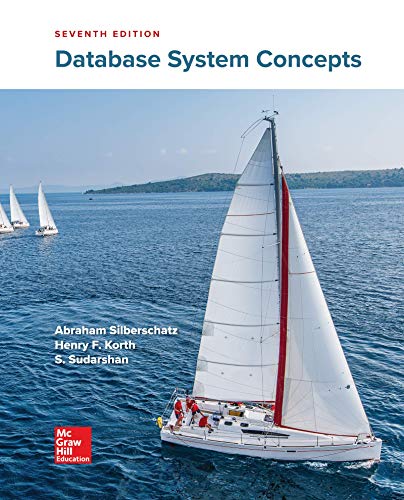
C++ Programming, Stack queue and deque
Improve the error code below, you can also create custom methods in the main class. See attached photo for the instructions.
main.cpp: In function ‘int main(int, char**)’: main.cpp:17:15: error: expected primary-expression before ‘char’ stack<char> s; ^~~~ main.cpp:21:17: error: ‘s’ was not declared in this scope s.push(x[i]); ^ main.cpp:22:37: error: ‘s’ was not declared in this scope else if (x[i]==')' and !s.empty()) ^ main.cpp:44:16: error: ‘s’ was not declared in this scope return (s.empty()); ^ main.cpp:47:14: error: jump to case label [-fpermissive] case 1: ^ main.cpp:16:16: note: crosses initialization of ‘std::__cxx11::string x’ string x=str; ^ main.cpp:56:14: error: jump to case label [-fpermissive] case 2: ^ main.cpp:16:16: note: crosses initialization of ‘std::__cxx11::string x’ string x=str; ^ main.cpp:74:14: error: jump to case label [-fpermissive] case 3: ^ main.cpp:16:16: note: crosses initialization of ‘std::__cxx11::string x’ string x=str; ^ main.cpp:90:14: error: jump to case label [-fpermissive] case 4: ^ main.cpp:16:16: note: crosses initialization of ‘std::__cxx11::string x’ string x=str; ^ main.cpp:110:14: error: jump to case label [-fpermissive] case 5: ^ main.cpp:16:16: note: crosses initialization of ‘std::__cxx11::string x’ string x=str; ^ main.cpp:130:14: error: jump to case label [-fpermissive] case 6: ^ main.cpp:16:16: note: crosses initialization of ‘std::__cxx11::string x’ string x=str; ^ g++: error: main.o: No such file or directory g++: fatal error: no input files compilation terminated.
main.cpp
![main.cpp: In function 'int main(int, char**)':
main.cpp:17:15: error: expected primary-expression before char'
stack<char> s;
main.cpp:21:17: error: 's' was not declared in this scope
s. push(x[i]);
main.cpp:22:37: error: 's was not declared in this scope
else if (x[i]--')' and !s.empty())
main.cpp:44:16: error: 's was not declared in this scope
return (s.empty());](https://content.bartleby.com/qna-images/question/2cb11186-80ff-4474-bc75-6eff69002743/8fc1cadf-bf9a-4bcc-8e8b-3bdfa942ad9b/0qj50i_thumbnail.png)
![Additionally, you are also going to solve the Brackets Problem in
the case O of the main.cpp file where you have to identify
whether a given string that contains brackets is valid or not.
Brackets may be parentheses ( and ), curly brackets { and },
and square brackets [ and ]. Each opening symbol must match
its corresponding closing symbol. For example, a left bracket,
"L" must match a corresponding right bracket, ")," as in the
following expression: [0]
Take note of the three considerations that will make the string
invalid: a mismatch where the closing bracket did not match
what was in the top of the stack (e.g. "(1)"), an excess opening
where we have completed checking the string where there
should have been more closing bracket/s (e.g. "(0"), and an
excess closing where we found a closing bracket but there's no
opening bracket to match it with (e.g. "0)").
