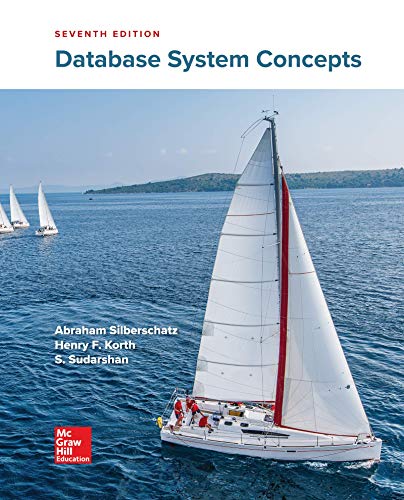
Java Built-in Comparator interface, Java Built-in Collections class : A simple case of how to sort LinkedList using Comparator. Please use Java Built-in LinkedList class. (https://docs.oracle.com/javase/8/docs/api/allclasses-noframe.html
Please make a summary for Java built-in Comparator interface & Collections class (especially sort(list, c) methods in Collections class).
Define a Person class which has name and age. Also define an ageComparator class which implements Comparator interface to compare the age of people.
Please create 5 instances from Person class and put them into a LinkedList at any order.
Sort them by their ages in ascending order, and print them.
You can put the pseudo code here (or you can write in Java code and submit the Java file with its output).
)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardThe two classes you will create will implement the operations defined in the interface as shown in the UML class diagram above. In addition, BinarySearchArray will implement a static method testBinarySearchArray() that populates the lists, and lets the user interactively test the two classes by adding and removing elements. The parameter BinarySearch can represent either class and tells testBinarySearchArray which class to test. Steps to Implement: 1) To get started create a new project in IntelliJ called BinarySearch. Add a class to your project called BinarySearchArray. Then add another class BinarySearchArrayList and an interface called BinarySearch. The interface BinarySearch includes public method stubs as shown in the diagram. You are allowed to add BinarySearchArrayList to the same file as BinarySearch but don't add an access modifier to this class, or for easier reading, you can declare the classes in separate files with public access modifiers. Only the class…arrow_forward1. fast please in c++arrow_forward
- Java Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardLooking at all four list implementations, which actions/methods tend to be less efficient in the Linked List implementation compared to the Array Implementation? Explain why for each action/method you specify.arrow_forwardPlease give a detailed answer and code examples for all of the questions below.What is an array and linked list?What the difference between array and List?Difference between ArrayList and Arrays in Java?arrow_forward
- In Java. The following is a class definition of a linked list Node:class Node{int info;Node next;}Show the instructions required to create a linked list that is referenced by head and stores in order, the int values 13, 6 and 2. Assume that Node's constructor receives no parameters.arrow_forwardIn Java, Explain when it might be preferable to use a map instead of a set.arrow_forwardWhat is an iterator? Why are iterators often used with linked lists?arrow_forward
- Starter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forwardSimple JAVA linkedlist code implementation please help and complete any part you can - Without using the java collections interface (ie do not import java.util.List,LinkedList, Stack, Queue...)- Create an implementation of LinkedList interface- For the implementation create a tester to verify the implementation of thatdata structure performs as expected Build Bus Route – Linked List- Your task is to:o Implement the LinkedList interface (fill out the implementation shell)o Put your implementation through its paces by exercising each of themethods in the test harnesso Create a client (a class with a main) ‘BusClient’ which builds a busroute by performing the following operations on your linked list:o§ Create (insert) 4 stations§ List the stations§ Check if a station is in the list (print result)• Check for a station that exists, and onethat doesn’t§ Remove a station§ List the stations§ Add a station before another station§ List the stations§ Add a station after another station§ Print the…arrow_forwardAdd the following method in the BST class that returns aniterator for traversing the elements in a BST in preorder./** Return an iterator for traversing the elements in preorder */java.util.Iterator<E> preorderIterator()arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
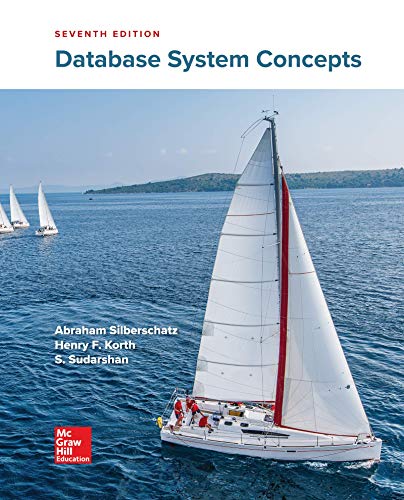
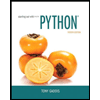
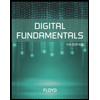
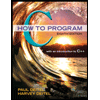
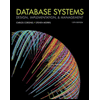
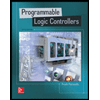