#Create binarysort158: main.o merge.o mergesort.o wrt.o gcc -o sort158 main.o merge.o mergesort.o wrt.o -lm #Create object file for main.cmain.o: main.c mergesort.h gcc -c main.c #Create object file for merge.cmerge.o: merge.c mergesort.h gcc -c merge.c #Create object file for mergesort.cmergesort.o: mergesort.c mergesort.h gcc -c mergesort.c wrt.o: wrt.c gcc -c wrt.c #Delete the all of the object filesclean: rm *.o sort158 #Create object file for wrt.c 6. Error: The makefile wont compile7. Error: I need to make the files to compile with eachothersort158a.c (main.c) #include "mergesort.h" int main(void){ int sz, key[] = { 4, 3, 1, 67, 55, 8, 0, 4, -5, 37, 7, 4, 2, 9, 1, -1 };int n = sizeof(key) / sizeof(int); /* the size of key [] */int i; /*remove first 4 elements from key [] */for(i = 4; i < n; i++) {key[ i - 4] = key[i]; } sz = n - 4; /* Update the size of key [] */wrt(key, sz); /* Print the contents of the orginal array */ mergesort(key, sz); /* Sort the array using mergesort */printf("After mergesort:\n"); /*prints "After mergesort:"*/wrt(key, sz); // Print the contents of the sorted array /* Restore first 3 elements in key[] */key[0] = 4;key[1] = 3;key[2] = 1;key[3] = 67;sz = n; return 0;}Merge.c #include "mergesort.h" void merge(int a[], int b[], int c[], int m, int n){ int i = 0, j = 0, k = 0; /* Loop through both arrays and compare their elements to eachother */ while (i < m && j < n) if (a[i] < b[j]) c[k++] = a[i++]; else c[k++] = b[j++]; /* If there are any remaining elements in array b[]. copy them to array c[]. */ while (i < m) /* pick up any remainder */ c[k++] = a[i++];/* If there are any remaining elements in array a[], copy them to array c[]. */ while (j < n) c[k++] = b[j++];}mergesort.h #include "mergesort.h"void mergesort(int key[], int n){int j, k, m, *w; // Find the largest power of 2 less than or equal to nfor (m = 1; m < n; m *= 2) /* m is a power of 2 */ ;// Check if n is a power of 2, if not exit with error messageif (n < m) { printf("ERROR: Array size not a power of 2 - bye!\n"); exit(1);}w = calloc(n, sizeof(int)); /* allocate workspace */assert(w != NULL); /* check that calloc() worked */ //Sort the array using mergesortfor (k = 1; k < n; k *= 2) { for (j = 0; j < n - k; j += 2 * k)/* merge two subarrays of key [] into a subarray of w[]. */ merge(key + j, key + j + k, w + j, k, k); for (j = 0; j < n; j++) key[j] = w[j]; /* write W back into key */ } free (w); /*free the workspace*/}Mergesort.h #include <assert.h>#include <stdio.h>#include <stdlib.h> // This function merges two sorted arrys a and b into a sorted array c.// paterameters: a - c sorts its array. m: is the elements in a n: is the number of elements in bvoid merge(int a[], int b[], int c[], int m, int n); //This function sorts an array of integers using the merge sort algorithm//key: the array of integers to be sorted//n: the number of elements in the arrayvoid mergesort(int key[], int n);//This function writes an array of integers//key: the array of integers to be written//sz: the number of elements in the arrayvoid wrt(int key [], int sz); wrt.c QUESTION: create a Makefile for this coding assignment please
#Create binary
sort158: main.o merge.o mergesort.o wrt.o
gcc -o sort158 main.o merge.o mergesort.o wrt.o -lm
#Create object file for main.c
main.o: main.c mergesort.h
gcc -c main.c
#Create object file for merge.c
merge.o: merge.c mergesort.h
