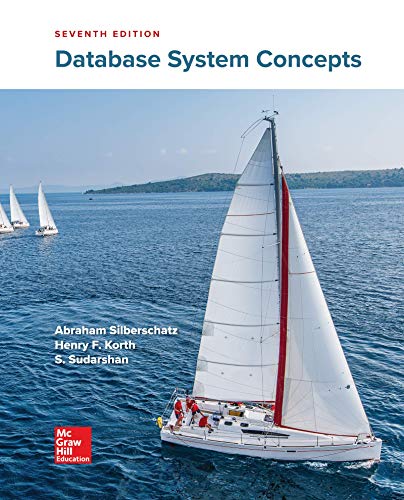
Concept explainers
can someone help me fix this code so it outputs properly
In order to better organize its business, the Lawrenceville Lawn Service wants to computerize its customer list. The information for each customer(name, address, size of lawn, and day of the week they wish their lawn to be mowed) should be stored in parallel arrays. Write a program that allows the user to perform the following functions:
Add a new customer
Remove a customer
Display a table of jobs for a given day. The table should be similar to the one shown in the sample run below, showing the name, address, cost and total charge including 7% sales tax. LLS charges 2 cents per square yard for cutting.
When run, the program output should look similar to:
Enter Display, Add, dElete, Quit: a ADD Enter name to be added: Tiger Woods Enter address: 65 Lakeshore Drive Enter lawn size: 1262 Enter day to be cut: Saturday Enter Display, Add, dElete, Quit: a ADD Enter name to be added: Julia Winitsky Enter address: 16 Manor Dr Enter lawn size: 2500 Enter day to be cut: Tuesday Enter Display, Add, dElete, Quit: e Delete Enter name for deletion: Tiger Woods ** DELETED ** Enter Display, Add, dElete, Quit: e DELETE Enter name for deletion: Josephine Bouchard ** Error Name not on list ** Enter Display, Add, dElete, Quit: X ** Please enter D, A, E, or Q ** Enter Display, Add, dElete, Quit: d DISPLAY Enter day for list: Tuesday LAWRENCEVILLE LAWN SERVICE Schedule for: Tuesday NAME ADDRESS COST TOTAL Julia Winitsky 16 Manor Dr. $50.00 $53.50 Enter Display, Add, dElete, Quit: q QUIT ** Program complete **
code:
#include <iostream>
#include <string>
#include <iomanip>
using namespace std;
const double COST_PER_SQ_YARD = .02;
const double TAX_RATE = .07;
string name[20];
string address[20];
int size[20];
string day[20];
int customerCount = 0;
void displayMenu();
void addCustomer();
void deleteCustomer();
void displayListByDay();
int main()
{
char userInput;
cout << "Welcome to Lawrenceville Lawn Service!" << endl;
displayMenu();
cin >> userInput;
userInput = toupper(userInput);
while (userInput != 'Q'){
switch (userInput)
{
case 'D':
displayListByDay();
break;
case 'A':
addCustomer();
break;
case 'E':
deleteCustomer();
break;
default:
cout << "Please enter D, A, E, or Q" << endl;
break;
}
displayMenu();
cin >> userInput;
userInput = toupper(userInput);
}
cout << "Program complete" << endl;
return 0;
}
void displayMenu()
{
cout << "Enter Display, Add, dElete, Quit: ";
}
void addCustomer()
{
string nameInput;
string addressInput;
int sizeInput;
string dayInput;
cout << "ADD" << endl;
cout << "Enter name to be added: ";
cin >> nameInput;
cout << "Enter address: ";
cin >> addressInput;
cout << "Enter lawn size: ";
cin >> sizeInput;
cout << "Enter day to be cut: ";
cin >> dayInput;
name[customerCount] = nameInput;
address[customerCount] = addressInput;
size[customerCount] = sizeInput;
day[customerCount] = dayInput;
customerCount++;
}
void deleteCustomer()
{
string nameInput;
bool deleted = false;
cout << "DELETE" << endl;
cout << "Enter name for deletion: ";
cin >> nameInput;
for (int i = 0; i < customerCount; i++)
{
if (name[i] == nameInput)
{
for (int j = i; j < customerCount - 1; j++)
{
name[j] = name[j + 1];
address[j] = address[j + 1];
size[j] = size[j + 1];
day[j] = day[j + 1];
}
customerCount--;
deleted = true;
break;
}
}
if (deleted)
cout << "** DELETED **" << endl;
else
cout << "** Error Name not on list **" << endl;
}
void displayListByDay()
{
string dayInput;
cout << "DISPLAY" << endl;
cout << "Enter day for list: ";
cin >> dayInput;
cout << endl;
cout << "LAWRENCEVILLE LAWN SERVICE" << endl;
cout << "Schedule for: " << dayInput << endl;
cout << endl;
cout << left << setw(20) << "NAME";
cout << left << setw(20) << "ADDRESS";
cout << left << setw(20) << "COST";
cout << left << setw(20) << "TOTAL" << endl;
for (int i = 0; i < customerCount; i++)
{
if (day[i] == dayInput)
{
cout << left << setw(20) << name[i];
cout << left << setw(20) << address[i];
cout << left << setw(20) << fixed << setprecision(2) << "$" << size[i] * COST_PER_SQ_YARD;
cout << left << setw(20) << fixed << setprecision(2) << "$" << (size[i] * COST_PER_SQ_YARD) * (1 + TAX_RATE) << endl;
}
}
}

Step by stepSolved in 3 steps

- Please solve this computer science problem.arrow_forwardWhich of the following statements are true. An array is one name for several memory locations. Memory for elements of an array is contiguous, i.e. the memory locations are side by side. Arrays may be 1, 2, 3 or even higher dimensional. All of the above statements are true.arrow_forwardFYI: Please write the code in Pseudocode (no programming language please) 1. Write pseudocode to load a single array with data. Then search that array for a match. Here are the specifics. You do NOT have to write the entire program. Load the array with data from a file named customerNumbers Ask the user to enter their customer number Search the array for the customer number If you find a match output FOUND If there is no match output NOT FOUNDarrow_forward
