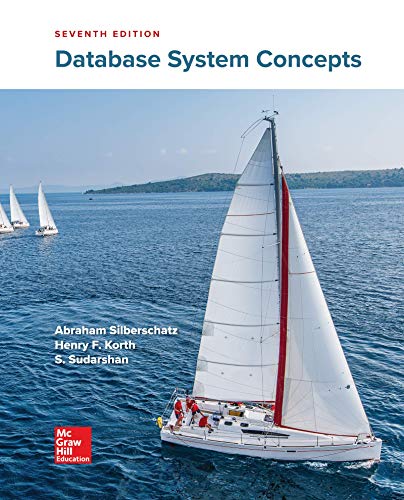
Create a php file for this task. Depending on whether "Top Scorers" or "Leaders" has been clicked, you need to display the top 10 teams sorted by their total scores or total points earned. You have to read the whole CSV file when calculating total scores and total points.
Top Scorers
Display a table with two columns: Team and Scores. The team that has the highest number of scores is displayed first. You have to display the top 10 teams only.
Note: in the CSV file, for the first row, you will see something like
Arsenal FC,4-3,Leicester City FC
that means for this particular match, Arsenal FC scored 4 and Leicester City FC scored 3. This rule applies to all other rows as well. For this "Top Scorers" display, you need to calculate the total score of every team and then display the top 10 teams.
Leaders
Display a table with two columns: Team and Points. The team that has the highest number of points is displayed first. You have to display the top 10 teams only.
Note: in the CSV file, for the first row, you will see something like
Arsenal FC,4-3,Leicester City FC
that means for this particular match, Arsenal FC scored 4 and Leicester City FC scored 3, so Arsenal FC wins this match. For a match, the winner earns 3 points, the loser earns zero points. If the match is a draw, both teams earn 1 point. This rule applies to all other rows as well. For this "Leaders" display, you need to calculate the total point of every team and then display the top 10 teams.
https://raw.githubusercontent.com/TriDang/cosc2430-2022-s2/main/data/football.csv
Hint 1: maintain an associative array like this
[
'team 1 name' => ['scores' => calculated_score, 'points' => 'calculated points'],
'team 2 name' => ['scores' => calculated_score, 'points' => 'calculated points'],
'team 3 name' => ['scores' => calculated_score, 'points' => 'calculated points']
]
In other words, use team names as the keys for the array. The value of each key is itself another array
Hint 2: loop through the CSV file
If the team name has not existed yet => add a new array element and assign calculated scores and calculated points as zeros.
Extract the FT column to get the score of each team. Add the score to each team
Based on the score of each team, determine the winner, loser, or drawer. Add appropriate points to each team
Hint 3: now you have an array of teams with all the needed information, just create 2 comparison functions and use the sort function to sort the teams.
Hint 4: use fgetcsv() to read each row; use explode() to extract the score of each team.

Step by stepSolved in 5 steps with 3 images

- JQuery selector $("#test").hide(); This will hide following please select from choice below. O This is a paragraph. O This is a paragraph. O This is paragraph.arrow_forwardWrite a regular expression that matches the day of year such as "1-1-2018" or "7/4/1776". Dates like "9-31-1955", "3/29-2018", and"5-28/1956" should not be matched. You do not have to worry about leap years (i.e. assume February has 28 days every time). Edit View Insert Format Tools Table 12pt v Paragraph v A To 田 画 D O wordsarrow_forwardThe Movie table has the following columns: ID - positive integer Title - variable-length string Genre - variable-length string RatingCode - variable-length string Year - integer Write ALTER statements to make the following modifications to the Movie table: Add a Producer column with VARCHAR data type (max 50 chars). Remove the Genre column. Change the Year column's name to ReleaseYear, and change the data type to SMALLINT.arrow_forward
- # "New" means new compared to previous level provincial capitals = { 'Nunavut': 'Iqaluit', 'Ontario': 'Toronto', 'Yukon': 'Whitehorse', 'Manitoba': 'Winnipeg' } province_name = input () while province_name != 'exit': if province_name in provincial_capitals: print (provincial_capitals [province_name]) del provincial_capitals [province_name] # New line else: Type the program's output print ('x') province_name input () Input Yukon Maine Yukon Ontario exit Outputarrow_forwardHW2b: R Exercises 1 Complete R Swirl 2 first! It will help you in this assignment! Instructions: Write one R script title HW26.R that contains your answers to problems 1- 5. At the top of the script write your name in a comment. Label each problem number using a comment and then type the code that solves that problem. Here's an example of the script format (where you should fill in the R code to solve each problem): 1 # Your Name # Assignment 3 , # Thu Feb 03 07:39:07 2022 2 4 5 # 1. 6 7 type the R commands solve problem 1 9. # 2. 10 11 type the R commands to solve problem 2 12 13 # 3. |14 15 etc. 1. What are the arguments for the Sequence Generation function seq() and give an example. Hint, use the methods you have learned to search for help in RStudio. 