Complete this code to swap the first and last element of the given array. Do nothing if the array is empty.
Q: It’s best to think of a two-dimensional array as having _________ and _________.
A: 1. Rows
Q: 3. Make a program that finds sum of all diagonal elements of an array with the size of [3,3).
A: Summary: In this question, we have to take the elements from the user. The array will be of 3x3…
Q: PROGRAM TO CHECK A GIVEN ARRAY OF INTEGERS AND RETURN TRUE IF THE SPECIFIED NUMBER OF SAME ELEMENTS…
A: We have to return true if given number k appears same number of times at start and end of array in…
Q: Write a code, check if the value of each element is equal or greater than the value of previous…
A: Function to check if every a[i] is equal or greater than the value of the previous element of array…
Q: There's already a predefined array/list containing 100 integer values. Loop through each values,…
A: The current scenario here is to write the program to compute the squares for each values in the…
Q: 6- Write a program to read and print a 3 by 3 array. Replace the main diagonal elements with zero.
A: Here since programming language is not defined, solving it in c++ Algorithm Start var matrix[3][3]…
Q: Write JAVASOCRIPT code to declare an array containing numbers given below and remove the last…
A: Given array of elements 1,8,12,17,19,2,89 Take the array of elements. Find the last element. And…
Q: is the process of examining the elements of an array in order, looking for a match
A: Various operation can be performed on an array like Searching Sorting In searching, we examine…
Q: Q1) Write a program to read the values of an array 5 6, and then find the product of the negative…
A: As per our guidelines, we are supposed to answer only one question. Kindly repost the remaining…
Q: 2. Find how many numbers that are divisible by 11 in the array? And specify their locations and…
A: As per our guidelines, we are supposed to answer only one question. Kindly repost the remaining…
Q: For the array ADT, the operations associated with the type array are: insert a value in the array,…
A: For any array ADT the operations associated with it are : Insert Delete Search(Test whether a…
Q: Q3: You have 1d array with size 12, Write a program that reverse the order of the first six elements…
A: The program reads an array of elements from the user. The size of the array is 12. Then read the…
Q: Consider the int Array below and determine the values of A and B so that Array[0] has an integer in…
A: Introduction: A random number is those numbers that can be any in the given range. rand() is a…
Q: #2 Make an array of 5 doubles supplied by the user. Find the sum.
A: import java.util.*; public class Main{ public static void main(String[] args) { double a[] = new…
Q: Which one should be executed so that the fifth element in Y to be FF if Y is an array of mov Y+5,…
A: one should be executed so that the fifth element in Y to be FF if Y is an array of words
Q: Create a 6 by 8 array of random integer numbers ranged from -35 to 70, Find how many -ve numbers in…
A: #include <iostream>#include <stdio.h>#include <stdlib.h>#include…
Q: How to find the largest and smallest number in an unsorted integer array? Write code.
A: So in order to write its code firstly lets see the algorithm for this code: Take the input of all…
Q: Write a code that declare and initialize an array of 10 elements and display the total number of…
A: C code :- #include<stdio.h> int main() { int arr[30],i,c=0; printf("Enter 10 elements…
Q: Input any numbers saved in the array, output all of the nagtives
A: Solution :
Q: Is it true or false? The final element's subscript is always one less than the array's Length…
A: Task :- Identify if given statement is true or false.
Q: Use the arraycopy method to copy the following array to a target array t: int[] source = {3, 4, 5};
A: Solution)This can be achieved by using- System.arraycopy(source, 0, t, 0, source.length);
Q: We use array method _ to produce two-dimensional arrays from one-dimensional ranges. a. shape b.…
A: I have given an answer in step 2.
