CALCULATOR For this task, you will create a simple calculator that performs addition, subtraction, multiplication, and division. Open the file calculator.html in your browser to see what your calculator will look like. Upon clicking the "Calculate!" button, your program should prompt the user for a number, an operator (+,-, *, or /), and another number. It should then print the input and answer on the calculator screen as shown in the examples. We have already set up the button on the page to call the function promptUser() when a user clicks it. The expectations for each of the three functions you must define are outlined below. You may find it easiest to work on one function at a time, checking your progress as you go by printing to the console. PROMPTUSER() FUNCTION The promptUser() function should first give the user three separate prompts for the first number, operator, and second number, respectively. The three separate prompt() calls to get the user input should be (in this order): "Enter first number: " "Enter operator (+,-, *, or /): " • "Enter second number: " It should then call the calc(x, operator, y) function to get the answer. Finally, it should call the printAnswer(x, operator, y, answer) function to print the answer to the page. CALC(X, OPERATOR, Y) FUNCTION The calc(x, operator, y) function takes the two numbers and operator as input and returns the answer. The parameters x and y should be the first and second numbers, respectively. The parameter operator is whatever operator the user entered. E.g., if the user enters "3", "+", and "5" (without the quotes), then this function should return "8". Input assumptions: You can assume the user gives you valid numbers for the two numbers. However, they may enter anything for the operator. If the operator they enter is not +, -, *, or /, then this function should return NaN (the javascript object NaN, not the string "NaN"). PRINTANSWER(X, OPERATOR, Y, ANSWER) FUNCTION The printAnswer(x, operator, y, answer) function takes the two numbers, operator, and answer as input. It prints the full equation to the page by adding it in a paragraph () to the element with id="calculatorDisplay". Note that to add a string to the end without replacing what's currently there, you use + instead of =. For example, executing the following line of code would add "5*2= 10": document.getElementById("calculator Display").innerHTML += "5 * 2 = 10"; If the answer is NaN the paragraph element added should belong to the class badinput. This will cause the text to display in red (as defined by the CSS rules for that class in calculator.css). You can check whether the answer is NaN using the existing function isNaN(). You can learn more about isNaN() here. Note that this function should not return anything. It simply adds another line to the display. The HTML file, calculator.html, starts with a few lines displayed in the calculator to illustrate what the output should look like. Your printAnswer(x, operator, y, answer) function should NOT replace the existing text. It should just add a new line each time. After adding a few lines, the CSS will automatically add a scroll bar to scroll down to the most recent line. The following picture shows what should display if the first query is 3, *, 7.
CALCULATOR For this task, you will create a simple calculator that performs addition, subtraction, multiplication, and division. Open the file calculator.html in your browser to see what your calculator will look like. Upon clicking the "Calculate!" button, your program should prompt the user for a number, an operator (+,-, *, or /), and another number. It should then print the input and answer on the calculator screen as shown in the examples. We have already set up the button on the page to call the function promptUser() when a user clicks it. The expectations for each of the three functions you must define are outlined below. You may find it easiest to work on one function at a time, checking your progress as you go by printing to the console. PROMPTUSER() FUNCTION The promptUser() function should first give the user three separate prompts for the first number, operator, and second number, respectively. The three separate prompt() calls to get the user input should be (in this order): "Enter first number: " "Enter operator (+,-, *, or /): " • "Enter second number: " It should then call the calc(x, operator, y) function to get the answer. Finally, it should call the printAnswer(x, operator, y, answer) function to print the answer to the page. CALC(X, OPERATOR, Y) FUNCTION The calc(x, operator, y) function takes the two numbers and operator as input and returns the answer. The parameters x and y should be the first and second numbers, respectively. The parameter operator is whatever operator the user entered. E.g., if the user enters "3", "+", and "5" (without the quotes), then this function should return "8". Input assumptions: You can assume the user gives you valid numbers for the two numbers. However, they may enter anything for the operator. If the operator they enter is not +, -, *, or /, then this function should return NaN (the javascript object NaN, not the string "NaN"). PRINTANSWER(X, OPERATOR, Y, ANSWER) FUNCTION The printAnswer(x, operator, y, answer) function takes the two numbers, operator, and answer as input. It prints the full equation to the page by adding it in a paragraph () to the element with id="calculatorDisplay". Note that to add a string to the end without replacing what's currently there, you use + instead of =. For example, executing the following line of code would add "5*2= 10": document.getElementById("calculator Display").innerHTML += "5 * 2 = 10"; If the answer is NaN the paragraph element added should belong to the class badinput. This will cause the text to display in red (as defined by the CSS rules for that class in calculator.css). You can check whether the answer is NaN using the existing function isNaN(). You can learn more about isNaN() here. Note that this function should not return anything. It simply adds another line to the display. The HTML file, calculator.html, starts with a few lines displayed in the calculator to illustrate what the output should look like. Your printAnswer(x, operator, y, answer) function should NOT replace the existing text. It should just add a new line each time. After adding a few lines, the CSS will automatically add a scroll bar to scroll down to the most recent line. The following picture shows what should display if the first query is 3, *, 7.
