C++, void traverseWithDijkstra(string start); ➔Use Dijkstra's algorithm to compute the single source shortest path in the graph from the start city to all other nodes in its component. ➔You don’t print anything in this function. Storing the distance associated with every node relative to the start node is enough. .hpp #ifndef GRAPH_H #define GRAPH_H #include #include using namespace std; struct vertex; struct adjVertex{ vertex *v; int weight; }; struct vertex{ vertex() { this->visited = false; this->distance = 0; this->pred = NULL; } string name; bool visited; int distance; vertex *pred; vector adj; }; class Graph { public: void createEdge(string v1, string v2, int num); void insertVertex(string name); void displayEdges(); void depthFirstTraversal(string sourceVertex); void traverseWithDijkstra(string sourceVertex); void minDistPath(string start, string end); private: vector vertices; };
C++,
void traverseWithDijkstra(string start);
➔Use Dijkstra's
➔You don’t print anything in this function. Storing the distance associated with every node relative to the start node is enough.
.hpp
#ifndef GRAPH_H
#define GRAPH_H
#include<
#include<iostream>
using namespace std;
struct vertex;
struct adjVertex{
vertex *v;
int weight;
};
struct vertex{
vertex() {
this->visited = false;
this->distance = 0;
this->pred = NULL;
}
string name;
bool visited;
int distance;
vertex *pred;
vector<adjVertex> adj;
};
class Graph
{
public:
void createEdge(string v1, string v2, int num);
void insertVertex(string name);
void displayEdges();
void depthFirstTraversal(string sourceVertex);
void traverseWithDijkstra(string sourceVertex);
void minDistPath(string start, string end);
private:
vector<vertex*> vertices;
};

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

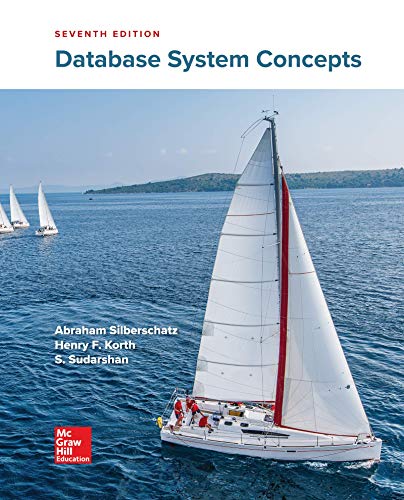
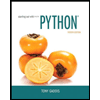
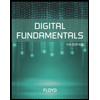
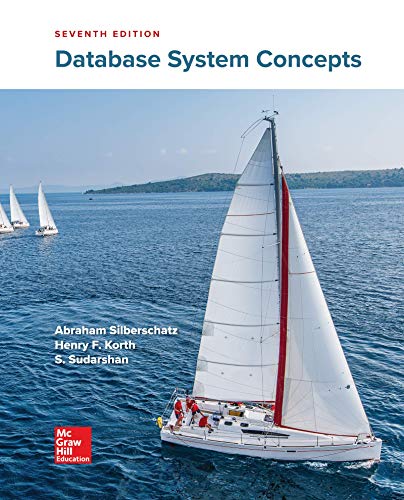
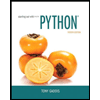
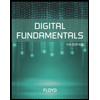
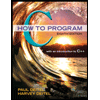
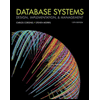
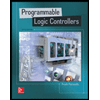