Create a function called MilitaryToRegularTime that converts time in the military time format into the regular format. For example, convert 2249 to 10:49 pm. The function should receive a single int parameter that represents the military time. It should return a std::string that contains the regular time counterpart of the given military time. Please see the sample output below to guide the design of your program
Military to Regular Time
Create a function called MilitaryToRegularTime that converts time in the military time format into the regular format. For example, convert 2249 to 10:49 pm.
The function should receive a single int parameter that represents the military time. It should return a std::string that contains the regular time counterpart of the given military time.
Please see the sample output below to guide the design of your program.
Note: Consider possible edge cases in user input to ensure your program works correctly.
Sample output:
Please enter the time in military time: 1433 The equivalent regular time is: 2:33 pm
Make sure that you examine the MilitaryToRegularTime function prototype in time_converter.h, implement it in time_converter.cc, and call it from inside of main.cc. You'll find that skeleton code has already been provided and you simply need to call the function, which can be called from inside main.cc because we include the header file via: #include "time_converter.h"
main.cc file
#include <iostream> | |
#include <string> | |
#include "time_converter.h" | |
int main() { | |
int military_time = 0; | |
std::cout << "Please enter the time in military time: "; | |
std::cin >> military_time; | |
// TODO: Call your function to convert from military time to regular time | |
// and assign its result to regular_time. | |
std::string regular_time; | |
std::cout << "The equivalent regular time is: " << regular_time << "\n"; | |
return0; | |
} |
time_converter.cc file
#include <iostream> | |
#include <string> | |
std::string MilitaryToRegularTime(int military_time) { | |
// TODO: convert military_time to regular time in string format. | |
// Hint: std::to_string() converts a given integer to a string. | |
return""; | |
} |
time_converter.h file
#include <iostream> | |
#include <string> | |
// Converts the time in military format to regular format. | |
std::string MilitaryToRegularTime(int military_time); |



main.cc file
#include <iostream>
#include <string>
#include "time_converter.h"
int main() {
int military_time = 0;
std::cout << "Please enter the time in military time: ";
std::cin >> military_time;
// TODO: Call your function to convert from military time to regular time
// and assign its result to regular_time.
std::string regular_time;
regular_time = MilitaryToRegularTime(military_time);
std::cout << "The equivalent regular time is: " << regular_time << "\n";
return0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

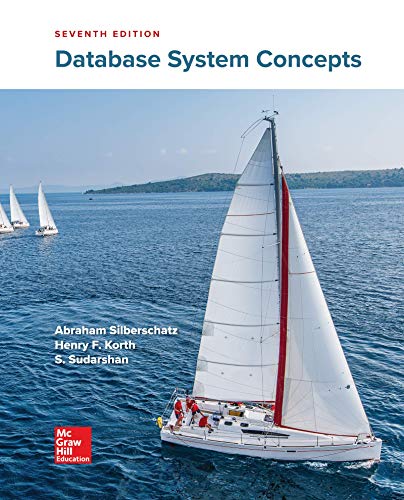
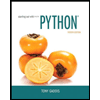
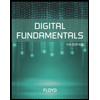
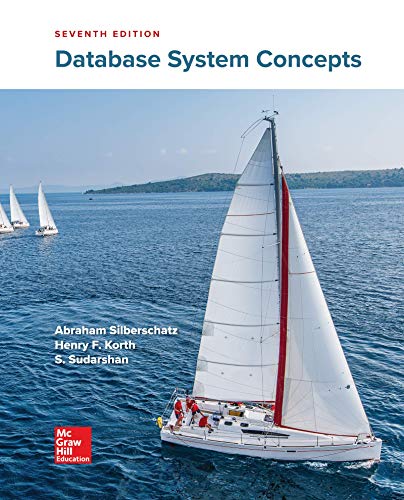
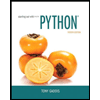
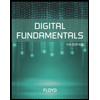
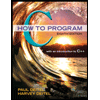
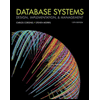
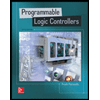