Complete this program to read in each of the four scores as float values, multiply each score by its corresponding weight, and then sum up the weighted scores to get the participant's final score as a float value.
Complete this program to read in each of the four scores as float values, multiply each score by its corresponding weight, and then sum up the weighted scores to get the participant's final score as a float value.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![This program tabulates scores for a figure skating competition that has four parts:
1. The short dance, which is worth 25% of the final score
2. The free skate, which is worth 50% of the final score
3. The partner dance, which is worth 10% of the final score
4. The original performance, which is worth 15% of the final score
The array `weights` stores values that represent how much of the final score each of the four parts is worth.
Complete this program to read in each of the four scores as `float` values, multiply each score by its corresponding weight, and then sum up the weighted scores to get the participant’s final score as a `float` value.
```cpp
#include <iostream>
#include <vector>
using namespace std;
const int WEIGHT_SIZE = 4;
int main() {
float weights[WEIGHT_SIZE] = {0.25, 0.50, 0.10, 0.15};
float total = 0.0;
// TODO: Write your code here
cout << "Final Score: " << total << endl;
}
```](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3ded9074-643d-40d8-825c-f0dff7af7ebf%2F0d01907f-a7b9-40ff-b85a-3631bc0a73fa%2Faogjyah_processed.jpeg&w=3840&q=75)
Transcribed Image Text:This program tabulates scores for a figure skating competition that has four parts:
1. The short dance, which is worth 25% of the final score
2. The free skate, which is worth 50% of the final score
3. The partner dance, which is worth 10% of the final score
4. The original performance, which is worth 15% of the final score
The array `weights` stores values that represent how much of the final score each of the four parts is worth.
Complete this program to read in each of the four scores as `float` values, multiply each score by its corresponding weight, and then sum up the weighted scores to get the participant’s final score as a `float` value.
```cpp
#include <iostream>
#include <vector>
using namespace std;
const int WEIGHT_SIZE = 4;
int main() {
float weights[WEIGHT_SIZE] = {0.25, 0.50, 0.10, 0.15};
float total = 0.0;
// TODO: Write your code here
cout << "Final Score: " << total << endl;
}
```

Transcribed Image Text:# Understanding the Mode of a Dataset
## Definition
The **mode** of a dataset is the item that appears most frequently.
## Instructions
You are required to write a program to read in numbers between 1 and 100 and then report the mode. If more than one number appears most frequently, choose the highest number. The program's output should be in the following format:
```
The most frequent value was 42, appearing 9 times.
```
## Sample Code
Below is a snippet of C++ code with the basic structure to find the mode of the entered numbers.
```cpp
#include <iostream>
using namespace std;
int main() {
// TODO: Write your code here
cout << "The most frequent value was " << 50 << ", appearing " << 8 << " times." << endl;
}
```
### Explanation
- **Include Directives**: `#include <iostream>` is used to include the standard input-output stream library.
- **Namespace**: The `using namespace std;` line allows the program to use all the entities in the `std` namespace.
- **Main Function**: This is the entry point of the program.
- A comment indicates where the code to find the mode should be inserted.
- The `cout` statement is used to output the most frequent value found and how many times it appeared.
## Visualization
There is a visual representation (pictured as a handwriting scribble) beside the code. It might suggest a flow or thought process, but it appears to be non-informative or illustrative for technical purposes.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
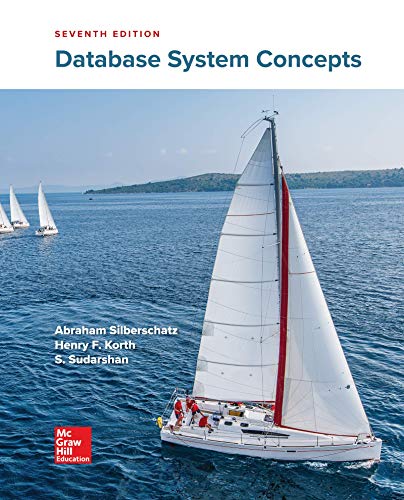
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
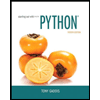
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
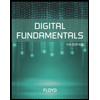
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
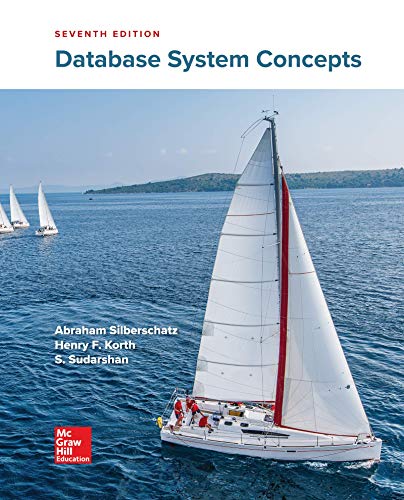
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
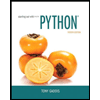
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
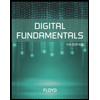
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
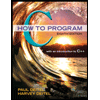
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
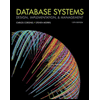
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
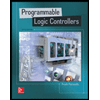
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education