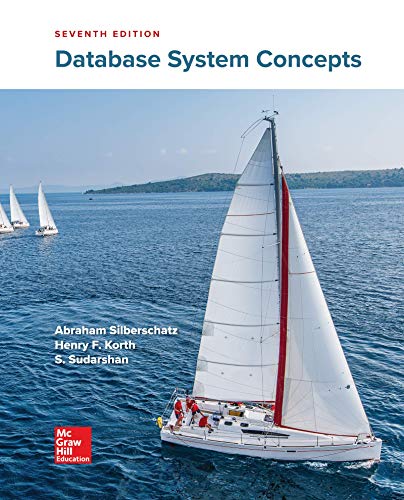
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Iterating over a dictionary example: Gradebook statistics.
Write a program that uses the keys(), values(), and/or items() dict methods to find statistics about the student_grades dictionary. Find the following:
- Print the name and grade percentage of the student with the highest total of points.
- Find the average score of each assignment.
- Find and apply a curve to each student's total score, such that the best student has 100% of the total points.
The language is in Python.
# student_grades contains scores (out of 100) for 5 assignments
student_grades = {
'Andrew': [56, 79, 90, 22, 50],
'Nisreen': [88, 62, 68, 75, 78],
'Alan': [95, 88, 92, 85, 85],
'Chang': [76, 88, 85, 82, 90],
'Tricia': [99, 92, 95, 89, 99]
}
So far I have the first part of the question:
for student, scores in student_grades.items():
print(student, sum(scores)/len(scores))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a prgram that creates a dictionary containing course numbers and room numbers of the room where the courses meet. The dictionary should have the following key value pairs: Course Number Room Number CS101 3004 CS102 4501 CS103 6755 NT110 1244 CM241 1411 This program should also create a dictionary containing course numbers and the names of the instructors that teach each course. The dictionary should have the following key-value pairs: Course Number Instructor CS101 Haynes CS102 Alvarado CS103 Rich NT110 Burke CM241 Lee This program should also create a dictionary containing the course numbers and the meeting times of each course. The dictionary should have the following key values Course number Meeting Time CS101 8:00 a.m. CS102 9:00 a.m. CS103 10:00 a.m. NT110 11:00 a.m. CM241 1:00 p.m. If…arrow_forwardThe following lines of code define three dictionaries for a movie database. They indicate the movies' genre, production year, and budget. The movie titles are dictionary keys in each case. Write a program that prompts the user to enter a search criteria including three elements: 1) a genre, 2) a year, 3) a minimum budget (in million dollars), separated by semicolons (;). Then searches the database and returns a list of all movie titles in the requested genre that are produced within or after the entered year and their budget were equal or greater than the entered minimum. If there is no movie matching the entered criteria just tell the user so. Example 1:Enter genre, starting year, and min budget: drama;2000;5We found these matching movies: ['the pianist','the salesman'] Example 2:Enter genre, starting year, and min budget: action;2020;10Sorry! We could not find a match! # Defining dictionaries (do not change this part of code) genres=…arrow_forwardSize not printing seven after deleting rick // Create a new set with some initial values const words = new Set(['bob', 'john', 'marry', 'hilton', 'bala']); // Add some more values ('rick', 'newton', 'bala') to the set words.add('rick'); words.add('newton'); words.add('bala'); // Check if 'hilton' is in the set words.has('hilton'); // Get the size of the set words.size; // Delete 'rick' from the set words.delete('rick'); // Convert the set to an array const wordsArray = Array.from(words); //Print the set console.log(words); //Print the result to check if 'hilton' is in the set console.log(words.has('hilton')); // print the size of the set console.log(words.size); //Print the array console.log(wordsArray);arrow_forward
- Using the Dictionary below, create a game that picks a random number based on the length of the dictonary. Using that random number, prompts the user for the capital of that state (the key for your dictionary) - Use the code snipet below Your program must prompt the user by asking "What is the capital of XXX:" and XXX is the state name. Your program should compare the guess to the actual capital for that state. If the guess is correct, increment the number of correct guesses. If the guess is wrong, increment the numner of incorrect guesses. When the user types in quit in eithe uppercase or lowercase, the program should print out the results of the game by telling the payer you had XX correct responses and YY incorrect responses. Then the program should end. Make