, the total is %d\n", a, b, c, sum(a, b, c));
} else {
// Display the result
System.out.printf("Provided Integers: %d and %d, the total is %d\n", a, b, sum(a, b));
}
}
public static int sum(int num1, int num2) {
return num1 + num2;
}
public static int sum(int num1, int num2, int num3) {
return num1 + num2 + num3;
}
public static int sum(int num1, int num2, int num3, int num4) {
return num1 + num2 + num3 + num4;
}
}
```
#### Explanation
1. **Imports and Class Declaration**:
- The program imports `java.util.Scanner` to read user input.
- The class `ReportSum4` contains all methods for handling user interactions and calculations.
2. **Main Method**:
- A `Scanner` object `sc` is created to take user input.
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
does not work when I provide # 4 integer.
please assist me with that
Solution
by Bartleby Expert
Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
does not work when I provide # 4 integer.
please assist me with that
Solution
by Bartleby Expert
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write in JAVAarrow_forwardWrite a method (with a parameter n), n: integer type that prints numbers from 1 to n. The print out should be 5 numbers per line. All the output numbers should be aligned well. Call the method with n=13 and n=10 in the main()arrow_forwardWrite a method that takes a RegularPolygon as a parameter, sets its number of sides to a random integer between 10 and 20 inclusive, and sets its side length to a random decimal number greater than or equal to 5 and less than 12. Use Math.random() to generate random numbers. This method must be called randomize() and it must take an RegularPolygon parameter. You can call your method in the program's main method so you can test whether it works, but you must remove or comment out the main method before checking your code for a score. You will also need to make sure you do not remove the import statement "import testing.Math" as this is required to check your code for a score.arrow_forward
- change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));arrow_forwardTASK 8. Repeat TASKs 4-7. Change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));Submit the source code files (.java files) and the console output screenshotsarrow_forwardI need help writing Big Integer Calculator using Java. The Calculator must calculate +,-,*,/, mod, GCF, LCM, and factorial. Create a method for each Arithmetic operation. For sample, calculator check the following link https://www.calculator.net/big-number-calculator.htmlarrow_forward
- change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c,d MinTest(a, b, c,d)); fix below code structurearrow_forwardCreate a method that takes a value and indicate whether it is positive or negative by a return aBoolean value.Declared as: boolean is Positive (float a) in javaarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
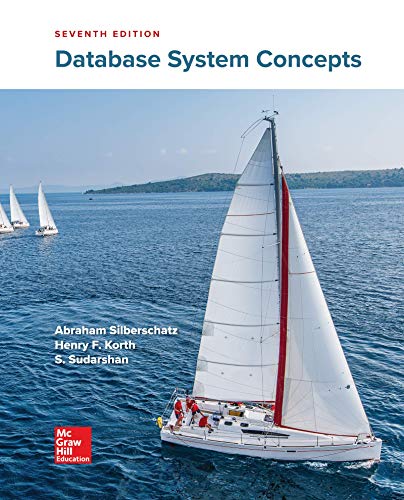
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
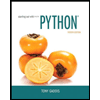
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
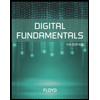
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
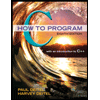
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
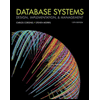
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
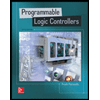
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education