"Dijkstra's single-source shortest path algorithm returns a results grid that contains the lengths of the shortest paths from a given vertex [the source vertex] to the other vertices reachable from it. Develop a pseudocode algorithm that uses the results grid to build and return the actual [shortest] path, as a list of vertices, from the source vertex to a given [target] vertex. (Hint: This algorithm starts with a given vertex [the target vertex] in the grid's first column and gathers ancestor [parent] vertices, until the source vertex is reached.)" *For your algorithm, assume that grid is the name of the results grid produced by Dijkstra's single-source shortest path algorithm. *Each vertex is identified by its label/name, which is in column 1 of grid. *As the first step of your algorithm, find the name of the source vertex. *Next, get the name of the target vertex from the user. Pseudocode should avoid details through broad-stroke statements. However, it must give enough information to outline the overall strategy. In addition to showing your algorithm, answer the following questions: - In pseudocode, to find the source vertex, you can simply write: find source vertex Without providing code, explain how this would be accomplished in real code. - Did you run into any challenges? If so, what were they and how did you solve them? - Besides the given grid, did you have to use any other collection? If so, which one and why? If not, why not?
"Dijkstra's single-source shortest path
*For your algorithm, assume that grid is the name of the results grid produced by Dijkstra's single-source shortest path algorithm.
*Each vertex is identified by its label/name, which is in column 1 of grid.
*As the first step of your algorithm, find the name of the source vertex.
*Next, get the name of the target vertex from the user.
Pseudocode should avoid details through broad-stroke statements. However, it must give enough information to outline the overall strategy.
In addition to showing your algorithm, answer the following questions: - In pseudocode, to find the source vertex, you can simply write: find source vertex Without providing code, explain how this would be accomplished in real code. - Did you run into any challenges? If so, what were they and how did you solve them? - Besides the given grid, did you have to use any other collection? If so, which one and why? If not, why not?
--MUST HAVE A UNIQUE, INDIVIDUAL RESPONSE (please don't copy from another site).--

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

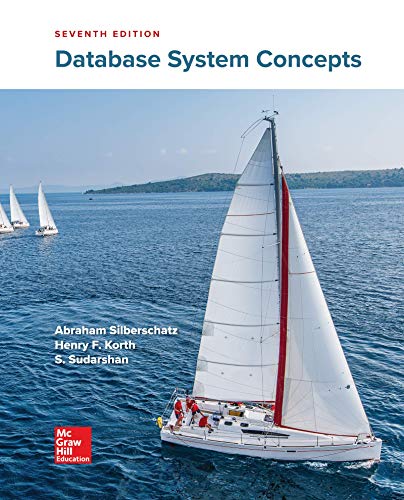
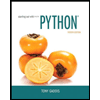
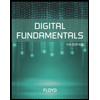
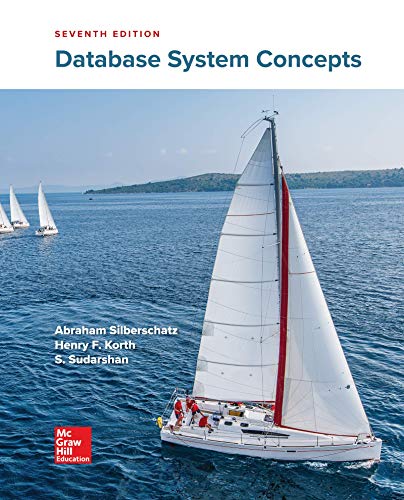
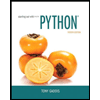
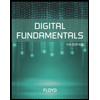
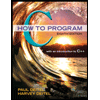
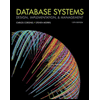
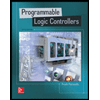