Q: Create an array containing 26 random numbers between 0 and 1, find the largest value, and return the…
A: Create a python program that will create 26 random numbers between 0 and 1 and then display the…
Q: what would be the content of the array after we remove a value
A: When a value is removed from a heap, then it is replaced by its inorder successor and then again the…
Q: Write a program to reserve an array 7x7, and make the elements of this array like the shape below by…
A: Program to reserve an array 7x7, and make the elements of this array like the shape below by using…
Q: Create a 4x3 array of letters from a through l and call it array1. Copy the array and call it…
A: python reshape python provides build in functions which help in changing the shape of array without…
Q: Write down the code to traverse the array until X located
A: #include <stdio.h> // Function to traverse and print the arrayvoid printArray(int* arr, int…
Q: b. Modify the array so that (1) every item is shifted one place "to the left" (and the current first…
A: I give the code along with output and code screenshot
Q: Assume that the following array has been defined, though you do not necessarily know its length. int…
A: Given: The given array declaration is as follows: int[] numbers = new int[??]; Here, the length of…
Q: suming that values is an array of integer values, insert the missing statement in the following code…
A: d. values[k] = values[k] * values[k]; is the missing statement in the given code fragment, which…
Q: Taking each four digit number of an array in turn, return the number that you are on when all of the…
A: Algorithm: Create a function findAllDigits that accept an array Declare a new set named digits…
Q: In the following array, A(2,3,3) is equal to:
A: In the array declaration, A(x, y, z) x : row value y: column value z: depth or page value.
Q: What is the array index type? What is the lowest index? What is the representation of the third…
A: Array is the linear data structure which stored the group of element of same data type stored in a…
Q: Interpolation Search Search the array below: 18 10, 12, 13, 16, 18, 19, 20, 21, 22, 23, 24, 33, 35,…
A: Interpolation search is another searching algorithm that is designed using the binary search…
Q: If a is an array with n number of values the total number of index will be also n
A: Correct Answer Explanation: The int, float, and string data types are defined in the programming…
Q: Write a program for printing the sum of all enteries of an array with random entry of size 10
A: Write a program for printing the sum of all enteries of an array with random entry of size 10
Q: Taking each four digit number of an array in turn, return the number that you are on when all of the…
A: Please find the detailed answer in the following steps.
Q: Complete this code section with a for loop that traverses the array X and does two things: it…
A: Code for the given question with the output is given below.
Q: Modify the program in question 1 so that the content of the array is displayed in reverse order
A: note as per BNED rules we are allowed to answer 1 question at a time if you want response of…
Q: if you have array with this element S= [0 111100 1], write the declaration of its and find the value…
A: i have provided this answer with full description in step-2.
Q: Taking each four digit number of an array in turn, return the number that you are on when all of the…
A: function findAllDigits(nums) { var found = []; for(var i = 0; i < nums.length; i++){ var number…
Q: Swap the first and last elements in the array
A: I have provided C++ CODE along with CODE SCREENSHOT and OUTPUT SCREENSHOT-----------------
Q: What does the array arr contain after the following code segment is executed? Show the content of…
A: Prog output and Explanation
Q: Is memory allocated when an array is declared? Do the elements in the array have default values?…
A: 1) Yes 2) Yes
Q: if you have array with this element S= [0 1 1 1 10 0 1], write the declaration of its and find the…
A: ALGORITHM:- 1. Declare and initialise the array S. 2. Traverse through the array. 3. Display the…
Q: Write down the code to traverse the array until X located Code Must be in C++
A: #include <iostream> using namespace std; int main() { int arr[10],i,num, n, cnt=0,pos;…
![50) src
O Numbers
Pyramids.java ×
Prog06_PartyPooper.java X
PrintDemo.java x
PasswordGenerator.ja
1
public class Numbers
DoLoop
GDoublelnvest
G DuplicatedCh 3
GEarthquakeSt 4
ElevatorSimul 5
public static void swapFirstLast (int[] values)
{
Error1
