Create a login button on the index page and take the user to a login page. Prompt them for their username and password and compare it to the username and password stored for them in web storage. If it matches, display an alert that says "Logged In" If it does not match, display a message on the page that the username or password does not match and try again. At the bottom of the login form, add a reset password link. Take the user to a reset password page that prompts them for their username and password and changes the password in web storage. Existing Code: Index.html Titan Sports Store Titan Sports Store < > The Titans Sports Store serves as the forefront store to get merch to rep your favorite school. St. Peterburg College and their sports teams have a wide variety of merch to choose from and show your support with. Titan Sports and Apparel LLC Copyright © 2021 Subscribe to our newsletter Register here Register.js function validateData() { var outputTable = ""; var validInformation = true; var mailformat = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/; if (document.getElementById('email').value.match(mailformat)) { outputTable += "EMAIL " + document.getElementById('email').value + ""; } else { outputTable += "EMAIL Invalid email"; validInformation = false; } var regx = /^[6-9]\d{9}$/; if (regx.test(document.getElementById("phone").value)) { outputTable += "phonenumber " + document.getElementById('phone').value + ""; } else { outputTable += "phonenumber Invalid phone "; validInformation = false; } if (document.getElementById('address').value != "") { outputTable += "address " + document.getElementById('address').value + ""; } else { outputTable += "address Address cannot be empty"; validInformation = false; } if (document.getElementById('city').value != "") { outputTable += "city " + document.getElementById('city').value + ""; } else { outputTable += "address city cannot be empty"; validInformation = false; } if (/^\d{5}(-\d{4})?$/.test(document.getElementById('zip').value)) { outputTable += "zip " + document.getElementById('zip').value + ""; } else { outputTable += "zip Invalid zip"; validInformation = false; } if (document.getElementById('contact').checked == true) { outputTable += "contact" + document.getElementById('contact').value + ""; } else { outputTable += "contactNot set"; validInformation = false; } if (document.getElementById('terms').checked) { outputTable += "Terms and condtionAccepted"; } else { outputTable += "Terms and condtionNot Accepted"; validInformation = false; } outputTable += ""; if (validInformation) { document.getElementById('results').hidden = true; alert("The users account has been registered"); } else { document.getElementById('results').innerHTML = outputTable; } const User = { email: document.getElementById('email'), phone: document.getElementById('phone'), address: document.getElementById('address'), city: document.getElementById('city'), state: document.getElementById('state'), zip: document.getElementById('zip'), conatct: document.getElementById('contact').value, TandC: document.getElementById('terms').checked ? "Accepted" : "Not Accpeted" } storeData(User); } function storeData(User) { if (typeof(Storage) !== "undefined") { localStorage.setItem(User.email, User); } else { console.log("Sorry, your browser does not support Web Storage..."); } }
Create a login button on the index page and take the user to a login page. Prompt them for their username and password and compare it to the username and password stored for them in web storage. If it matches, display an alert that says "Logged In" If it does not match, display a message on the page that the username or password does not match and try again.
At the bottom of the login form, add a reset password link. Take the user to a reset password page that prompts them for their username and password and changes the password in web storage.
Existing Code:
Index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Titan Sports Store</title>
<link rel="stylesheet" href="./css/style.css">
</head>
<body>
<header>
Titan Sports Store
</header>
<div class="banner">
<div class="previous"><</div>
<div class="next">></div>
</div>
<main class="content">The Titans Sports Store serves as the forefront store to get merch to rep your favorite school. St. Peterburg College
and their sports teams have a wide variety of merch to choose from and show your support with.</main>
<footer>Titan Sports and Apparel LLC Copyright © 2021 <a href="subscribe.html">Subscribe to our newsletter</a> <a href="register.html">Register here</a></footer>
<script src="./js/app.js"></script>
</body>
</html>
Register.js
function validateData() {
var outputTable = "<table>";
var validInformation = true;
var mailformat = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/;
if (document.getElementById('email').value.match(mailformat)) {
outputTable += "<tr><td>EMAIL </td><td>" + document.getElementById('email').value + "</td></tr>";
} else {
outputTable += "<tr><td>EMAIL </td><td>Invalid email</td></tr>";
validInformation = false;
}
var regx = /^[6-9]\d{9}$/;
if (regx.test(document.getElementById("phone").value)) {
outputTable += "<tr><td>phonenumber </td><td>" + document.getElementById('phone').value + "</td></tr>";
} else {
outputTable += "<tr><td>phonenumber </td><td> Invalid phone </td></tr>";
validInformation = false;
}
if (document.getElementById('address').value != "") {
outputTable += "<tr><td>address </td><td>" + document.getElementById('address').value + "</td></tr>";
} else {
outputTable += "<tr><td>address </td><td>Address cannot be empty</td></tr>";
validInformation = false;
}
if (document.getElementById('city').value != "") {
outputTable += "<tr><td>city </td><td>" + document.getElementById('city').value + "</td></tr>";
} else {
outputTable += "<tr><td>address </td><td>city cannot be empty</td></tr>";
validInformation = false;
}
if (/^\d{5}(-\d{4})?$/.test(document.getElementById('zip').value)) {
outputTable += "<tr><td>zip </td><td>" + document.getElementById('zip').value + "</td></tr>";
} else {
outputTable += "<tr><td>zip </td><td> Invalid zip</td></tr>";
validInformation = false;
}
if (document.getElementById('contact').checked == true) {
outputTable += "<tr><td>contact</td><td>" + document.getElementById('contact').value + "</td></tr>";
} else {
outputTable += "<tr><td>contact</td><td>Not set</td></tr>";
validInformation = false;
}
if (document.getElementById('terms').checked) {
outputTable += "<tr><td>Terms and condtion</td><td>Accepted</td></tr>";
} else {
outputTable += "<tr><td>Terms and condtion</td><td>Not Accepted</td></tr>";
validInformation = false;
}
outputTable += "</table>";
if (validInformation) {
document.getElementById('results').hidden = true;
alert("The users account has been registered");
} else {
document.getElementById('results').innerHTML = outputTable;
}
const User = {
email: document.getElementById('email'),
phone: document.getElementById('phone'),
address: document.getElementById('address'),
city: document.getElementById('city'),
state: document.getElementById('state'),
zip: document.getElementById('zip'),
conatct: document.getElementById('contact').value,
TandC: document.getElementById('terms').checked ? "Accepted" : "Not Accpeted"
}
storeData(User);
}
function storeData(User) {
if (typeof(Storage) !== "undefined") {
localStorage.setItem(User.email, User);
} else {
console.log("Sorry, your browser does not support Web Storage...");
}
}

Step by step
Solved in 2 steps with 4 images

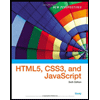
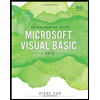
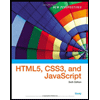
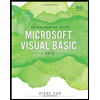