count_outgoing_Tlights(): """Return the number of outgoing flights for the airport with the iata the given routes information. >>>count_outgoing_flights('AA1', TEST_ROUTES_DICT_FOUR_CITIES) >>>count_outgoing_flights ('AA2', TEST_ROUTES_DICT_FOUR_CITIES) 2 1 return len(routes [iata]) # Complete this function (including type contract and docstring) # based on the handout description pass def count_incoming_flights(): """Return the number of incoming flights for the airport with the iata the given routes information. >>>count_incoming_flights ('AA1', TEST_ROUTES_DICT_FOUR_CITIES) >>>count_incoming_flights ('AA2', TEST_ROUTES_DICT_FOUR_CITIES) 2 1 num=0 for i in routes: if iata in routes[i]: num+=1 return num # Complete this function (including type contract and docstring) # based on the handout description
def reachable_destinations(iata_src: str, n: int, routes: RouteDict) -> List[Set[str]]:
"""Return a list of the sets of airports where the set at index i is
reachable in at most i flights. Note that iata_src will always appear at
index 0, because it is reachable without flying anywhere.
Precondition: n >= 0
>>> reachable_destinations('AA1', 0, TEST_ROUTES_DICT_FOUR_CITIES)
[{'AA1'}]
>>> expected = [{'AA1'}, {'AA2', 'AA4'}]
>>> result = reachable_destinations('AA1', 1, TEST_ROUTES_DICT_FOUR_CITIES)
>>> expected == result
True
>>> expected = [{'AA1'}, {'AA2', 'AA4'}, {'AA3'}]
>>> result = reachable_destinations('AA1', 2, TEST_ROUTES_DICT_FOUR_CITIES)
>>> expected == result
True
"""
# Complete your function below
![def count_outgoing_flights():
"""Return the number of outgoing flights for the airport with the iata in
the given routes information.
>>>count_outgoing_flights
('AA1',
TEST_ROUTES_DICT_FOUR_CITIES)
>>>count_outgoing_flights
('AA2',
TEST_ROUTES_DICT_FOUR_CITIES)
2
1
||||||
return len (routes [iata])
# Complete this function (including type contract and docstring)
#based on the handout description
pass
def count_incoming_flights():
"""Return the number of incoming flights for the airport with the iata in
the given routes information.
>>>count_incoming_flights ('AA1', TEST_ROUTES_DICT_FOUR_CITIES)
>>>count_incoming_flights ('AA2', TEST_ROUTES_DICT_FOUR_CITIES)
2
1
num=0
for i in routes:
if iata in routes [i]:
num+=1
return num
# Complete this function (including type contract and docstring)
#based on the handout description
pass](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F99722726-db42-4938-b031-e662dbbc7dd1%2F5ef44efc-34d9-49a7-ba0f-68ee8aa81249%2F86rewx_processed.png&w=3840&q=75)
![def count_total_flights():
# Complete this function (including type contract and docstring)
#based on the handout description
pass
def reachable_destinations (iata_src: str, n: int, routes: RouteDict) -> List [Set
"""Return a list of the sets of airports where the set at index i is
reachable in at most i flights. Note that iata_src will always appear at
index 0, because it is reachable without flying anywhere.
Precondition: n >= 0
>>> reachable_destinations ('AA1', 0, TEST_ROUTES_DICT_FOUR_CITIES)
[{'AA1'}]
>>> expected = [{'AA1'}, {'AA2', 'AA4'}]
>>> result = reachable_destinations ('AA1', 1, TEST_ROUTES_DICT_FOUR_CITIES)
>>> expected == result
True
>>> expected = [{'AA1'}, {'AA2', 'AA4'}, {'AA3'}]
>>> result = reachable_destinations ('AA1', 2, TEST_ROUTES_DICT_FOUR_CITIES)
>>> expected == result
True
||||||
# Complete your function below](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F99722726-db42-4938-b031-e662dbbc7dd1%2F5ef44efc-34d9-49a7-ba0f-68ee8aa81249%2Fwwzw18a_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

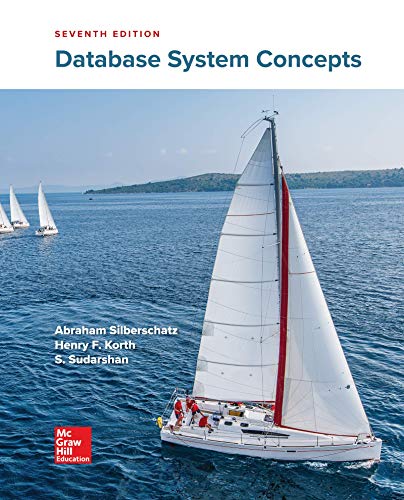
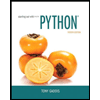
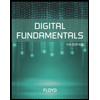
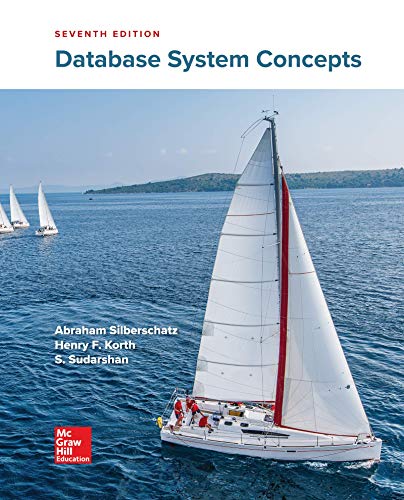
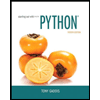
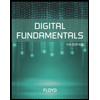
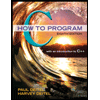
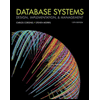
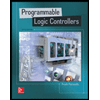