gcc -c merge.c
#Create object file for mergesort.c
mergesort.o: mergesort.c mergesort.h
gcc -c mergesort.c
wrt.o: wrt.c
gcc -c wrt.c
#Delete the all of the object files
clean:
rm *.o sort158
#Create object file for wrt.c
6. Error: The makefile wont compile
7. Error: I need to make the files to compile with eachother
sort158a.c (main.c)
#include "mergesort.h"
int main(void)
{
int sz, key[] = { 4, 3, 1, 67, 55, 8, 0, 4,
-5, 37, 7, 4, 2, 9, 1, -1 };
int n = sizeof(key) / sizeof(int); /* the size of key [] */
int i;
/*remove first 4 elements from key [] */
for(i = 4; i < n; i++) {
key[ i - 4] = key[i];
}
sz = n - 4; /* Update the size of key [] */
wrt(key, sz); /* Print the contents of the orginal array */
mergesort(key, sz); /* Sort the array using mergesort */
printf("After mergesort:\n"); /*prints "After mergesort:"*/
wrt(key, sz); // Print the contents of the sorted array
/* Restore first 3 elements in key[] */
key[0] = 4;
key[1] = 3;
key[2] = 1;
key[3] = 67;
sz = n;
return 0;
}
Merge.c
#include "mergesort.h"
void merge(int a[], int b[], int c[], int m, int n)
{
int i = 0, j = 0, k = 0;
/* Loop through both arrays and compare their elements to eachother */
while (i < m && j < n)
if (a[i] < b[j])
c[k++] = a[i++];
else
c[k++] = b[j++];
/* If there are any remaining elements in array b[]. copy them to array c[]. */
while (i < m) /* pick up any remainder */
c[k++] = a[i++];
/* If there are any remaining elements in array a[], copy them to array c[]. */
while (j < n)
c[k++] = b[j++];
}
mergesort.h
#include "mergesort.h"
void mergesort(int key[], int n)
{
int j, k, m, *w;
// Find the largest power of 2 less than or equal to n
for (m = 1; m < n; m *= 2) /* m is a power of 2 */
;
// Check if n is a power of 2, if not exit with error message
if (n < m) {
printf("ERROR: Array size not a power of 2 - bye!\n");
exit(1);
}
w = calloc(n, sizeof(int)); /* allocate workspace */
assert(w != NULL); /* check that calloc() worked */
//Sort the array using mergesort
for (k = 1; k < n; k *= 2) {
for (j = 0; j < n - k; j += 2 * k)
/* merge two subarrays of key [] into a subarray of w[]. */
merge(key + j, key + j + k, w + j, k, k);
for (j = 0; j < n; j++)
key[j] = w[j]; /* write W back into key */
}
free (w); /*free the workspace*/
}
Mergesort.h
#include <assert.h>
#include <stdio.h>
#include <stdlib.h>
// This function merges two sorted arrys a and b into a sorted array c.
// paterameters: a - c sorts its array. m: is the elements in a n: is the number of elements in b
void merge(int a[], int b[], int c[], int m, int n);
//This function sorts an array of integers using the merge sort
//key: the array of integers to be sorted
//n: the number of elements in the array
void mergesort(int key[], int n);
//This function writes an array of integers
//key: the array of integers to be written
//sz: the number of elements in the array
void wrt(int key [], int sz);
wrt.c
QUESTION: create a Makefile for this coding assignment please
Unlock instant AI solutions
Tap the button
to generate a solution
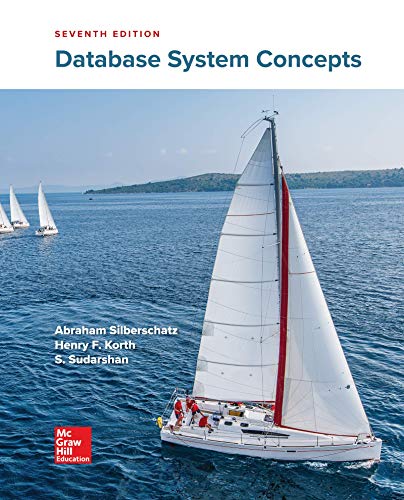
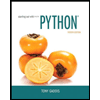
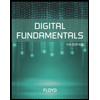
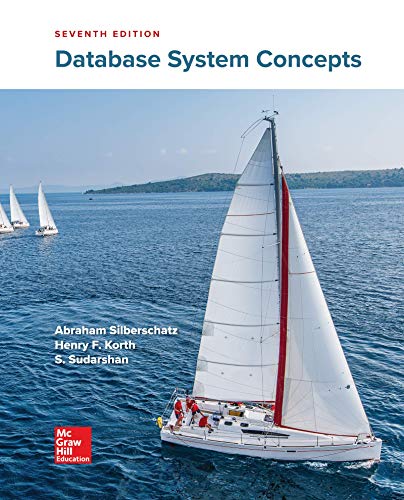
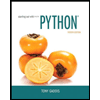
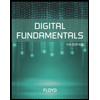
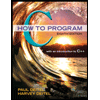
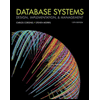
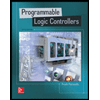