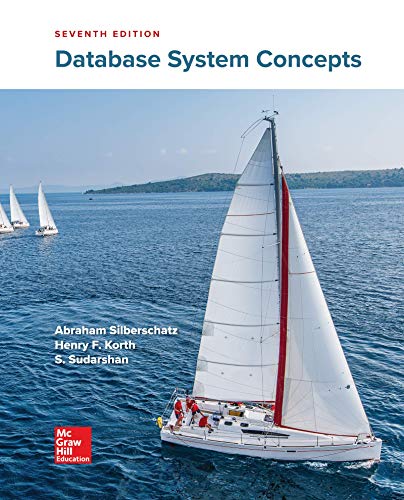
Create a class called Car (Car.java). It should have the following private data members:
String make
String mode
lint year
Provide the following methods:
default constructor (set make and model to an empty string, and set year to 0)
non-default constructor Car(String make, String model, int year)
getters and setters for the three data members
method print() prints the Car object’s information, formatted as follows:
Make: Toyota
Model: 4Runner
Year: 2010
public class Car {
}
Complete the following unit test for the class Car described above. Make sure to test each public constructor and each public method, print the expected and actual results.
// Start of the unit test of class Car
public class CarTester {
public static void main(){
// Your source codes are placed here
return;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- 1. RetailItem Class Write a class named Retailltem that holds data about an item in a retail store. The class should have the following fields: • description. It is a String object that holds a brief description of the item. • unitsOnHand. It is an int variable that holds the number of units currently in inventory. • price. It is a double that holds the item's retail price. Write appropriate mutator methods that store values in these fields and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetailItem objects and stores the following data in them. Units On Hold Description Designer Jeans Jacket Price 40 34.95 12 59.95 Shirt 20 24.95arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forwardQ3: Define a class named Car that has the following specifications:Two attributes:plateNumber: to keep car’s plate number as String value. speed: to keep car’s current speed as integer value.Seven methods:Car( String pn , int s), a constructor method used to initialize the instantiated objects. setPlateNumber( String pn), act as a set method for the attribute plateNumber. setSpeed( int s), act as the set method for the attribute speed.getPlateNumber(), act as the get method for the attribute plateNumber.getSpeed(), act as the get method for the attribute speed.fines (), returns the amount of traffic fine (500 SR) if car’s speed exceeds 120 k/h, otherwise 0. main(...), act as the main method for the program, and it should do the following:1. Instantiate three objects, namely car1, car2, and car3, using the populated constructor.2. Change the speed value of the first car (car1) to 130.3. Change the plate number of the second car (car2) to “Java123”.4. Print the following statement,The…arrow_forward
- Car Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable…arrow_forward1- Design a class named Rectangle to represent a Rectangle. The class contains: • three variables integer data field named height, integer data filed named width and integer data filed named length. The default values are 1 for height, length, and width are 1.0 for each of them respectively. • A no-arg constructor that creates a default Rectangle. • A constructor that creates a Rectangle with the specified height, length and width. • A method named getVolume() that returns the volume of this Rectangle. (Volume = height * width * length) %3! 2- Write a test program called Test Rectangle that: • Creates two Rectangle objects: one object with height 11, length 5, and width 7, the other object with height 10, length 2 and width 6 • Display the volume of each Rectangle object.arrow_forwardJAVA Programming Problem 3 - Book A Book has such properties as title, author, and numberOfPages. A Volume will have properties such as volumeName, numberOfBooks, and an array of book objects (Book [ ]). You are required to develop the Book and Volume classes, then write an application (DemoVolume) to test your classes. The directions below will give assistance. Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages, Create a second class called Volume with the following properties using appropriate data types: volumeName, numberOfBooks and Book [ ]. The Book [ ] contains an array of book objects. Directions Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages, The only methods necessary in the Book class, for this exercise, are the constructor and a toString(). Create a second class called Volume with the following properties using appropriate data types:…arrow_forward
- this is my retail item code: //Import statements public class RetailItem { private String description; private int units; private double price; public RetailItem() { } public RetailItem(String x, int y, double z) { description = x; units = y; price = z; } public RetailItem(RetailItem i) { } public void setDescription(String x) { description = x; } public void setPrice(double z) { price = z; } void setUnits(int y) { units = y; } public int getUnits() { return units; } public String getDescription() { return description; } public double getPrice() { return price; } // Main class public static void main(String[] args) { String str = "Shirt"; RetailItem r1 = new RetailItem("Jacket", 12,…arrow_forwardPersonal Information Class: Design a class called Employee that holds the following data about an employee: name ID number Department Job Title Class. Store your class in a separate file called employee.py. Your class will have an initializer method that will be passed the information entered by the user as arguments. Write appropriate accessor and mutator methods for each data attribute. Write a __str__ method to print the contents of the class (see example of __str__ on p. 523). Main program: Your main program should create three instances of the class. Your program should get the information from the user and pass it as parameters to the initializer method. Using the __str__ method invoked by the print function, the program should display the personal information for the three individuals. Output and Sample Dialog: Enter employee name: Mary Smith Enter employee ID: 123456 Enter department: Accounting Enter position: Accountant Enter employee name: Joe Morales Enter…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
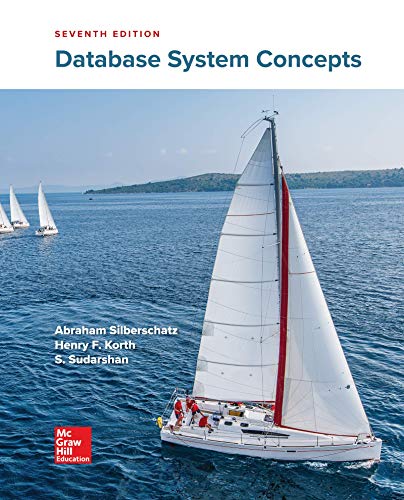
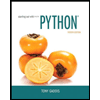
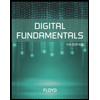
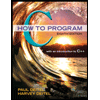
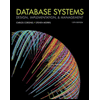
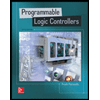