Define a struct menuItemType with two components: menuItem of type string and menuPrice of type double. Write a program to help a local restaurant automate its breakfast billing system. The program should do the following: a. Show the customer the different breakfast items offered by the restaurant. b. Allow the customer to select more than one item from the menu. c. Calculate and print the bill.
Define a struct menuItemType with two components: menuItem of type string and menuPrice of type
double. Write a program to help a local restaurant automate its breakfast billing system. The program
should do the following:
a. Show the customer the different breakfast items offered by the restaurant.
b. Allow the customer to select more than one item from the menu.
c. Calculate and print the bill.
Assume that the restaurant offers the following breakfast items (the price of each item is shown to the
right of the item):
Plain Egg - $1.45
Bacon and Egg - $2.45
Muffin - $0.99
French Toast - $1.99
Fruit Basket - $2.49
Cereal - $0.69
Coffee - $0.50
Tea - $0.75
Use an array menuList of type menuItemType, as defined
Your program must contain at least the following
functions:
- Function getData: This function loads the data into the array menuList.
- Function showMenu: This function shows the different items offered by the restaurant and tells
the user how to select the items.
- Function printCheck: This function calculates and prints the check. (Note that the billing amount
should include a 5% tax.)
A sample output is:
Welcome to Johnny's Restaurant
Bacon and Egg - $2.45
Muffin - $0.99
Coffee - $0.50
Tax - $0.20
Amount Due - $4.14
Format your output with two decimal places. The name of each item in the output must be left justified.
You may assume that the user selects only one item of a particular type.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

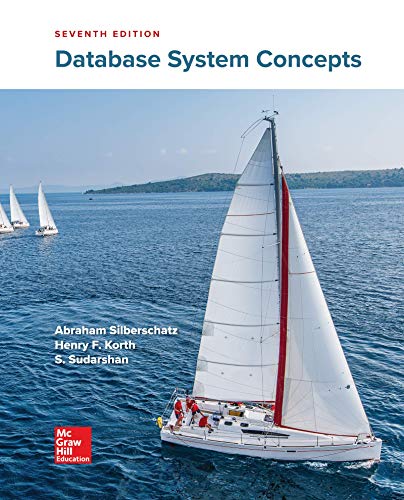
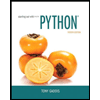
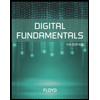
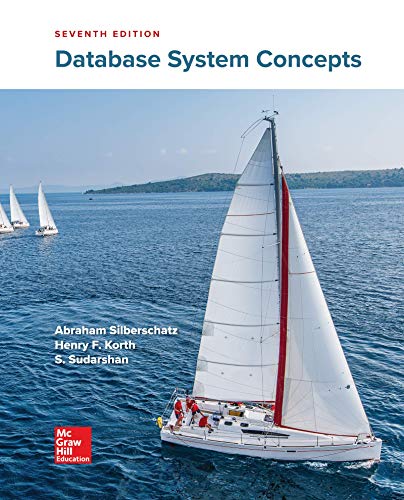
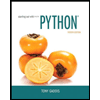
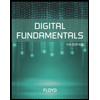
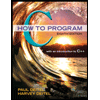
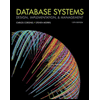
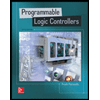