Change this code in C to Create functions and function prototypes to replace each of the calculator actions. Prototype each function above main and write the function (definition) below main. - The input by the user and output statement of each calculation should stay in main and not be moved to a function except the output for function “iseven” which should be done in the function. - Each function will accept the value(s) supplied by the user as parameters and return the result in a return statement (not a printf statement except for “iseven”). - The result returned by each function should be outputted by a printf statement in main. - Create a function named display that contains: menu display statements, the user prompt to select an option, user input. This function should have no parameters and should return a character as the option chosen by the user. ---------------------------------------------------------------------------------------------------------- #include #include int main(void) { char menuoption; int intx, inty, answeri, count, n, fact; double doublex, doubley, answerd; //prompt user do{ //menu printf("\nOperation Menu Option\n"); printf("Add A\n"); printf("Subtract S\n"); printf("Multiply M\n"); printf("Divide D\n"); printf("Remainder R\n"); printf("Squared V\n"); printf("Square Root T\n"); printf("Factorial F\n"); printf("Is even E\n"); printf("Greater integer G\n"); printf("Less integer L\n"); printf("Exit program X\n\n"); printf("Please enter a menu option from the table above: "); scanf("%c", &menuoption); switch (menuoption) { //addition case'a': case'A': printf("Please enter the first number: "); scanf("%lf", &doublex); printf("Please enter the second number: "); scanf("%lf", &doubley); answerd= doublex + doubley; printf("Answer = %.2lf", answerd); break; //subtraction case 's': case'S': printf("Please enter the first number:"); scanf("%lf", &doublex); printf("Please enter the second number:"); scanf("%lf", &doubley); answerd= doublex-doubley; printf("Answer = %.2lf", answerd); break; //multiplication case 'm': case'M': printf("Please enter the first number:"); scanf("%lf", &doublex); printf("Please enter the second number:"); scanf("%lf", &doubley); answerd= doublex * doubley; printf("Answer = %.2f\n", answerd); break; //division case 'd': case'D': printf("Please enter the first number:"); scanf("%lf", &doublex); printf("Please enter the second number:"); scanf("%lf", &doubley); if (doubley == 0) //checking if denominator is zero printf("invalid, cannot divide by zero\n"); else { answerd= doublex / doubley; printf("Answer = %.2f\n", answerd); } break; //remainder case 'r': case'R': printf("Please enter the first number:"); scanf("%d", &intx); printf("Please enter the second number:"); scanf("%d", &inty); answeri= intx % inty; printf("Answer = %d\n", answeri); break; //squared case 'v': case'V': printf("Please enter the number to be squared:"); scanf("%lf", &doublex); answerd= doublex*doublex; printf("Answer = %.2lf", answerd); break; //square root case 't': case'T': printf("Please enter the number to take the square root of:"); scanf("%lf", &doublex); if (doublex < 0) // checking is number for square root is negative printf("invalid, cannot square root negative number\n"); else { answerd= sqrt(doublex); printf("Answer = %.2f\n", answerd); } break; //factorial case 'f': case'F': fact = 1; printf("Please enter the number to take the factorial:"); scanf("%d", &n); if (n<0) printf("Cannot take the factorial of a negative number."); else if (n>0) { for (count= n ; count>= 1; count--) { fact = fact * count; } printf("Factorial = %d", fact ); } else printf("The factorial of 0 is 1."); break; //even case 'e': case'E': printf("Please enter the first number:"); scanf("%d", &intx); answeri= intx % 2; if (answeri==0) printf("The number is even.\n"); else printf("The number is not even.\n"); break; //greater integer case 'g': case'G': printf("Please enter the first number:"); scanf("%lf", &doublex); answeri= ceil(doublex); printf("Answer = %d", answeri); break; //lesser integer case 'l': case'L': printf("Please enter the first number:"); scanf("%d", &doublex); answeri= floor(doublex) ; printf("Answer = %d", answeri); break; //exit program case 'x': case'X': return 0; break; //improper input default: printf("Input not recognized.\n"); } } while (1); return 0; }
Change this code in C to Create functions and function prototypes to replace each of the
calculator actions. Prototype each function above main and write the
function (definition) below main.
- The input by the user and output statement of each calculation should
stay in main and not be moved to a function except the output for
function “iseven” which should be done in the function.