- PLEASE HELP ME WITH THIS! THANK YOU! Develop a program that will define and store a student record. The data will be entered by the user. Afterwards, calculate and display the GPA of each student. You will need to use structure with array. In case you don't know how to calculate GPA, here's the formula: Sample Console Input Expected Console Output 2John Anthony10912345ECEPhysics1003Mathematics702Geology501Jose10854321CPEPhysics803Mathematics602Geology901 Enter number of students to record: 2student name: John AnthonyID number: 10912345degree: ECEcourse 1 name: Physicscourse 1 grade: 100course 1 total units: 3course 2 name: Mathematicscourse 2 grade: 70course 2 total units: 2course 3 name: Geologycourse 3 grade: 50course 3 total units: 1student name: JoseID number: 10854321degree: CPEcourse 1 name: Physicscourse 1 grade: 80course 1 total units: 3course 2 name: Mathematicscourse 2 grade: 60course 2 total units: 2course 3 name: Geologycourse 3 grade: 90course 3 total units:…arrow_forwardEach of two players rolls a dice and whoever rolls the highest number (between 1 and 6 inclusive) gets 4 points, and if it is a draw, both players get 2 points. The player with the highest number of points after 10 rounds of the game is the winner (or the game could be drawn). The following arrays contain the results of dice rolls of each of two players in 10 consecutive rounds of the game.player1=[3, 2, 5, 6, 2, 5, 1, 6, 4, 5]player2=[4, 2, 4, 3, 6, 5, 3, 2, 4, 1] Write a MATLAB program that computes the running total points of each player and displays one of the following messages (whichever applies) after each round n for n = 1, 2, . . . , 10:Player 1 is leadingPlayer 2 is leadingIt is a tie [Hint: You should use an if construct.]Also, at the end of your program, use the find command to identify (and display) the rounds in which dice rolls were the same for the two players, and use the length command to compute (and display) the number of such rounds. Include appropriate headings in…arrow_forwardThis program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int array and the ratings in another int array. Output these arrays (i.e., output the roster). Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Player 1 -- Jersey number: 84, Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 (2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. The program initially outputs the menu, and outputs the menu after a user chooses an option. The…arrow_forward
- Common Time Zones Function Name: commonTimeZones() Parameters: code1( str ), code2( str) Returns: list of common time zones ( list ) Description: You and your friend live in different countries, but you figure there's a chance that both of you might be in the same time zone. Thus, you want to find out the list of possible time zones that you and your friend could both be in. Given two country codes, write a function that returns a list of the time zones the two countries have in common. Be sure not to include any duplicate time zones. If the two country codes do not have any common time zones, return the string 'No Common Time Zones' instead. Note: You can assume that the codes will always be valid. example test cases: >>> commonTimeZones('can', 'usa') [UTC-08:00', 'UTC-07:00', 'UTC-06:00', 'UTC-05:00', 'UTC-04:00] >>> commonTimeZones('rus', 'chn') [UTC+08:00] For this assignment, use the REST countries API (https://restcountries.com/#api-endpoints-v2). For all of your requests, make…arrow_forwardProgramming language: Processing from Java Question attached as photo Topic: Use of Patial- Full Arraysarrow_forwardIn C Programming Write a main function that declares an array of 100 ints. Fill the array with random values between 1 and 100. Calculate the average of the values in the array. Output the average.arrow_forward
- Calculate average test score: Write a program that reads 10 test scores in an array named testScores. Then pass this array to a function to calculate the average test scores, return the average to main function and display it. Input Validation: The test scores should be between 0 and 100 (inclusive). Add comments and version control.arrow_forwardIn this lab, you use what you have learned about searching an array to find an exact match to complete a partially prewritten C++ program. The program uses an array that contains valid names for 10 cities in Michigan. You ask the user to enter a city name; your program then searches the array for that city name. If it is not found, the program should print a message that informs the user the city name is not found in the list of valid cities in Michigan. The file provided for this lab includes the input statements and the necessary variable declarations. You need to use a loop to examine all the items in the array and test for a match. You also need to set a flag if there is a match and then test the flag variable to determine if you should print the the Not a city in Michigan. message. Comments in the code tell you where to write your statements. You can use the previous Mail Order program as a guide. Instructions Ensure the provided code file named MichiganCities.cpp is open. Study…arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
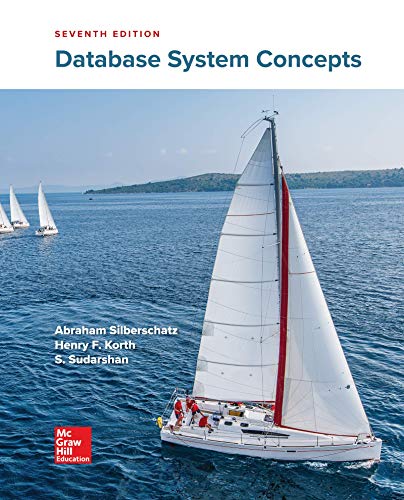
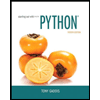
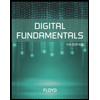
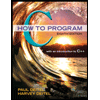
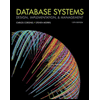
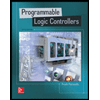