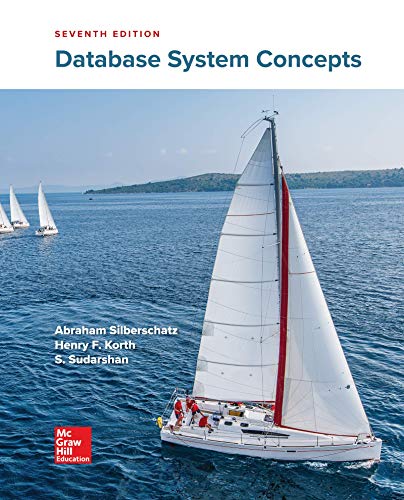
- Consider the following
program specification:
Write a program that will prompt the user for 10 integer values and stores them in an array. The values entered by the user only be between 0 and 100 and the program should validate these appropriately. The program should then:
- Display the average of the values
- Display the highest value in the array
- Display the lowest value in the array
- Discuss how the values should be validated. What control structures could be used?

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Consider the following
program specification:
Write a program that will prompt the user for 10 integer values and stores them in an array. The values entered by the user only be between 0 and 100 and the program should validate these appropriately. The program should then:
- Display the average of the values
- Display the highest value in the array
- Display the lowest value in the array
- Discuss how the values should be validated. What control structures could be used? Using eclipse
- Consider the following
program specification:
Write a program that will prompt the user for 10 integer values and stores them in an array. The values entered by the user only be between 0 and 100 and the program should validate these appropriately. The program should then:
- Display the average of the values
- Display the highest value in the array
- Display the lowest value in the array
- Discuss how the values should be validated. What control structures could be used? Using eclipse
- Write C code for a program that does the following: Create a character array that displays the message "My Friends Ages Program" at the declaration Create a second array that will store 4 integer values Use assignment statements to store the following friend's ages ages[0] = 25 ages[1] = 27 ages[2] = 24 ages[3] = 26 Use a puts() statement to display the message in the first bullet Use a for() statement to display each age stored in the array element The end output statement should display the friend's ages.arrow_forwardThe logic will allow the user to: Load a single dimensional array of size 50 with a random number The random number will range from 1 to 1,000 (you may have duplicate values) Find the highest value and the index location that it was in Find the smallest value and the index location that it was in Display the array’s contents. Display the highest value and its index location Display the lowest value and its index location Allow the user to execute this application multiple times (some sort of loop?) You will need one for loop to load the array as well as one or two for loops to search that array. The rand also has a “nasty” tendency to create the same results repeatedly in an exe. To avoid that, we have to “shuffle the deck” every time. This ensures that all numbers are an equal probability of appearing and not the same set of values (would create a boring game). // Add this to "shuffle the deck" every time to ensure that // different values could occur else the exe produces the…arrow_forwardJAVA based programarrow_forward
- Calculate average test score: Write a program that reads 10 test scores in an array named testScores. Then pass this array to a function to calculate the average test scores, return the average to main function and display it. Input Validation: The test scores should be between 0 and 100 (inclusive). Add comments and version control.arrow_forwardIn visual basic Create an array that will hold 10 integer values.arrow_forwardWrite a flowchart and C code for a program that does the following: Declare an array that will store 9 values Use a for() statement to store user entered scores for all 9- holes Create a function and pass the array into the definition of the function to compute the total Use an accumulating total statement to compute the total score Display the overall total to the output screen so that the golfer can see if he won.arrow_forward
- Problem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forwardWrite the pseudocode statement that will make a variable testAverage equal to the average of the first 3 scores in an array testScores.arrow_forwardIn visual basic Declare a two-dimensional array that has 3 columns and 4 rows.arrow_forward
- Visual basic 6.0arrow_forwardC++ Visual Studio 2019 Complete #7 Customer Accounts and #8 Search Function for Customer Accounts Program. Create the array with only 2 elements. 7.Customer Accounts Write a program that uses a structure to store the following data about a customer account: Name Address City, State, and ZIP Telephone Number Account Balance Date of Last Payment The program should use an array of at least 10 structures. It should let the user enter data into the array, change the contents of any element, and display all the data stored in the array. The program should have a menu-driven user interface. Input Validation: When the data for a new account is entered, be sure the user enters data for all the fields. No negative account balances should be entered. 8. Search Function for Customer Accounts Program Add a function to Programming Challenge 7 (Customer Accounts) that allows the user to search the structure array for a particular customer's account. It should accept part of the customer's name as an…arrow_forwardThere are 2 arrays, the first contains item numbers in inventory and the second parallel array contains the price of the items. The user will enter the item number they want to purchase, the program checks to see if it is a valid entry. If it is valid, it will tell the user the price of the item. If it is an invalid item, a message will display telling the user they entered an invalid item. Type up the code below, execute the program a few times.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
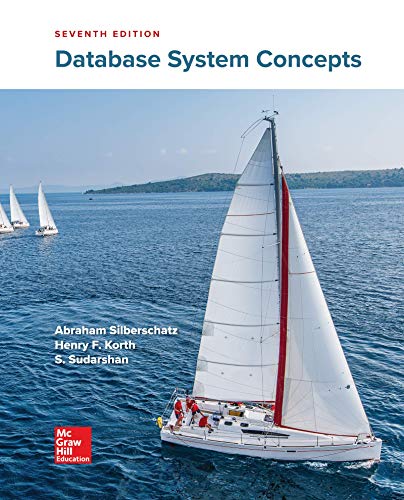
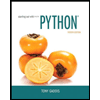
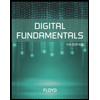
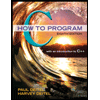
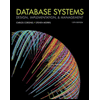
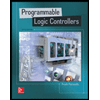