- Each function will accept the value(s) supplied by the user as parameters
and return the result in a return statement (not a printf statement
except for “iseven”).
- The result returned by each function should be outputted by a printf
statement in main.
- Create a function named display that contains: menu display
statements, the user prompt to select an option, user input. This
function should have no parameters and should return a character as
the option chosen by the user.
----------------------------------------------------------------------------------------------------------
#include<math.h>
#include<stdio.h>
int main(void)
{
char menuoption;
int intx, inty, answeri, count, n, fact;
double doublex, doubley, answerd;
//prompt user
do{
//menu
printf("\nOperation Menu Option\n");
printf("Add A\n");
printf("Subtract S\n");
printf("Multiply M\n");
printf("Divide D\n");
printf("Remainder R\n");
printf("Squared V\n");
printf("Square Root T\n");
printf("Factorial F\n");
printf("Is even E\n");
printf("Greater integer G\n");
printf("Less integer L\n");
printf("Exit program X\n\n");
printf("Please enter a menu option from the table above: ");
scanf("%c", &menuoption);
switch (menuoption)
{
//addition
case'a': case'A':
printf("Please enter the first number: ");
scanf("%lf", &doublex);
printf("Please enter the second number: ");
scanf("%lf", &doubley);
answerd= doublex + doubley;
printf("Answer = %.2lf", answerd);
break;
//subtraction
case 's': case'S':
printf("Please enter the first number:");
scanf("%lf", &doublex);
printf("Please enter the second number:");
scanf("%lf", &doubley);
answerd= doublex-doubley;
printf("Answer = %.2lf", answerd);
break;
//multiplication
case 'm': case'M':
printf("Please enter the first number:");
scanf("%lf", &doublex);
printf("Please enter the second number:");
scanf("%lf", &doubley);
answerd= doublex * doubley;
printf("Answer = %.2f\n", answerd);
break;
//division
case 'd': case'D':
printf("Please enter the first number:");
scanf("%lf", &doublex);
printf("Please enter the second number:");
scanf("%lf", &doubley);
if (doubley == 0) //checking if denominator
is zero
printf("invalid, cannot divide by zero\n");
else
{
answerd= doublex / doubley;
printf("Answer = %.2f\n", answerd);
}
break;
//remainder
case 'r': case'R':
printf("Please enter the first number:");
scanf("%d", &intx);
printf("Please enter the second number:");
scanf("%d", &inty);
answeri= intx % inty;
printf("Answer = %d\n", answeri);
break;
//squared
case 'v': case'V':
printf("Please enter the number to be squared:");
scanf("%lf", &doublex);
answerd= doublex*doublex;
printf("Answer = %.2lf", answerd);
break;
//square root
case 't': case'T':
printf("Please enter the number to take the square root of:");
scanf("%lf", &doublex);
if (doublex < 0) // checking
is number for square root is negative
printf("invalid, cannot square root negative number\n");
else
{
answerd= sqrt(doublex);
printf("Answer = %.2f\n", answerd);
}
break;
//factorial
case 'f': case'F':
fact = 1;
printf("Please enter the number to take the factorial:");
scanf("%d", &n);
if (n<0)
printf("Cannot take the factorial of a negative number.");
else if (n>0)
{
for (count= n ; count>= 1; count--)
{
fact = fact * count;
}
printf("Factorial = %d", fact );
}
else
printf("The factorial of 0 is 1.");
break;
//even
case 'e': case'E':
printf("Please enter the first number:");
scanf("%d", &intx);
answeri= intx % 2;
if (answeri==0)
printf("The number is even.\n");
else
printf("The number is not even.\n");
break;
//greater integer
case 'g': case'G':
printf("Please enter the first number:");
scanf("%lf", &doublex);
answeri= ceil(doublex);
printf("Answer = %d", answeri);
break;
//lesser integer
case 'l': case'L':
printf("Please enter the first number:");
scanf("%d", &doublex);
answeri= floor(doublex) ;
printf("Answer = %d", answeri);
break;
//exit program
case 'x': case'X':
return 0;
break;
//improper input
default:
printf("Input not recognized.\n");
}
} while (1);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 10 images

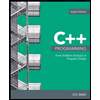
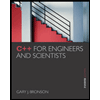
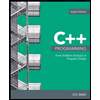
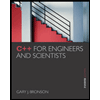