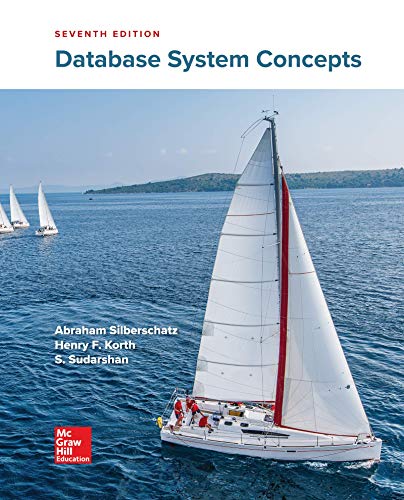
Concept explainers
Creating Packages and Importing Classes
The purpose of this lab is for you to learn how to become proficient in creating packages in Java and being able to import these packages into classes in other packages. Management of different source code files and folders is key to programming organization, scalable project size, and promoting reusability in software development.
Steps:
- Create a new Java Project named “Packages” from within Eclipse.
- Following the example in the Week 6 lecture, create six packages: Main, add, subtract, multiply, divide, and modulo. ALL of these packages should be within the “src” folder in the Eclipse package Explorer. ALL of the packages should be on the same “level” in the file hierarchy.
- In the “Main” package, create the “Lab04.java” file and add the needed boilerplate (“Hello, World!” style) code to create the main method for the application.
- For each of the arithmetic packages (add, subtract, multiply, divide, and modulo), create a single class (you should name these files the same as the package name but capitalized; e.g. “Add.java”, “Subtract.java”, “Multiply.java”, etc.)
- Follow the specifications below for detailed implementation details:
Lab 4 Specifications
Inside each of the arithmetic classes (Add, Subtract, Multiply, Divide, Modulo), make sure EACH class contains the following:
Two private fields
// Example Fields:
private int n1;
private int n2;
A constructor that passes two values that can be saved in the private fields
// Example Constructor:
public Add(int n1, int n2){
this.n1 = n1;
this.n2 = n2;
}
The following three methods must be supported by ALL arithmetic classes:
public int getResult(); // This should return the result of n1 arithmetic operator n2 // n1 + n2 for add
public void changeVals(int n1, int n2); // This should change or “reset” the values in the
// private class fields
public String toString(); // This returns a string that displays the equation with result
// e.g. “3 + 5 = 8”
Inside the “Lab04.java” file should contain the following:
All package and import statements needed to reference the other packages and classes.
Inside the main method, create class objects for each of arithmetic classes
// Example:
Add a = new Add(5, 3);
For each class object, make sure to ALSO print out the toString method of each to verify the current equation, call the method to change the values, and print out the toString method again to verify the changes.
// Example:
System.out.println(a.toString());
a.changeVals(4, 4);
System.out.println(a.toString());
Verify, by running the program, that everything works as expected.

Step by stepSolved in 4 steps with 2 images

- You are going to create a game or application that utilizes many of the features of the course. Youare to build a single game or application that is built using an object-oriented paradigm and utilizesmany of the features from the course. Most of these features you will come across naturally whilebuilding your game or application so picking the right project is the most important part early on. Notonly will you code the project, but you will also develop a short video of you using the game orapplication and fill out a series of short written responses relating to your program and program code.You are to use our graphics library for this project. Using tKinter or otherlibraries will need written consent from your instructor and pyGame is off limits.Part A: Project Code Requirements:1. Object Oriented: There is at least one class and one instance of that class created to simplifyand encapsulate the actions of the program.2. Graphical: Interaction with the user must be graphical and not…arrow_forwardOnce you and your team have settled on a method, the following step is to choose a programming language to use for the project. Is there a particular language that you think would be better suited to the implementation of your strategy?arrow_forwardFor most applications, a team of developers works together building code for specific features and functionality. This collaboration provides numerous benefits to the developers as well as the users of the finished product. What are some of the benefits of team-based approach to application development? What are some of the challenges to this approach and how can a development manager prevent these from negatively impacting the final product? What are some "good programming principles" that you can implement to help others work with the code you write? Are you able to implement these practices for your weekly programming assignment? (Provide justification for your response regardless of which position you choose to take.)arrow_forward
- What concerns arise when mixing packages with different "licenses" in a project?arrow_forwardWhat is the purpose of the sandbox model?arrow_forwardSuppose you are working on an application. You want to make sure that everyone can use the application. What is the best practice for testing your application? A) a homogenous group of testers B) themselves as testers C) testers having specific skill set D) a diverse group of testersarrow_forward
- This is to be done in Java. Only need to do Task Class and Task Service For Project One, which is due in Module Six, you are asked to develop a mobile application for a customer. The customer will provide you with the requirements. Your job is to code the application and provide unit tests to verify that the application meets the customer’s requirements. For this milestone, you will focus on delivering the task services. The purpose of these services is to add, update, and delete task objects within the application. The task service uses in-memory data structures to support storing tasks (no database required). In addition, there is no user interface for this milestone. You will verify the task service through JUnit tests. The task service contains a task object along with the task service. The requirements are outlined below. Task Class Requirements The task object shall have a required unique task ID String that cannot be longer than 10 characters. The task ID shall not be null and…arrow_forwardHello! I need help with the first problem, here are the requirements! Please use Eclipse if possible! and showing a screenshot of it would also be helpful. Also comments displaying what the code is also very helpful so I understand it. Thank you so much!!! Assignment Requirements Create a new Java Project in your Eclipse workspace named as before, smith3 or jones3 for example. Create a package with the very same name as your project name. In this one package, write one Java program for each exercise as required below. Choose descriptive variable names and identifiers in all programs 3. Write a program that deliberately contains an endless or infinite while loop. The loop should generate multiplication questions with single digit random integers. Users can answer the questions and get immediate feedback. After each question, the user should be able to stop the questions and get an overall result. See Example Output. Example Output What is 7 * 6 ? 42 Correct. Nice work! Want more…arrow_forwardQuestion 4. C# I have the project done, i’m just not sure if the output is right or not. Help would be appreciated!arrow_forward
- Why is it essential to understand the "package's API stability" before integrating it into a project?arrow_forwardWhat do you call the programming method that starts with a very simple yet fully-functional product and then gradually adds more complex functionality?arrow_forwardIdle thought While building the framework, anticipate issues. After all. This mean? various obstacles as you build the framework. So anything. This mean?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
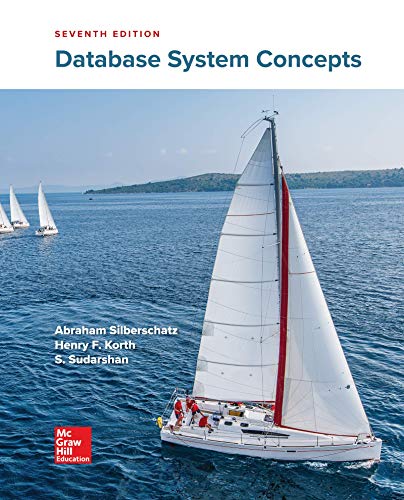
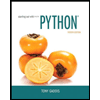
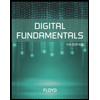
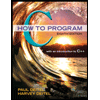
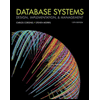
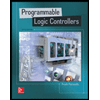