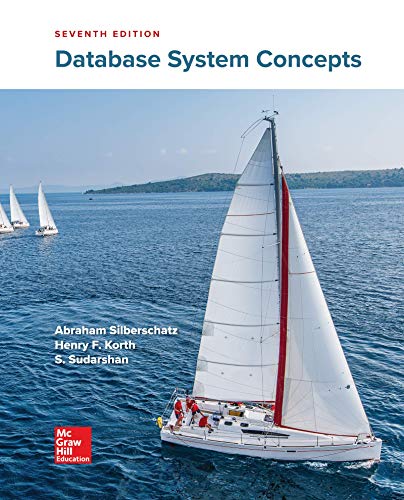
Concept explainers
- Create an array of 100 integers using a random generator. ( 1 for loop)
- Provide the sum and average of the array. (1 for loop)
- Find the smallest and largest number in the array. Determine its position in the array. (2 for loops)
- How many numbers are below the average, and how many numbers are above the average. (1 for loop)
- Print all data to the console, but do not print the array to the console.
So this is what I have so far.
import java.util.Scanner; *do I need this?*
import java.util.Random;
import java.util.Arrays;
public class MainClass
{
public static final int N = 100;
public static int[] data;
public static int sum;
public static int min;
public static int max;
public static double avg;
public static void main(String[] args)
{
Random rndGen= new Random(-1); *is this necessary for random gen?*
sum = 0;
int[] data = new int [N]; * do I need this even though I declared at beginning
for(int i = 0; i < N; i++)
// populate array
//calculate array
//calculate statistics
//calculate sum or array
//display in data
System.out.println("The Max array is " + Max);
System.out.println("The Min array is " +Min);
System.out.println("The sum of the array is " + Sum);
System.out.println("The average of the array is " + Avg);
System.out.println("The position of the smallest and largest in the array is" +location);
System.out.println("There are +numbersBelow+ " below the average, and " +numbersAbove+ "above the average");

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- A for loop is commonly used to iterate over all elements of an array.A. True B. Falsearrow_forward(FYI: Pseudocode is required (Not any programming language) Design a pseudocode program that loads an array with the following 7 values. Add one more word (of your own choosing) for a total of 8 words. biffcomelyfezmottleperukebedraggledquisling Be sure to use lowercase, as shown above. This will make the processing easier. Ask the user to enter a word Search through this array until you find a match with the word the user entered. Once you find a match, output "Yes, that word is in the dictionary". If you get to the end of the array and do NOT find a match, output "No, that word is not in the dictionary". The program should work with any set of words in the arrays. If I were to change the words in the arrays, it should still work. If you need help, look at the search example in your textbook.arrow_forwardpython exercise Given the following list: testlist2 = (23,50,12,80,10,90,34,78,4,19,53) Use testlist2 to create Numpy array of int16 and then display the array. Display the number of dimension of testnp. Display the shape of testnp. Display the number of bytes of testnp. Display the mean, sum, max, and min of testnp.arrow_forward
- Problem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forwardTake arr as any integer array of any size.arrow_forwardProgram: Using a multidimensional array, create a triangular-shaped array. You will ask the user how many lines they want to see and then create the array, fill it, and then print it. You will fill the array with one 1 in the first row, two 2’s in the second row, etc. This should work for any integer that the user enters. (Just because I am starting on 1 does not mean row 0 was skipped.) You must: use a loop to create the array shape. You must: use nested for loops to fill the array and to print the values back to the screen. Your program should print as shown below. Example Output: How many lines would you like in your triangle? >>>9 1 2 2 3 3 3 4 4 4 4 5 5 5 5 5 6 6 6 6 6 6 7 7 7 7 7 7 7 8 8 8 8 8 8 8 8 9 9 9 9 9 9 9 9 9 If your code looks like the code below, it is not what I’m asking for. The code below is making a square multidimensional array, not a triangular one. The code below is just leaving certain spots empty so that it looks like a triangle. I will ask you…arrow_forward
- CODE IN JAVA design a program that grades arithmetic quizzes as follows: (Use the below startup code for Quizzes.java and provide code as indicated by the comments) 1. Ask the user how many questions are in the quiz. 2. Use a for loop to load the array. Ask the user to enter the key (that is, the correct answers). There should be one answer for each question in the quiz, and each answer should be an integer. They can be entered on a single line, e.g., 34, 7, 13, 100, 8 might be the key for a 5-question quiz. You will need to store the key in an array called "key". 3. Ask the user to enter the student's answers for the quiz to be graded. There needs to be one answer for each question. Note that each answer can simply be compared to the key as it is entered. If the answer is correct, add 1 to a correct answer counter. need this in a separate for loop from the loop used to load the array. 4. When the user has entered all of the answers to be graded, print the number correct…arrow_forwardHello, Stuck on this question. All programming is in Python. 2.) Design a program that generates a 7-digit lottery number. The program should have an INTEGER array with 7 elements. Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Then write another loop that displays the contents of the array. All programming is in Python.arrow_forwardcan you please write this in java.util.scanner form and can you make it so i can copy and past it, thank you.can you please write this in java.util.scanner form and can you make it so i can copy and past it, thank you. 2D Array OperationsWrite a program that creates a two-dimensional array initialized with test data. Use anyprimitive data type that you wish. The program should have the following methods:• getTotal. This method should accept a two-dimensional array as its argument andreturn the total of all the values in the array.• getAverage. This method should accept a two-dimensional array as its argument andreturn the average of all the values in the array.• getRowTotal. This method should accept a two-dimensional array as its first argumentand an integer as its second argument. The second argument should be the subscriptof a row in the array. The method should return the total of the values in thespecified row.• getColumnTotal. This method should accept a two-dimensional array…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
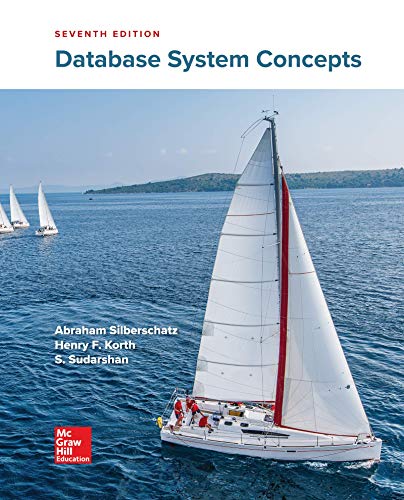
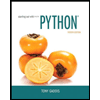
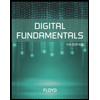
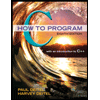
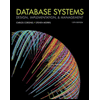
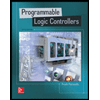