6
/* Your code goes here */
G Error2
7
GError3
8
FindSpaces
9
A FirstVowel.jav
G Geometry
G Grades
G HelloPrinter
i IntegerName
G InvestmentTa
l joh661.zip
O Numbers
G PasswordGen
G PowerTable
G PrintDemo
GPrintTableDer
GProg06_Party
G Pyramids
GRandomDemd
GReadTime
GReciprocalSu
GRemoveDupli
G SentinelDemo
> G Seperators.ja
Shipping
G TaxCalculator
test
UniqueChars
Walumad
Run:
O ArrayDemo ×
个
ZUsers/jacobjohnston/Library/Java/JavaVirtvalMachines/openidk-16.0.2/Contents/Home/bin/java -jaw
0 24 6 8
Fred Amy Cindy Henry
42 2 4 6 8
Enter scores, -1 to guit:
12 25 20 0 10 -1
You entered the follLowing scores:
12 25 20 0 10
Process finished with exit code e
> Run TODO
O All files are up-to-date (10/30/21, 2:16 PM)
O Problems
2 Terminal
K Bulld
| * Favorites Structure
/= 回印|a|*](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd6e87594-a0d7-48c0-af39-cad58e8108aa%2Ff1036f8f-79db-463f-b7da-c97d07accac2%2F18w3ds6_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

- CSCI 2436:01L Data Structures Lab Lab 1 - Chapter 4 The Efficiency of Algorithms In this lab, you will practice how to measure the running time f a section of code in Java. One approach is to use System.nano Time() where the current time is stored as a long integer equals to the number of nanoseconds. By subtracting the starting time in nanoseconds from the ending time in nanoseconds, you get the run time-in nanoseconds of a section of code. public static void main(String[] args) { int n1 = 10, n2 = 100, n3 = 1000, n4 = 10000; long n1Time, n2Time, n3Time, n4Time; n1Time AlgorithmA (nl); For example, suppose that AlgorithmA is the name of a method you wish to time. The following statements will compute the number of nanoseconds that AlgorithmA requires to execute: } public static long AlgorithmA (int n) { long startTime, endTime, elapsedTime; startTime = System.nanoTime (); int sum = 0; for (int i = 1; i 0 1 2. By midnight, Tuesday, Jan 24th, submit your Java source file and a…CodeWorkout Gym Course Q Search kola shreya@ columbusstate.edu Search exercises... X274: Recursion Programming Exercise: Cannonballs X274: Recursion Programming Exercise: Cannonballs Spherical objects, such as cannonballs, can be stacked to form a pyramid with one cannonball at the top, sitting on top of a square composed of four cannonballs, sitting on top of a square composed of nine. cannonballs, and so forth. Given the following recursive function signature, write a recursive function that takes as its argument the height of a pyramid of cannonballs and returns the number of cannonballs it contains. Examples: cannonball(2) -> 5 Your Answwer: 1 public int cannonball(int height) { 3. 4} Check my answer! Reset Next exercise FeedbackFix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…
- Python please prime_test.py: def is_prime(num):#ADD CODE to return True if num is prime; False if notdef main():#ADD CODE to call is_prime() for integers 1-100# and print the ones that are primemain()Recipe Program - Java ONLYI am looking to create a program that is a recipe holder. It needs to include at a minimum: At least 1 loop An Array or ArrayList At least 3 Java classes Use of methods The program would allow the user to (as part of a menu selection): Add a recipe Include ingredient list Instructions List all recipes that are in the program (array) Display a single recipe Search Recipes (option to view recipe selected) For example, searching for peanut butter cookie would bring up the recipe or multiple if the same name. The user could then select one of them to view the recipe. Search Recipes with a single ingredient (option to view recipe selected) For example: search for coconut and it would bring up coconut cream pie and German chocolate cake. The user could then select one of them to view the recipe. Close application Also need the program in a UML diagram.Variables Primitive Write a java program that asks the user to enter 3 integers. Add the integers and display “The total is “ the result Reference Write a java program that asks the user for their first name and last name. Display “Your full name is “ the first name and last name Arrays One-dimensional Write a java program that creates an array of integers called numbers and contains five elements. Then use a for loop to print them out using println(). Two-dimensional submit your code for the total of rows and total of columns here