Using the methods of a stack and your SLL implementation,
create a separate program that will print accepted if a given
string is valid exclusively in terms of brace pairing and
otherwise, print not accepted.](https://content.bartleby.com/qna-images/question/2cb11186-80ff-4474-bc75-6eff69002743/8fc1cadf-bf9a-4bcc-8e8b-3bdfa942ad9b/trr7l1_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- C# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. This would occur in the NumberStack class, not the main class. These are the provided variables: private int [] stack;private int size; Create a method that will reverse the stack when put into the main class.arrow_forwardFor the code shown in the screenshot below, draw a picture of the program stack when the function findSlope() is called the first time. You only have to draw the part of the stack for findZero() and findSlope().arrow_forwardNesting procedure calls how many return addresses will be on the stack by the time Sub3 is called? main PROC call Subl exit main ENDP Subl PROC call Sub2 ret Subl ENDP Sub2 PROC call Sub3 ret Rectangu Sub2 ENDP Sub3 PROC ret Sub3 ENDP 2 O1 3arrow_forward
- What will be the stack content after following operations: Push 5 Push 8 Pop Push 1 Push 12 Poparrow_forward1. A company wants to evaluate employee records in order to lay off some workers on the basis of service time (the most recently hired employees are laid off first). Only the employee ids are stored in stack. Write a program using stack and implement the following functions: Main program should ask the appropriate option from the user, until user selects an option for exiting the program. Enter_company (emp_id)- the accepted employee id(integer) is pushed into stack. Exit_company ()- The recently joined employee will be laid off from the company Show employee ()- display all the employees working in the company. count()- displays the number of employees working in the company. i) ii) iii) iv)arrow_forwardCPU Priority Scheduling-preemptive: Write a Java program to implement a priority scheduling algorithm that uses low number to represent high priority. Your program should first prompts the user to input a list of process ID, arrival time, burst time, and priority for each process to be run on the CPU. The list is terminated by 0 0 0 0 for the process ID, arrival time, burst time, and priority. The program output should draw a Gantt chart (as text) that shows the scheduling order of the processes using the Priority scheduling algorithm. Also print the turnaround time, response time, and waiting time for each process along with their average for all processes. Make sure to display very helpful messages to the user for input and output.arrow_forward
- 1)Write a Java program which stores three values by using singly linked list.- Node class1. stuID, stuName, stuScore, //data fields2. constructor3. update and accessor methods- Singly linked list class which must has following methods:1. head, tail, size// data fields2. constructor3. update and accessor methodsa. getSize() //Returns the number of elements in the list.b. isEmpty( ) //Returns true if the list is empty, and false otherwise.c. getFirstStuID( ), getStuName( ), getFirstStuScore( )d. addFirst(stuID, stuName, stuScore)e. addLast(stuID, stuName, stuScore)f. removeFirst ( ) //Removes and returns the first element of the list.g. displayList( ) //Displays all elements of the list by traversing the linked list.- Test class – initialize a singly linked list instance. Test all methods of singly linked list class. 2. Write a Java program which stores three values by using doubly linked list.- Node class4. stuID, stuName, stuScore, //data fields5. constructor6. update and…arrow_forwardstacks and queues. program must be able to handle all test cases without causing an exception Note that this problem does not require recursion to solve (though you can use that if you wish).arrow_forwardAll files are included below, seperated by the dashes. "----" //driver.cpp #include <iostream> #include <string> #include "stackLL.h" #include "queueLL.h" #include "priorityQueueLL.h" using namespace std; int main() { /////////////Test code for stack /////////////// stackLLstk; stk.push(5); stk.push(13); stk.push(7); stk.push(3); stk.push(2); stk.push(11); cout<<"Popping: "<<stk.pop() <<endl; cout<<"Popping: "<<stk.pop() <<endl; stk.push(17); stk.push(19); stk.push(23); while( ! stk.empty() ) { cout<<"Popping: "<<stk.pop() <<endl; } // output order: 11,2,23,19,17,3,7,13,5 stackLLstkx; stkx.push(5); stkx.push(10); stkx.push(15); stkx.push(20); stkx.push(25); stkx.push(30); stkx.insertAt(-100, 3); stkx.insertAt(-200, 7); stkx.insertAt(-300, 0); //output order: -300,30,25,20,-100,15,10,5,-200 while( ! stkx.empty() ) cout<<"Popping: "<<stkx.pop() <<endl; ///////////////////////////////////////…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
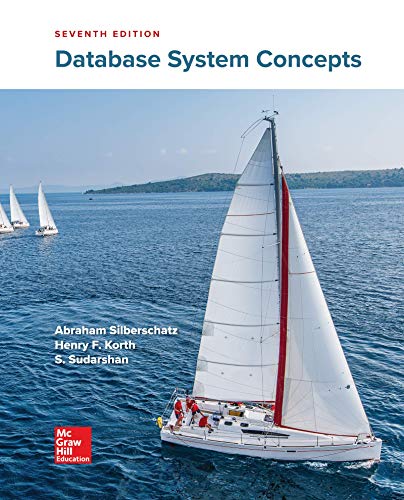
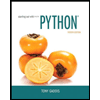
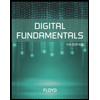
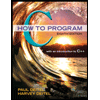
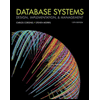
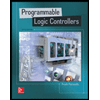