Python
Background
Once upon a time, in a certain medieval village, a group of mysterious strangers appeared in jeans and T-shirts. The strangers managed to learn enough Old English to explain that they had been enjoying their favourite pastime – belting out tunes at a karaoke party – when they saw a blinding flash and heard a thunderous roar, lost consciousness, and found themselves transported back in time without any explanation.
The villagers were most interested in the strangers’ wide-ranging knowledge of popular songs from the future. They understood that the strangers belonged to some sort of bard class. The villagers were also party animals, and had a feast every night. The bards agreed to come to some of the parties and sing one Billboard Top 40 song whenever they did. When they weren’t there, the villagers would sing these songs to each other, reverently, knowing that they held clues to the future of their world. The more they learned, the more they were able to share, and some were even initiated into the mysterious strangers’ inner circle and allowed to become bards themselves.
Your task
Simulate the above village with its nightly parties.
You’ll be given a list of villagers and bards. You’ll also get a list of songs. The bards start out knowing every song, but no one else knows any songs.
You’ll be given a list of parties with their attendees, in the order that the parties are held. Every party features singing. There are two ways a party can unfold:
If there’s at least one bard present, they sing a song that none of the regular villagers at the party know yet. Specifically, the bard sings the first new song alphabetically, according to Python’s sort order of strings.
If there are no bards present, everyone at the party sings all the songs they know at that point.
Either way, whenever a song is sung, everyone at the party learns and remembers it.
If a villager learns enough songs, and they’re at a party with a bard, they too become a bard and learn every song.
Finally, you’ll calculate some statistics about the simulation.
Sets
One of the concepts you’ll be practicing throughout this assignment is sets.
A set is very much like a list, with a few differences:
There are no duplicate values in a set. No matter what you do, values are always unique.
Sets have no order. There are no indices and you can’t access individual elements.
Checking whether a value is in a set is instantaneous, no matter the size.
Sets have their own set of operations as described in set theory. You may find set union and set difference useful and, in general, you will find sets a valuable tool throughout this assignment.
Input File
Each test case is one input file. Here’s a sample input file:
VILLAGERS Luke Sawczak Dan Zingaro* Freddie Prinze Jr. Arnold Rosenbloom SONGS People, I've Been Sad Call Me Maybe What a Man Gotta Do Delete Forever PARTIES Dan Zingaro,Freddie Prinze Jr.,Arnold Rosenbloom Arnold Rosenbloom,Luke Sawczak,Freddie Prinze Jr. Dan Zingaro,Luke Sawczak Freddie Prinze Jr.,Luke Sawczak,Arnold Rosenbloom
People with an asterisk after their name are bards. However, the asterisk isn’t part of their name – notice there are no asterisks in the list of parties.*
Of course, there may be any number of villagers, bards, songs, and parties (including zero). An important task is to write the function, which parses the input into the villagers, bards, songs, and parties structures in Python. (HINT: how can you tell when one part of the file ends? You need to keep track of which part of the file you are working on now!)read_input
Statistics
After the simulation, you will prepare and return these stats on your village’s parties:
unheard_songs: The set of songs that have never been heard by non-bards.
billboard_top: A list of the n best-known songs in descending order from songs known by the most people to songs known by the fewest. Break ties alphabetically, according to Python’s sort order of strings. (HINT: the list method is going to be very helpful here. What criterion does it sort on by default? How many times will you need to sort? What can the parameter be used for?)sortkey
all_bards: The set of people who are bards, whether they started out as one or became one by learning enough songs.
average_attendees: The average number of people at a party. Round up to the nearest integer (so both 1.7 and 1.1 should become 2).
![A BARD DAY'S NIGHT
a.k.a. BARD ROCK CAFE
a.k.a. THE SCHOOL OF BARD KNOCKS
a.k.a. A BARD RAIN'S A-GONNA FALL
# Imports
from typing import Optional, TextI0 # Specific annotations
from math import ceil # For stats
|
# Constants
# Minimum number of songs for a villager to be promoted to a bard
BARD_THRESHOLD = 10
# Number of songs for the billboard_top statistic
BILLBOARD_N = 40
# DO NOT use these as variables in your code;
# they are only for type contracts.
# Maps from {name: songs that this villager knows}
villagers_type = dict[str, set[str]]
bards_type = set[str]
# Maps from {song name: names of people, including bards, that know this song}
songs_type = dict[str, set[str]]
# A list of parties; each party is a set of the attendee names
parties_type = list[set[str]]
def read_input (
f: Text10,
5) -> tuple [villagers_type, bards_type, songs_type, parties_type]:
A 2 A 31
) -> tuple [villagers_type, bards_type, songs_type, parties_type]:
JE JE JE
Read the given file and return the villagers, bards, songs, and parties.
f is an open file containing VILLAGERS and bards, SONGS, and PARTIES,
in that order. One villager or bard per line;
one song per line; one party per line, consisting of attendees
separated by commas. The parties are given in the order they're held.
201
# TODO
pass
# Party functions
#We highly recommend adding helper functions here!
def sing_at_party
villagers: villagers_type, bards: bards_type, songs: songs_type, party: set[str]
) -> None:
A bard sings if present, otherwise the villagers sing.
# TODO
pass
def update_bards_after_party(
villagers: villagers_type, bards: bards_type, songs: songs_type, party: set[str]
5) -> None:
###
Promote attendees who have learned enough songs to bards,
iff there is another bard present at the party.
# TODO
pass
# Stats functions
def unheard_songs (
A 2 A 31 V
E](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8b9e7b26-fa3a-4338-9695-86acdf518073%2Fd4d584e8-469a-4f43-b58b-659e587b5b25%2Fq6okqlu8_processed.png&w=3840&q=75)
![def unheard_songs (
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> set[str]:
Return a set of songs that have never been heard by non-bards.
(This means that only the bards know it.)
11 11 11
# TODO
pass
def billboard_top(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
3) -> list[str]:
Return a list of the BILLBOARD_N most popular songs by number of people
who know them, in descending order. Break ties alphabetically.
# TODO
pass
def all_bards (
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
parties: parties_type,
) -> set[str]:
"""Return the set of the village's bards."""
# TODO
pass
def average_attendees(
villagers: villagers_type,
bards: bards_type,
songs: songs_type,
A 2 A 31 A
1
songs: songs_type,
parties: parties_type,
) -> int:
Return the average number of attendees at parties in the village.
Round up to the nearest integer.
11 11 11
# TODO
pass
# Main process
def run(filename: str) -> dict[str, object]:
111111
Run the program: read the input, host the parties,
and return a dictionary of resulting statistics keyed by name:
unheard_songs, billboard_top, all_bards, average_attendees
filename is the name of an input file.
# TODO
pass
# Run program
if __name__ == "__main__":
# Sample input from the handout -- you can tweak this if you like
stats_handout = run("handout_example.txt")
print("Results of handout sample input")
for key, value in stats_handout.items():
print (f" (key}: {value}")
print()
# Sample bigger input -- you can tweak this if you like
stats_bigger = run("bigger example.txt")
print("Results of bigger
A 2 A 31](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8b9e7b26-fa3a-4338-9695-86acdf518073%2Fd4d584e8-469a-4f43-b58b-659e587b5b25%2F06ydjcc_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

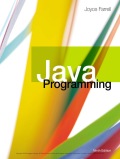
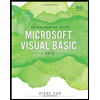
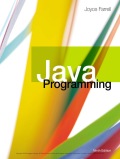
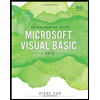
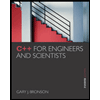
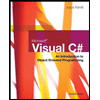