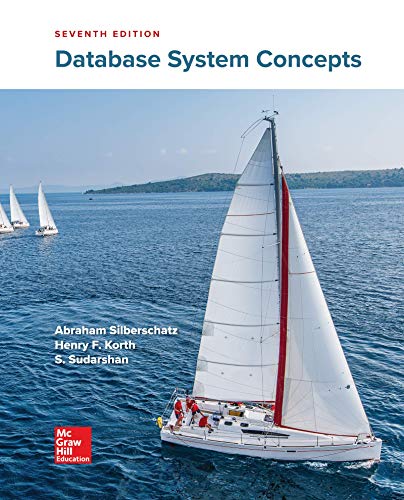
CPSC 131: Introduction to Computer Programming II
Program 3: Inheritance and Interface
1 Description of the Program
In this assignment, you will make two classes, Student and Instructor, that inherit from a
superclass Person. The implementation of class Person is given. You will also need to write
a test program to test the methods you write for these two classes. The implementation
details are described as follows.
Stage 1: In the first file Student.java, you should include the following additional instance
variables and methods (other than all instance variables and methods inherited from class
Person):
• Private instance variables studentID, and major;
• A constructor takes four inputs (name, age, studentID and major);
• Two additional getter methods to return each of instance variables (accessor);
• Two setter methods to change each of instance variables (mutator);
• A method toString that converts a student’s information into string form. The
string should have the format as shown in Figure 1. You should override superclass
toString() method.
• A method compareTo that implements the interface Comparable, so that Student
objects can be sorted by studentID in an ascending order.
Stage 2: In the second file Instructor.java, you should include the following additional
instance variables and methods (other than all instance variables and methods inherited
from class Person):
• Private instance variable salary;
• A constructor takes three inputs (name, age, and salary);
• One additional getter method to return the instance variable (accessor);
• One setter method to change the instance variable (mutator);
1
• A method toString that converts an instructor’s information into string form. The
string should have the format as shown in Figure 1. Specifically, you need to format
salary value to 2 decimal places, and make them right aligned. You should also
override superclass toString() method.
• A method compareTo that implements the interface Comparable, so that Instructor
objects can be sorted by salary in an ascending order.
Stage 3: In the third file PersonTester.java, you will need to do the followings:
1. You need to read data file “data1.txt” into an array of Student objects, Specifically,
(a) The first number in the first line of the file is used to determine the array size.
(b) The remaining lines are the student records. You will need to create a Student
object using each line’s information, and put it into the array.
2. Do sorting of the initialized array of Student objects, and then print them out. The
outputs should be nicely labeled and formatted, as shown in Figure 1.
3. Repeat the above steps to read data file “data2.txt” into an array of Instructor
objects, do sorting and print them out. The outputs should be nicely labeled and
formatted, as shown in Figure 1. To avoid code redundancy, you may use a loop to
handle the repetitive procedure.
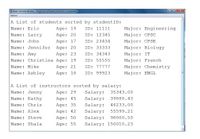

Trending nowThis is a popular solution!
Step by stepSolved in 7 steps with 5 images

- create a program for these to questions using javaarrow_forwardPython:Please read the following. The Game class The Game class brings together an instance of the GameBoard class, a list of Player objects who are playing the game, and an integer variable “n” indicating how many tokens must be aligned to win (as in the name “Connect N”). The game class has a constructor and two additional methods. The most complicated of these, play, is provided for you and should not be modified. However, you must implement the constructor and the add_player method. __init__(self,n,height,width) The Game constructor needs to initialize three instance variables. The first, self.n, simply holds the integer value “n” provided as a parameter. The second, self.board, should be a new instance of the GameBoard class (described below), built using the provided height and width as dimensions. The third, self.players, should be an initially empty list. add_player(self,name,symbol) The add_player method is provided a name string and a symbol string. These two values should be…arrow_forwardIn this java assignment, we will be creating a pay stub for an employee using classes. Each class must be implemented into their own file, a total of 5 classes/files. We are going to implement the following classes: Employee – This class represents an employee. It needs the following fields exposed via getters and setters: Employee ID (we will need to make this unique, for now, just hard code it to 1). First name, Last name, Middle initial Address, City, Zip Phone, Email Hourly Rate PayPeriod – This class represents an employee’s payment information. An employee will eventually have more than one pay period. It must contain the following fields (exposed via getters and setters): Pay period Id (hard code it for now) Employee Id (who is this for) Start Date, End Date Number of hours PayrollManager – This class provides the functionality we need to compute and display our payroll. It should implement the following methods: double CalculateGrossPay (Employee, Payperiod) – This…arrow_forward
- Write a C#program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string) ClassRegistration -…arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward6. Design a Java class with a main method that does the following. You must include a meaningful comment for main and each method a. Main invokes a method readScores to read input from a file (name of your choice) that contains the name of the bowlers (no more than 25) and their bowling scores (two scores per bowler). The program stops reading values when it has reached the end of the file. For example, the file might contain: Bob 203 176 Jane 210 253 Jack 300 189 Eileen 220 298 • As the method reads the data, it stores the name into a String array and the average of the two bowling scores into an array of double. • The method returns the actual number of bowlers read in b. Main then invokes a method computeOverallAvg to: compute (a) the overall average score of all the bowlers combined, (b) the average score and name of the bowler with the highest average score and (c) the average score and name of the bowler with the lowest average scores. print to the console (a) the overall average…arrow_forward
- Written in Python with docstring please if applicable Thank youarrow_forwardProgram - Python Make a class.The most important thing is to write a simple class, create TWO different instances, and then be able to do something. For example, create a pizza instance for Suzie, ask her for the toppings from the choices, and store her pizza order. Then create a second pizza instance for Larry, ask for his toppings and store his pizza order. Then print both of their orders back out. You do not need to make your class very complicated. (Shouldn't be the pizza example but something similar)arrow_forwardJava- Suppose that Vehicle is a class and Car is a new class that extends Vehicle. Write a description of which kind of assignments are permitted between Car and Vehicle variables.arrow_forward
- !! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forwardPLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forwardI already have the code for the assignment below, but the code has an error in the driver class. Please help me fix it. The assignment: Make a recursive method for factoring an integer n. First, find a factor f, then recursively factor n / f. This assignment needs a resource class and a driver class. The resource class and the driver class need to be in two separate files. The resource class will contain all of the methods and the driver class only needs to call the methods. The driver class needs to have only 5 lines of code The code of the resource class: import java.util.ArrayList;import java.util.List; public class U10E03R{ // Recursive function to // print factors of a number public static void factors(int n, int i) { // Checking if the number is less than N if (i <= n) { if (n % i == 0) { System.out.print(i + " "); } // Calling the function recursively // for the next number factors(n, i +…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
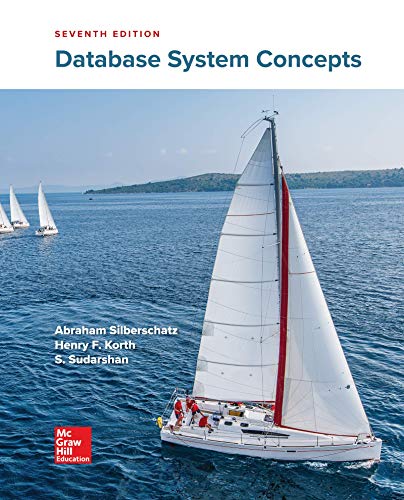
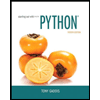
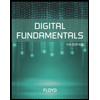
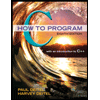
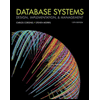
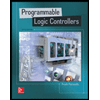