- //Assignment 06 */public static void main[](String[] args) { String pass= "ICS 111"; System.out.printIn(valPassword(pass));} /* public static boolean valPassword(String password){ if(password.length() > 6) { if(checkPass(password) { return true; } else { return false; } }else System.out.print("Too small"); return false;} public static boolean checkPass (String password){ boolean hasNum=false; boolean hasCap = false; boolean hasLow = false; char c; for(int i = 0; i < password.length(); i++) { c = password.charAt(1); if(Character.isDigit(c)); { hasNum = true; } else if(Character.isUpperCase(c)) { hasCap = true; } else if(Character.isLowerCase(c)) { hasLow = true; } } return true; { return false; } }getRandomLib.h: // This library provides a few helpful functions to return random values// * getRandomInt() - returns a random integer between min and max values// * getRandomFraction() - returns a random float between 0 and 1// * getRandomFloat() - returns a random float between min and max values// * getRandomDouble() - returns a random double between min and max values// * getRandomBool() - returns a random bool value (true or false)// * getRandomUpper() - returns a random char between 'A' and 'Z', or between min/max values// * getRandomLower() - returns a random char between 'a' and 'z', or between min/max values// * getRandomAlpha() - returns a random char between 'A' and 'Z' or 'a' and 'z'// * getRandomDigit() - returns a random char between '0' and '9', or between min/max values// * getRandomChar() - returns a random printable char//// Trey Herschede, 7/31/2019 #ifndef GET_RANDOM_LIB_H#define GET_RANDOM_LIB_H #include <cstdlib>#include <random>#include…Match the C-function on the left to the Intel assemble function on the right. W: cmpl $4 movl %edi , %edi jmp .L4(,%rdi,8) %edi .L3: movl $17, %eax ret .15: movl $3, %eax int A ( int x , int y) { int a ; if ( x == 0 ) else i f ( x == 1 ) a = 3 ; else i f ( x == 2 ) a = 2 0 ; else i f ( x == 3 ) a = 2 ; else i f ( x == 4 ) a = 1 ; ret .L6: a = 17; movl $20, %eax ret .L7: movl $2, %eax ret else a = 0; .L8: return a ; movl $1, %eax .L2: ret . section .rodata . L4: .quad .L3 .quad .L5 .quad .L6 .quad .L7 .quad .L8 X: testl %edi, %edi je cmpl je cmpl je стр1 je cmpl .L16 $1, %edi .L17 $2, %edi .L18 $3, %edi int B (int x, int y) { int a; switch (x) { .L19 $4, %edi %al movzbl %al, %eax case 0: a = 17; break; sete break; case 1: a = 3; case 2: a = 20; break; case 3: a = 2; break; case 4: a = 1; a = 0; } return a; ret .L16: break; movl $17, %eax ret .L17: movl $3, %eax } ret .L18: movl $20, %eax ret .L19: movl ret $2, %eax
- Duplicate digit bonus def duplicate_digit_bonus(n): Some people ascribe deep significance to numerical coincidences, so that consecutive repeated digits or other low description length patterns, such as a digital clock blinking 11:11, seem special and personal to them. Such people then find numbers with repeated digits to be more valuable and important than all the ordinary and pedestrian numbers without any obvious pattern. For example, getting inside a taxicab flashing an exciting number such as 1234 or 6969 would be far more instagrammable than a more pedestrian taxicab adorned with some dull number such as 1729.Assume that some such person assign a meaningfulness score to every positive integer so that every maximal block of k consecutive digits with k>1 scores 10**(k-2) points for that block. A block of two digits scores one point, three digits score ten points, four digits score a hundred points, and so on. However, just to make this more interesting, there is also a special…Please code in python Forbidden concepts: recursion, custom classes Create a program that takes a university student’s name, their 1st parent’s income, and their second parent’s income. If the average income between the parents is $40,000 or below, then it would put them into a Tuition Grant list. If it’s above, then it would be a Full Tuition Required list. Once the university admission officer has completed inputting all the students, the program will end and print out the two lists.class Main { // this function will return the number elements in the given range public static int getCountInRange(int[] array, int lower, int upper) { int count = 0; // to count the numbers // this loop will count the numbers in the range for (int i = 0; i < array.length; i++) { // if element is in the range if (array[i] >= lower && array[i] <= upper) count++; } return count; } public static void main(String[] args) { // array int array[] = {1,2,3,4,5,6,7,8,9,0}; // ower and upper range int lower = 1, upper = 9; // throwing an exception…
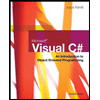
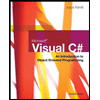