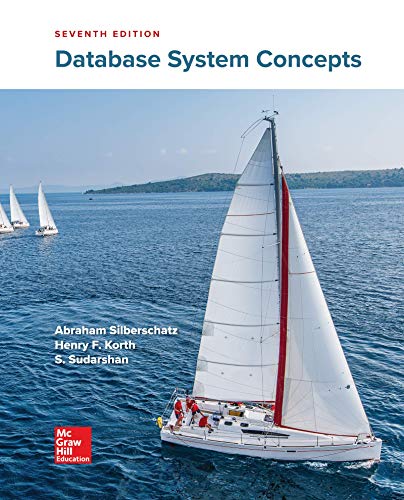
-
Create a Java class called "Person" with the following instance variables:
- a String variable named "name"
- an integer variable named "age"
-
Create a constructor for the Person class that takes in a String parameter "name" and an integer parameter "age" and initializes the instance variables with those values.
-
Create a method in the Person class called "introduce" that returns a String introducing the person, including their name and age.
-
In the main method, create a new instance of the Person class and pass in the values "John" and 30 for the name and age parameters, respectively. Then, call the "introduce" method on the new instance and output the result to the console.
Output: "Hi, my name is John and I am 30 years old."
-
Finally, create another instance of the Person class with a different name and age, and call the "introduce" method on that instance as well.
Output: "Hi, my name is Jane and I am 25 years old."

Step by stepSolved in 4 steps with 2 images

- 5. CODE IN PYTHON Create a BankAccount class which has an __init__ method which accepts an account name and starting balance to store as instance fields. The class should also have a method to deposit money, a method to withdraw money, and a method called "get_balance" which returns a string of the format "{account_name} has a balance of {balance}" (do not print this out from within the class, just return the string). In the same file, create an instance of the BankAccount class with a hardcoded name and starting balance. Deposit any amount to the account and withdraw any amount from the account (both can be hardcoded) and then print the result of calling the get_balance method on the instance.arrow_forwardJAVA. THIS IS A PROGRAMMING ASSIGNMENT NOT A WRITING ASSIGNMENT IF YOU ANSWERED BEFORE DO NOT ANSWER AGAIN PLEASE DO NOT PASTE A PAPER Design a class to store the following information about computer scientists⦁ Name⦁ Research area(s)⦁ Major contribution A scientist may have more than one area of research.⦁ Implement the usual setters and getters methods ⦁ Implement a method that takes a research area as an input and return thenames of scientists associated with that area ⦁ Implement a method that takes a word as an input and return the names ofscientists whose contribution mention that word Next, perform desktop research to find at least five prominent black computerscientists, with their research area(s) and major contribution, and store this data intoobjects of the class you designed. You can just hardcode this data. No user input needed.Define JUnit tests to check your research area and contribution search methods.arrow_forwardWrite a Java class named PhoneRecord.java that performs the following functions: The class should contain the following data attributes and methods: private String name - The customer's name private String number - The customer's phone number public PhoneRecord(String personName, String phoneNumber) - Constructor get/set Methods - For each data attribute public String toString( ) - Special method to be used when printing a phoneRecord object Thank you so much for your help. Trying to write this class has really been stumping me.arrow_forward
- Create a class Course, which has one field: String courseName Create the constructor, accessor, and mutator for the class. Then, in the main method of this class, create an instance of the class with the name "CST1201". Write an equivalent while statement to replace the following for statement for (int i=2; i<100; i=i+2) { System.out.println(i); }arrow_forward1. Create a properly encapsulated class named Person that has the following: A String instance variable called name. A constructor that takes a String parameter and sets the instance variable. A properly named getter for the instance variable. A correctly overrided equals method which returns true if the name instance variable of the parameter and the reference variable calling the method are equal. (Do not forget the null and class type checks!) Compile your Person class. 2. Create a properly encapsulated class named Employee that inherits from Person and has the following: ● ● ● A String instance variable called office (e.g. "LWH 2222"). A String instance variable called hourlywage (e.g. "$45.15"). A constructor that takes three Strings as parameters for name, office, and hourlyWage and sets the instance variables of both the parent class and the Employee class. An overridden toString method that returns the concatenation of name and hourlyWage instance variables (separated by a…arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forward
- A. Build a class named car. This class is defined as follows: It has the fields: Car ID, Car model, Car make, Car color, Car year. Build a constructor that accepts the five parameters (Car ID, Car model, Car make, Car color, Car year). Override the method toString() to return the string representation of Car ID, Car model, Car make, Car color, Car year. Build a method named display to print the values returned by toString() method. Override the equals() method to compare the Car model, Car make, Car color of two Car objects. The method returns true if all the values of these fields are the same, and false otherwise. In the equals method you must check the following: a. If you compare the Car object to itself, return true.! b. If you compare car object with another car object return true, otherwise return false. Hint use : instanceof operator for this purpose. Override the hashcode() method to return same hash codes for objects that are equal according to the equals() method you built…arrow_forwardIn a Java program, create an automobile class that will be used by a dealership as a vehicle inventory program. The following attributes should be present in your automobile class: private string make, private string model, private string color, private int year, private int mileage. Your program should have appropriate methods such as: constructor, add a new vehicle, remove a vehicle, update vehicle attributes. At the end of your program, it should allow the user to output all vehicle inventory to a text file.arrow_forwardOverriding is useful when a method has the same signature and type as a method in the parent class. O a local variable has the same name as an instance variable. O you want to change the return type of a method. O a method has the same signature as another method in the same class.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
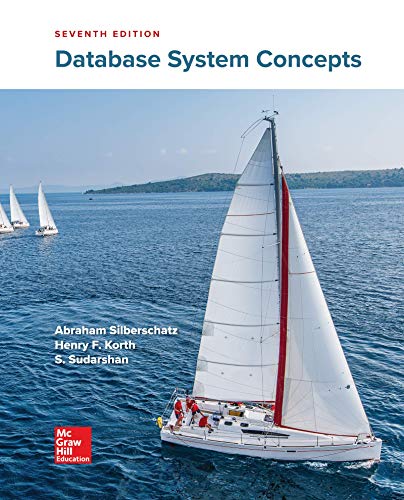
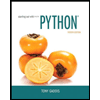
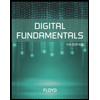
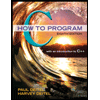
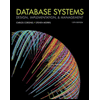
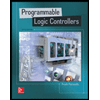