sure to do the Outline and Logic parts for your program. You can include your Python code in yout Word document, or submit it as a Python (;py) file Upload your work to this assignment when completed. # Pick…arrow_forwardRead strings from input until 'stop' is read. For each string read: If the string is a key in dictionary patients_record, delete the key from patients_record and output the string followed by ' deleted'. Otherwise, output the string followed by ' not a key'.arrow_forwardAssume, you have been given a dictionary where the keys present the name of the father and the value is a list of names of the sons of that person. For example A has 3 sons namely X, Y, and Z. Again X has three sons namely E, F, and G. So, A is the grandfather of E, F, and G. family = {"A" : ["X", "Y", "Z"], "B": ["M", "N"], "W" : ["A", "B"], "X" : ["E", "F", "G"]} You need to write a code which takes an input and prints the names of all his grandsons in the format shown in output samples. If he does not have any grandchildren just print “Get your sons married first! Wanna_be_grandpa!!” You can assume the input will always be a key from the dictionary. But we will check your code with a different dictionary. So do not write a code for this particular dictionary only. Enter Assume, you have been given a dictionary where the keys present the name of the father and…arrow_forward
- What's wrong with this cell?arrow_forwardgiven dictionaries, d1 and d2, create a new dictionary with the following property. For each entry(a,b) in d1, if a is not a key of d2 then add (a,b) to the new dictionary. For each entry (a,b) in d2, if a is not a key of d1 then add (a,b) to the new dictionary. For example, if d1 is {2:3, 8:19, 6:4, 5:12} and d2 is {2:5, 4:3, 3:9}, then the new dictionary should be {8:19, 6,4, 5:12, 4:3, 3:9}. using puthonarrow_forwardUse Java pl DataFileWords.txt: lovegentlensslovepeacelovekindnesslovepeaceunderstandingkindnessjoypeaceunderstandingfriendshipgentlenssgoodnesslovepeacegoodnesslovegentlensscontrolunderstandingunderstandinggentlensslovepeacepatiencecontrolkindnessgentlensslovepeacejoygoodnesspeacefriendshipgoodnesskindnesslovepeacegoodnesscontrolpatiencejoygentlensspatiencegentlensspatiencekindnessgoodnessjoygentlenssgentlenssarrow_forward
- In python: student_dict is a dictionary with students' name and pedometer reading pairs. A new student is read from input and added into student_dict. For each student in student_dict, output the student's name, followed by "'s pedometer reading: ", and the student's pedometer reading. Then, assign average_value with the average of all the pedometer readings in student_dict..arrow_forwardFor example, suppose the word “robot” is found in lines 7, 18, 94, and 138. The dictionary would contain an element in which the key was the string “robot”, and the value was a list containing the numbers 7, 18, 94, and 138. Once the dictionary is built, you should prompt the user for a word. If the word is found in the dictionary, then print out all the lines on which they are found in ascending order. The code for the print out is provided in the stub code. A stub code is provided for you. If you do not see it, click the "Load default template" button. # The get_word_dict function returns a dictionary containing# the words from line_list as keys, and their line numbers# as values.def get_word_dict(line_list):# Create a line counter.count = 0 # Create a dictionary to hold the words.word_dict = {} # Step through the list of lines.#######Add you code below############# ####################################### Return the dictionary.return word_dict #####DO NOT CHANGE ANYTHING…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
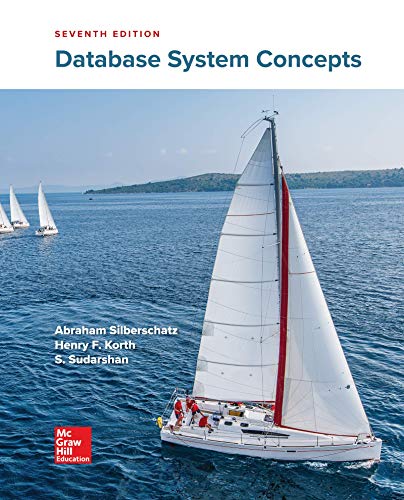
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
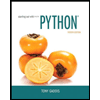
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
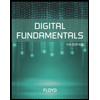
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
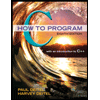
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
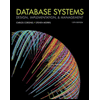
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
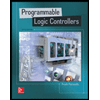
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education