Chapter5: Making Decisions
Section: Chapter Questions
Problem 2GZ
Related questions
Question
What would this code look like in JavaScript + html?

Transcribed Image Text:CALCULATOR
For this task, you will create a simple calculator that performs addition, subtraction, multiplication, and
division.
Open the file calculator.html in your browser to see what your calculator will look like. Upon clicking the
"Calculate!" button, your program should prompt the user for a number, an operator (+,-, *, or /), and
another number. It should then print the input and answer on the calculator screen as shown in the
examples.
We have already set up the button on the page to call the function promptUser() when a user clicks it.
The expectations for each of the three functions you must define are outlined below. You may find it
easiest to work on one function at a time, checking your progress as you go by printing to the console.
PROMPTUSER() FUNCTION
The promptUser() function should first give the user three separate prompts for the first number,
operator, and second number, respectively. The three separate prompt() calls to get the user input should
be (in this order):
●
"Enter first number: "
"Enter operator (+,-, *, or /): "
"Enter second number: "
It should then call the calc(x, operator, y) function to get the answer. Finally, it should call the
printAnswer(x, operator, y, answer) function to print the answer to the page.
CALC(X, OPERATOR, Y) FUNCTION
The calc(x, operator, y) function takes the two numbers and operator as input and returns the answer.
The parameters x and y should be the first and second numbers, respectively. The parameter operator is
whatever operator the user entered. E.g., if the user enters "3", "+", and "5" (without the quotes), then this
function should return "8".
Input assumptions: You can assume the user gives you valid numbers for the two numbers. However,
they may enter anything for the operator. If the operator they enter is not +, -, *, or /, then this function
should return NaN (the javascript object NaN, not the string "NaN").
PRINTANSWER(X, OPERATOR, Y, ANSWER) FUNCTION
The printAnswer(x, operator, y, answer) function takes the two numbers, operator, and answer as input.
It prints the full equation to the page by adding it in a paragraph (<p>) to the element with
id="calculatorDisplay". Note that to add a string to the end without replacing what's currently there, you
use += instead of =. For example, executing the following line of code would add "5*2= 10":
document.getElementById("calculator Display").innerHTML
+= "<p>5 * 2 = 10</p>";
If the answer is NaN the paragraph element added should belong to the class badinput. This will cause
the text to display in red (as defined by the CSS rules for that class in calculator.css). You can check
whether the answer is NaN using the existing function isNaN(). You can learn more about isNaN() here.
Note that this function should not return anything. It simply adds another line to the display. The HTML
file, calculator.html, starts with a few lines displayed in the calculator to illustrate what the output should
look like. Your printAnswer(x, operator, y, answer) function should NOT replace the existing text. It
should just add a new line each time. After adding a few lines, the CSS will automatically add a scroll bar
to scroll down to the most recent line. The following picture shows what should display if the first query is
3, *, 7.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
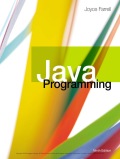
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
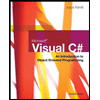
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
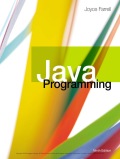
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
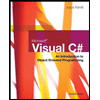
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
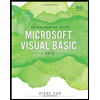
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
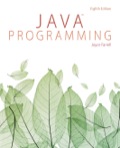
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT