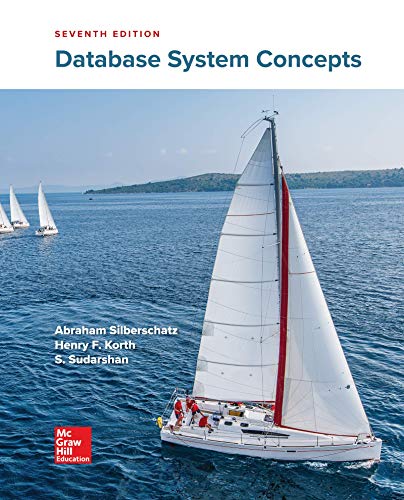
Concept explainers
PLEASE NOTE: IT'S A TEXT BOOK EXERCISE.
BOOK NAME: Fundamentals of Computer
Problem: Min Method (Return the Smaller Number)
Create a method GetMin(int a, int b), which returns the smaller of two numbers. Write a program, which takes as input three numbers and prints the smallest of them. Use the method GetMin(…), which you have already created.
Sample Input and Output
Input | Output | Input | Output |
---|---|---|---|
1 2 3 |
1 | -100 -101 -102 |
-102 |
Hints and Guidelines
Define a method GetMin(int a, int b) and implement it, after which invoke it from the main program as shown below. In order to find the minimum of three numbers, first, find the minimum of the first two and then the minimum of the result and the third number:
var min = GetMin(GetMin(num1, num2), num3);
Refences:
- Title: Programming Basics with C#
- Authors: Svetlin Nakov & Team
- ISBN: 978-619-00-0902-3 (9786190009023)
- Edition: Faber Publishing, Sofia, 2019

Step by stepSolved in 2 steps

- Student name: CIS 232 Introduction to Programming Homework Assignment 10 Due Date: 11/23/2020 Instructor: Dr. Lomako Problem Statement: Write a Java program with the main method and a multiplication method (must be created, not the library method). Create “HW10_lastname.java” program that: Prints a program title Creates two n x n square matrices A and B and initializes them with random values Displays the matrices A and B Calls the multiplication method that multiplies two square matrices and returns a matrix AB that is the result of the multiplication. Prints AB matrixarrow_forwardFinancial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forwardPython: numpy def serial_numbers(num_players):"""QUESTION 2- You are going to assign each player a serial number in the game.- In order to make the players feel that the game is very popular with a large player base,you don't want the serial numbers to be consecutive. - Instead, the serial numbers of the players must be equally spaced, starting from 1 and going all the way up to 100 (inclusive).- Given the number of players in the system, return a 1D numpy array of the serial numbers for the players.- THIS MUST BE DONE IN ONE LINEArgs:num_players (int)Returns:np.array>> serial_numbers(10)array([1. 12. 23. 34. 45. 56. 67. 78. 89. 100.])>> serial_numbers(12)array([1. 10. 19. 28. 37. 46. 55. 64. 73. 82. 91. 100.])""" # print(serial_numbers(10)) # print(serial_numbers(12))arrow_forward
- Java Calculating the User's Sum Write a complete program that reads in numbers and calculates the sum of the numbers in that range. Code Specifications In the main method, read input from the user. Read in two integers from the user: a lower end of the range and an upper end of the range. Check if the numbers are valid: the lower number cannot be greater than the upper number. If the numbers are invalid, use a loop to ask for new numbers. Continue looping until you get two valid values. Write a method called calculateTheSum. The method takes in a lower and upper end of the range. The method calculates the sum of all values from lower (inclusive) to upper (inclusive). Invoke the method from main the output the result. Test Cases I recommend testing your code using the test cases below. I've listed the user inputs along with a sample of the result. User Inputs Result lower = 10, upper = 1 the program should ask for new input lower = 5, upper = -5 the program should ask…arrow_forwardOn Thursday, November 4, 2021, Big Man Games wrote: Computer programs are great at computing mathematical formulas. Once the formula is properly encoded you can use the code as much as you want without reprogramming it and you can share it with non-programmers without any trouble. This lab is an example of such a formula. Once you program it you won’t have to worry about the area of a circle again. Write and test a program that computes the area of a circle. This program should request a number representing a radius as input from the user. Use the formula 3.14 × radius2 to compute the area. Tip: There are a couple of ways to code an exponent. Look in the Operators unit for help (and you can’t use an x for multiplication). Tip: You will need to use the float data type to compute the remainder. The output should explain the results. Don’t just print a number. Tip: For your print statement you will need to use the comma, “,”, or plus, “+” symbols to stitch your output together. (“The…arrow_forwardUsing introduction to Python You have been asked to write an application that automatically grades a quiz. The quiz has 15 multiple-choice questions. The correct answers are: 1.A, 2.C, 3.A, 4.B, 5.B, 6.D, 7.D, 8.A, 9.C, 10.A, 11.B, 12.C, 13.D, 14.C, 15.B The program will start by creating a list holding the quiz answers key shown above. It will then prompt the user for the student name and their answers file and read them into a separate list. It will finally compare the key vs. answers lists to calculate & display: Number of correct answersNumber of incorrect answersQuestions incorrectly answered.Whether the student has passed or failed the quizA student must correctly answer 11 out of 15 questions to pass.The user can grade as many students as they wish. Notes The file containing the student’s answers is organized as 15 lines. Each line contains the answer to the corresponding question. The responses are single character strings, from A to D, uppercase. Must use functional…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
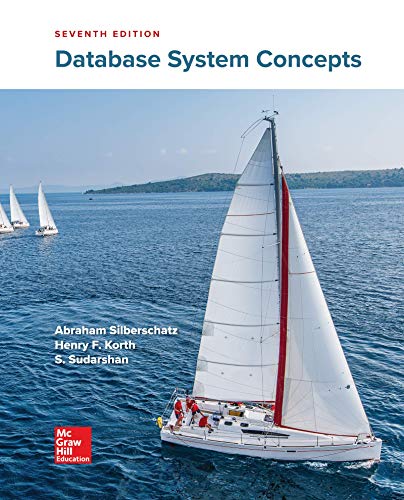
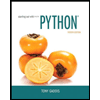
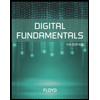
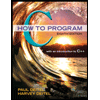
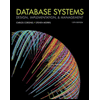
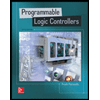