CODING IN PYTHON: Please help with Python I want to see written code, not screen shots, please.. 1. Write a Python program that will convert your height from feet and inches, to centimeters. Be sure to explain to possible users what the program will do. 2. Write a Python program to print the calendar of a given month and year. NOTE: Use 'calendar' module. 3. Write a Python program that will take a number of feet being input by a user, and convert the feet to inches, yards, and miles. The results of this conversion will be displayed when the program is run.
CODING IN PYTHON: Please help with Python I want to see written code, not screen shots, please..
1. Write a Python program that will convert your height from feet and inches, to centimeters. Be sure to explain to possible users what the program will do.
2. Write a Python program to print the calendar of a given month and year.
NOTE: Use 'calendar' module.
3. Write a Python program that will take a number of feet being input by a user, and convert the feet to inches, yards, and miles. The results of this conversion will be displayed when the program is run.

PYTHON CODE:
# getting the data from the user
data=input("Enter your height in feet and inches(e.g 5 5 ,sep by space):")
# checking for valid data
if data:
# splitting the data by spaces
t=data.split()
# converting string into float
feet=float(t[0])
inches=float(t[1])
cm=0
# calculating the cm
cm=feet * 30.48 + inches * 2.54
# printing the result
print("%s Feet,%s Inches equivalent to %.2f cm" %(str(feet),str(inches),cm))
else:
print("There is no data to process.")
2. PYTHON CODE:
import calendar
# getting the month and year
m=int(input("Enter the month: "))
y=int(input("Enter the year: "))
# printing the calendar
print(calendar.month(y,m))
3. PYTHON CODE:
# getting the input
feet=float(input("Enter the feet: "))
# converting the feet to inches
inches=feet * 12
# converting the feet to yards
yards= feet * 0.333333
# converting the feet to miles
miles= feet * 0.000189393939
print("Feet: ",feet)
print("Inches: ",inches)
print("Yards: ",yards)
print("Miles: ",miles)
SCREENSHOT FOR OUTPUT:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

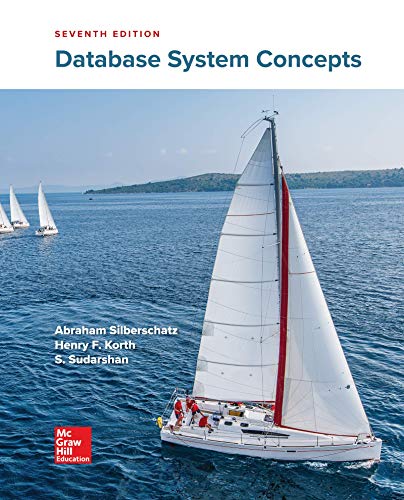
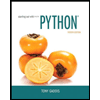
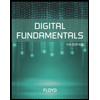
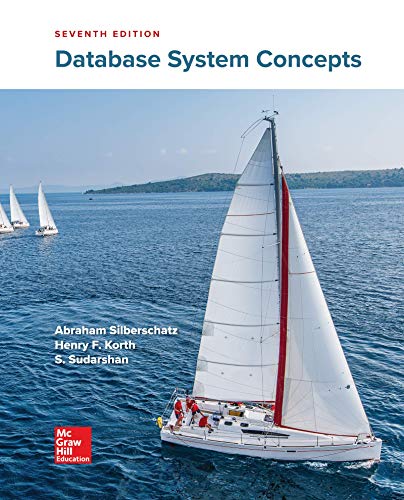
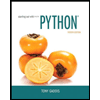
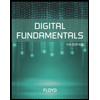
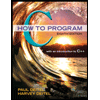
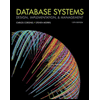
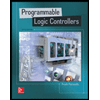