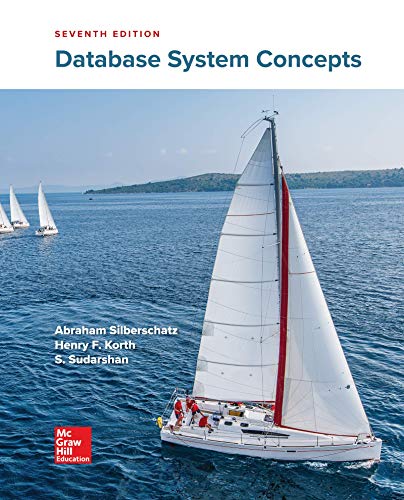
correct the syntax errors
using System;
class GFG {
void KMPSearch(string pat, string txt)
{
int M = pat.Length;
int N = txt.Length;
int[] lps = new int[M];
int j = 0;
int i = 0;
while (i < N) {
if (pat[j] == txt[i]) {
j++;
i++;
}
if (j == M) {
Console.Write("Found pattern "
j = lps[j - 1];
}
else if (i < N && pat[j] != txt[i]) {
if (j != 0)
j = lps[j - 1];
else
i = i + 1;
}
}
}
void computeLPSArray(string pat, int M, int[] lps)
{
int len = 0;
int i = 1;
lps[0] = 0;
while (i < M) {
if (pat[i] == pat[len]) {
len++;
lps[i] = len;
i++;
}
else
{
if (len != 0) {
len = lps[len - 1];
}
else
{
lps[i] = len;
i++;
}
}
}
}
public static void Main()
{
string txt = "ABABDABACDABABCABAB";
new GFG().KMPSearch(pat, txt);
}
}
![main.cpp:4:17: error: 'string' has not been declared
4 | void KMPSearch(string pat, string txt)
main.cpp:4:29: error: string' has not been declared
4 | void KMPSearch(string pat, string txt)
Aununun
main.cpp:31:25: error: string' has not been declared
31 |
void computelPSArray(string pat, int M, int[] lps)
main.cpp:31:50: error: expected
or .
before lps'
31 |
void computeLlPSArray(string pat, int M, int[] lps)
main.cpp:55:8: error: expected :' before 'static'
55 | public static void Main()
main.cpp:61:2: error: expected ;' after class definition
61 | }
|
main.cpp: In member function 'void GFG::KMPSearch(int, int)':
main.cpp:6:15: error: request for member Length' in 'pat', which is of non-class type 'int'
6 |
int M = pat.Length;
main.cpp:7:15: error: request for member Length' in txt’, which is of non-class type 'int'
int N = txt.Length;
main.cpp:8:6: warning: structured bindings only available with -std3c++17' or (-std=gnu++17'
8 |
int[] lps
new int[M];](https://content.bartleby.com/qna-images/question/1a2ba6b3-393c-46f7-b189-5deedb6a552a/61b3dd88-5bf4-4dce-8011-ce7cedd53e8e/pqwwpq_thumbnail.jpeg)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

- #include <stdio.h> struct Single { int num; }; void printSingle(int f) { int binaryNum[33]; int i = 0; while(f>0) { binaryNum[i] = f % 2; f = f/2; i++; } for (int j=i-1; j>= 0; j--) { printf("%d",binaryNum[j]); } } int main() { struct Single single; single.num = 33; printf("Number: %d\n",single.num); printSingle(single.num); return 0; }arrow_forwardpublic class arrayOutput { public static void main (String [] args) { final int NUM_ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; int sumVal; userVals [0] = 3; userVals [1] = 5; userVals [2] = 8; Type the program's output sumVal = 0; for (i = 0; i < userVals.length; ++i) { sumVal= sumVal + userVals [i]; System.out.println (sumVal); } ? ? ??arrow_forward#include <stdio.h> struct Single { int num; }; void printSingle(int f) { int binaryNum[33]; int i = 0; while(f>0) { binaryNum[i] = f % 2; f = f/2; i++; } for (int j=i-1; j>= 0; j--) { printf("%d",binaryNum[j]); } } int main() { struct Single single; single.num = 33; printf("Number: %d\n",single.num); printSingle(single.num); return 0; }arrow_forward
- using System; class main { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { int i; Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo[i]); } } } } This code is supposed to count from 1-88 and then select three random numbers from the list but instead it generates an infinite loop of random numers between 1-88. how can it be fixed?arrow_forwardQ1 #include <stdio.h> int arrC[10] = {0}; int bSearch(int arr[], int l, int h, int key); int *joinArray(int arrA[], int arrB[]) { int j = 0; if ((arrB[0] + arrB[4]) % 5 == 0) { arrB[0] = 0; arrB[4] = 0; } for (int i = 0; i < 5; i++) { arrC[j++] = arrA[i]; if (arrB[i] == 0 || (bSearch(arrA, 