2. Use R as a calculator to compute the following values. а. 27(38-17) b. In(147) 436 V С. 12 3. Create the following vectors in R and use R commands to answer the questions. а 3 (5, 10, 15, 20, ..., 160) b = (87, 86, 85,..., 56)arrow_forwardR-Studio 1.) You have an airport routes.csv file that contains 3409 airports, each row has a 3-letter code and the number of routes. Example: Airport NumberOfRoutes AAE 9 AAL 20 AAN 2 AAQ 3 AAR 8 AAT 2 AAX 1 AAY 1 ABA 4 ABB 2 ABD 6 ABE 13 ABI 2 ABJ 49 ABL 4 ABM 1 ABQ 42 ABR 1 ABS 1 ABT 3 ABV 30 ABX 4 ABY 4 ABZ 41 ACA 8 ACC 54 ACE 116 ACH 2 ACI 2 ACK 6 ACR 2 ACT 2 ACU 1 ACV 3 ACX 6 ACY 10 ACZ 1 ADA 17 ADB 66 ADD 105 ADE 23 ADF 2 ADK 1 ADL 51 ADQ 11 ADU 3 ADZ 11 AEB 3 AEP 65 For this dataset, consider the following models:(a) Suppose the given data points follow a power law distribution. Estimate the corresponding α parameter. (b) Suppose the given data points follow an exponential distribution. Estimate the corresponding λ parameter. (c) Suppose the given data points follow a uniform distribution. Estimate the corresponding range parameters [a, b] of the uniform distribution.(d)…arrow_forward
- # "New" means new compared to previous level provincial_capitals 'Yukon': 'Whitehorse', 'Manitoba': 'Winnipeg', 'BC': 'Victoria', 'Ontario': 'Toronto' input () while province_name != 'exit': province_name if province_name in provincial_capitals: print (provincial_capitals [province_name]) del provincial_capitals [province_name] # New line else: print ('x') province_name input () U Input Yukon BC Yukon lowa exit Outputarrow_forwardGiven num_rows and num_cols, output the label for each seat of a theater. Each seat is followed by a space, and each row is followed by a newline. Define the outer for loop, initialize curr_col_let with the starting row letter, and define the inner for loop. If the input is: 23 1A 1B 1C 2A 2B 2C Notes: • Rows are in ascending order. Seats in the first row all start with the integer 1. • Columns are in alphabetical order. Seats in the first column all start with the letter 'A'. print(x, end="), where " are two single quotes, outputs x without ending with a default newline. chr(ord(letter) + 1) can be used to increment letters. ● ● Learn how our autograder works 487180.3542414.qx3zqy7 1 num_rows = int(input()) 2 num_cols int(input()) 123456 = print (f'{curr_row}{curr_col_let}', end=' ') curr_col_let = chr(ord(curr_col_let) + 1) 7 8 print()arrow_forwardInclude a formula in the last column on the third row to calculate 75% of the Cost. The formula is =0.75*b3. You do not need to select a number format. Include a formula in the next two rows of the same column, adjusting the row reference as necessary.arrow_forward
- 3. Which among the following shows a valid use of the Direction enumeration as a parameter to the moveCharacter function? Select al that apply. enum Direction { case north, south, west, east}func moveCharacter(x: Int, y: Int, facing: Direction) {// code here} moveCharacter(x: 0, y: 0, facing: .southwest) moveCharacter(x: 0, y: 0, facing: Direction.north) moveCharacter(x: 0, y: 0, facing: .south) moveCharacter(x: 0, y: 0, facing: Direction.northeast)arrow_forwardAdd formulas to complete the table of hours used. In cell B17, create a nested formula with the IF and SUM functions that check if the total number of hours worked in week 1 (cells B9:F9) is equal to 0. If it is, the cell should display nothing (indicated with two quote marks: ""). Otherwise, the cell should display the total number of hours worked in week 1. Copy the formula from cell B17 to fill the range B18:B20arrow_forwardYou are given 2 text files named drywet.txt and wetwet.txt. wetwet.txt data file refers to the probability of next day being a wet day if the current day is wet. drywet.txt data file refers to the probability of next day being a wet day if the current day is dry. NOTE: the data for the same location in wetwet.txt and drywet.txt will have the same line number. These files are in the format of: (excerpt from wetwet.txt)-97.58 26.02 0.76 0.75 0.77 0.74 0.80 0.86 0.94 0.97 0.89 0.77 0.74 0.77 -97.19 26.03 0.73 0.76 0.75 0.71 0.79 0.85 0.92 0.95 0.90 0.81 0.76 0.75 -98.75 26.35 0.74 0.76 0.76 0.73 0.67 0.84 0.83 0.85 0.80 0.71 0.71 0.76 … In each line, the first and second numbers represent the location’s longitude and latitude. The following 12 numbers represent the probability of the next day being a wet day of the month. For example, on the first line of the excerpt above 0.75 means that in February (4th column), there is a 75% of chance that the next day is a wet day if today is a wet…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
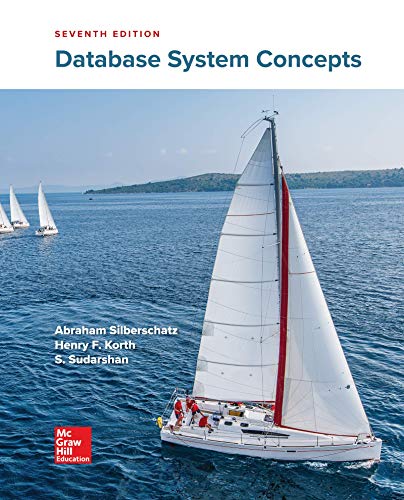
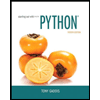
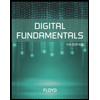
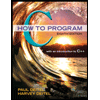
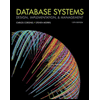
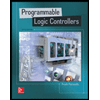