car_year is read from input. Write multiple if statements: • If car_year is before 1968, output 'Probably has few safety features. • If car_year is after 1969, output 'Probably has seat belts. • If car_year is after 1990, output 'Probably has electronic stability control. If car_year is after 2002, output 'Probably has airbags. ● ► Click here for examples 1 car_year= int(input()) 2 if car_year < 1968 3 print ('Probably has few safety features. 'D 4 elif car_year > 1969: 5 print ('Probably has seat belts.') 6 elif car_year > 1990: 7 print ('Probably has electronic stability control.') 8 elif car_year > 2002: 9 print ('Probably has airbags.')
car_year is read from input. Write multiple if statements: • If car_year is before 1968, output 'Probably has few safety features. • If car_year is after 1969, output 'Probably has seat belts. • If car_year is after 1990, output 'Probably has electronic stability control. If car_year is after 2002, output 'Probably has airbags. ● ► Click here for examples 1 car_year= int(input()) 2 if car_year < 1968 3 print ('Probably has few safety features. 'D 4 elif car_year > 1969: 5 print ('Probably has seat belts.') 6 elif car_year > 1990: 7 print ('Probably has electronic stability control.') 8 elif car_year > 2002: 9 print ('Probably has airbags.')
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
100%

Transcribed Image Text:### Understanding Conditional Statements in Python
In this example, we have a task where `car_year` is read from input, and we need to write multiple `if` statements based on different conditions:
- If `car_year` is before 1968, the output is `'Probably has few safety features.'`
- If `car_year` is after 1969, the output is `'Probably has seat belts.'`
- If `car_year` is after 1990, the output is `'Probably has electronic stability control.'`
- If `car_year` is after 2002, the output is `'Probably has airbags.'`
Here is the Python code that solves this:
```python
car_year = int(input())
if car_year < 1968:
print('Probably has few safety features.')
elif car_year > 1969:
print('Probably has seat belts.')
elif car_year > 1990:
print('Probably has electronic stability control.')
elif car_year > 2002:
print('Probably has airbags.')
```
### How It Works:
1. **Input Statement**:
```python
car_year = int(input())
```
- This line takes a year as input and converts it to an integer, storing it in the variable `car_year`.
2. **Conditional Statements**:
- The `if` statement checks if the `car_year` is less than 1968. If true, it prints `'Probably has few safety features.'`.
- The `elif` statements check subsequent conditions in order:
- If `car_year` is greater than 1969, it prints `'Probably has seat belts.'`.
- If `car_year` is greater than 1990, it prints `'Probably has electronic stability control.'`.
- If `car_year` is greater than 2002, it prints `'Probably has airbags.'`.
### Important Notes:
- The statements are checked in sequence. Once a condition is true, the corresponding block of code is executed, and the rest are skipped.
- Ensure proper ordering of conditions to achieve the desired logic flow.
- The use of `elif` (else if) helps streamline multiple conditions in a readable way.
This code snippet is useful to determine potential safety features based on the car's manufacturing year.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
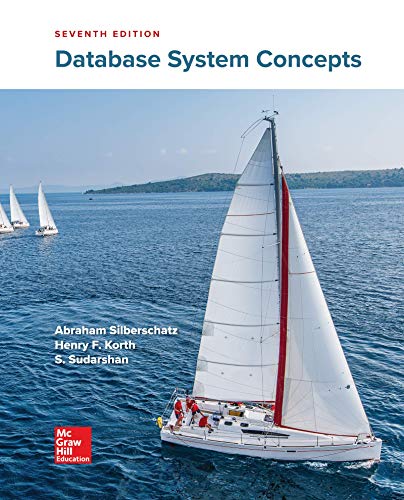
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
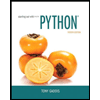
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
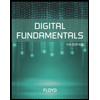
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
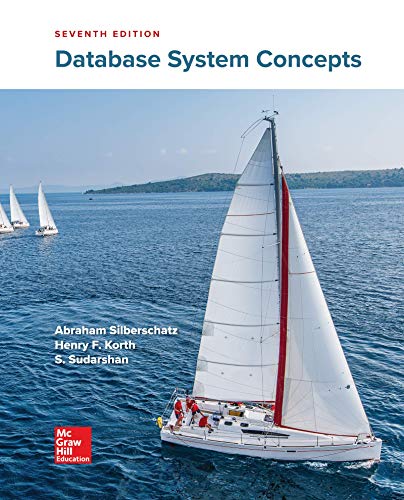
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
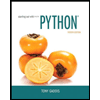
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
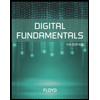
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
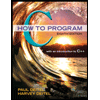
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
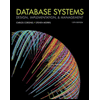
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
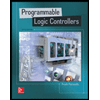
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education