0, 5, arrB[i]) != -1)) { continue; } else arrC[j++] = arrB[i]; } for (int i = 0; i < j; i++) { int temp; for (int k = i + 1; k < j; k++) { if (arrC[i] > arrC[k]) { temp = arrC[i]; arrC[i] = arrC[k]; arrC[k] = temp; } } } for (int i = 0; i < j; i++) { printf("%d ", arrC[i]); } return arrC; } int bSearch(int arr[], int l, int h, int key) { if (h >= l) { int mid = l + (h - l) / 2; if…arrow_forwardint funcB(int); int funcA(int n) { if (n 4) { return n* funcA(n - 5); } else { return n- funcB(n - 1); int main() { cout << funcA(13); return 0; What is the output of this program? Please show our work.arrow_forward
- Question 37 public static void main(String[] args) { Dog[] dogs = { new Dog(), new Dog()}; for(int i = 0; i >>"+decision()); } class Counter { private static int count; public static void inc() { count++;} public static int getCount() {return count;} } class Dog extends Counter{ public Dog(){} public void wo(){inc();} } class Cat extends Counter{ public Cat(){} public void me(){inc();} } The Correct answer: Nothing is output O 2 woofs and 5 mews O 2 woofs and 3 mews O 5 woofs and 5 mews Oarrow_forward#include <stdio.h> struct Single { int num; }; void printSingle(int f) { int binaryNum[33]; int i = 0; while(f>0) { binaryNum[i] = f % 2; f = f/2; i++; } for (int j=i-1; j>= 0; j--) { printf("%d",binaryNum[j]); } } int main() { struct Single single; single.num = 33; printf("Number: %d\n",single.num); printSingle(single.num); return 0; }arrow_forwardIn the C programming language, if all function prototypes are listed at the top of your code, outside and above all functions, you do not need to worry about the order of the function definitions within the code. True Falsearrow_forward
- Fix the errors and send the code please // Application allows user to enter a series of words // and displays them in reverse order import java.util.*; public class DebugEight4 { public static void main(String[] args) { Scanner input = new Scanner(System.in); int x = 0, y; String array[] = new String[100]; String entry; final String STOP = XXX; StringBuffer message = new StringBuffer("The words in reverse order are\n"); System.out.println("Enter any word\n" + "Enter + STOP + when you want to stop"); entry = input.next(); while(!(entry.equals(STOP))) { array[x] = entry; ++x; System.out.println("Enter another word\n" + "Enter " + STOP + " when you want to stop"); entry = input.next(); } for(y = x - 1; y > 0; ++y) { message.append(array[y]); message.append("\n"); } System.out.println(message) }arrow_forward#include <stdio.h> int arrC[10] = {0}; int bSearch(int arr[], int l, int h, int key); int *joinArray(int arrA[], int arrB[]) { int j = 0; if ((arrB[0] + arrB[4]) % 5 == 0) { arrB[0] = 0; arrB[4] = 0; } for (int i = 0; i < 5; i++) { arrC[j++] = arrA[i]; if (arrB[i] == 0 || (bSearch(arrA, 0, 5, arrB[i]) != -1)) { continue; } else arrC[j++] = arrB[i]; } for (int i = 0; i < j; i++) { int temp; for (int k = i + 1; k < j; k++) { if (arrC[i] > arrC[k]) { temp = arrC[i]; arrC[i] = arrC[k]; arrC[k] = temp; } } } for (int i = 0; i < j; i++) { printf("%d ", arrC[i]); } return arrC; } int bSearch(int arr[], int l, int h, int key) { if (h >= l) { int mid = l + (h - l) / 2; if…arrow_forwardvoid fun(int i) { do { if (i % 2 != 0) cout =1); cout << endl; } int main() { int i = 1; while (i <= 8) { fun(i); it; } cout <arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
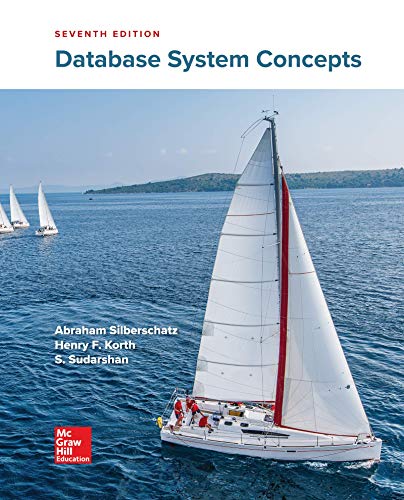
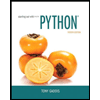
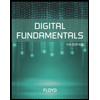
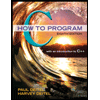
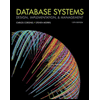
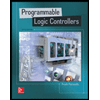