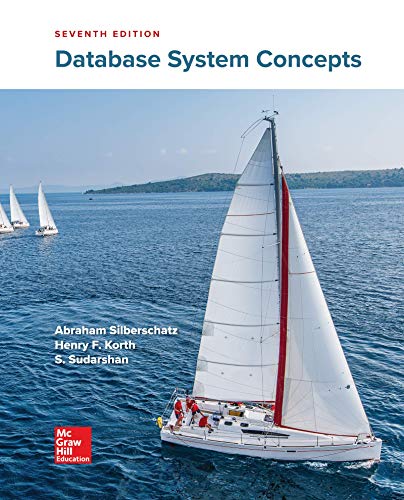
C PROGRAMMING HELP
I'm trying to get a portion of my script to work as a udf inside of a header file that already contains two other udfs that are working properly.
Here is the script with // lines saying the start and end of the portion i want to be a udf in the header file that I will also paste below the script.
Script:
#include <stdio.h>
#include <math.h>
#include "PROJECT HEADER2.h"
int main()
{
// Open the input file
FILE *input_file = fopen("Data.txt", "r");
if (input_file == NULL) {
printf("Error: could not open input file\n");
return 1;
}
// Open the output file
FILE *output_file = fopen("Result.txt", "w");
if (output_file == NULL) {
printf("Error: could not open output file\n");
fclose(input_file);
return 1;
}
//EVERYTHING STARTING HERE
// Read the input file and compute coefficients for each line
int line_count = 0;
double a1, a2, y0, y_prime0, lambda1, lambda2, C1, C2, C_alpha, theta;
while (fscanf(input_file, "%lf %lf %lf %lf", &a1, &a2, &y0, &y_prime0) == 4) {
// Compute the roots of the equation
double discriminant = a2 * a2 - 4 * a1;
// Complex roots
if (discriminant < 0) {
lambda1 = -a2 / (2 * a1);
lambda2 = sqrt(-discriminant) / (2 * a1);
// Repeated real roots
} else if (discriminant == 0) {
lambda1 = lambda2 = -a2 / (2 * a1);
// Distinct real roots
} else {
lambda1 = (-a2 + sqrt(discriminant)) / (2 * a1);
lambda2 = (-a2 - sqrt(discriminant)) / (2 * a1);
}
//AND ENDING HERE I WANT IN HEADER FILE
// Compute coefficients and write output to file based on type of roots
compute_coefficients(lambda1, lambda2, &C1, &C2, &C_alpha, &theta);
fprintf(output_file, "%d %lf %lf\n",
(lambda1 == lambda2) ? 2 : ((fabs(lambda1 - lambda2) < 1e-10) ? 1 : 3),
(lambda1 == lambda2) ? C1 : ((fabs(lambda1 - lambda2) < 1e-10) ? C1 : C_alpha),
(lambda1 == lambda2) ? C2 : ((fabs(lambda1 - lambda2) < 1e-10) ? C2 : theta)
);
display_output(lambda1, lambda2, C1, C2, C_alpha, theta);
line_count++;
}
// Close the input and output files
fclose(input_file);
fclose(output_file);
return 0;
}
Header File:
#ifndef PROJECT_HEADER2_H
#define PROJECT_HEADER2_H
//Calculation function
void compute_coefficients(double lambda1, double lambda2, double *C1, double *C2, double *C_alpha, double *theta)
{
if (lambda1 == lambda2) {
// Repeated real roots
*C1 = 1;
*C2 = 0;
*C_alpha = lambda1;
*theta = 0;
} else if (fabs(lambda1 - lambda2) < 1e-10) {
// Distinct real roots (with rounding tolerance)
*C1 = 1;
*C2 = lambda1;
*C_alpha = 0;
*theta = 0;
} else {
// Distinct real roots
*C1 = (lambda2 - 1) / (lambda2 - lambda1);
*C2 = (1 - lambda1) / (lambda2 - lambda1);
*C_alpha = (lambda1 - lambda2) / (lambda2 - 1);
*theta = atan2(1 - lambda1, lambda2 - 1);
}
}
//Print function
void display_output(double lambda1, double lambda2, double C1, double C2, double C_alpha, double theta)
{
printf("lambda1 = %lf\n", lambda1);
printf("lambda2 = %lf\n", lambda2);
printf("C1 = %lf\n", C1);
printf("C2 = %lf\n", C2);
printf("C_alpha = %lf\n", C_alpha);
printf("theta = %lf\n", theta);
}
#endif
Thank you for your help!

Step by stepSolved in 4 steps

That didn't quite work for me, can you do a demonstration please?
That didn't quite work for me, can you do a demonstration please?
- def wear_a_jacket(us_zip:str) -> bool: This function should use https://openweathermap.org/ API (user account required) to collect its weather information. The purpose of this function is to inform you if you should wear a jacket or not. It will be given a US zip code. This function should query the API to figure out the current temperature in the region associated with the US zip code. If the temperature is less than 60 degrees Fahrenheit (main.feels_like float: This function should use https://openweathermap.org/ API (user account required) to collect its weather information. This function should retrieve the historical temperature in Fahrenheit at a particular US location. You will be given a number of days, hours, and minutes and a United States zip code. The time values given are the cumulative total time in the past minus the current time, you should retrieve the historical temperature (current.temp). In other words, if the parameter days is 4 hours is 0 and minutes is 5.…arrow_forward>> classicVinyls.cpp For the following program, you will use the text file called “vinyls.txt” attached to this assignment. The file stores information about a collection of classic vinyls. The records in the file are like the ones on the following sample: Led_Zeppelin Led_Zeppelin 1969 1000.00 The_Prettiest_Star David_Bowie 1973 2000.00 Speedway Elvis_Presley 1968 5000.00 Spirit_in_the_Night Bruce_Springsteen 1973 5000.00 … Write a declaration for a structure named vinylRec that is to be used to store the records for the classic collection system. The fields in the record should include a title (string), an artist (string), the yearReleased (int), and an estimatedPrice(double). Create the following…arrow_forwardPython S3 Get File In the Python file, write a program to get all the files from a public S3 bucket named coderbytechallengesandbox. In there there might be multiple files, but your program should find the file with the prefix and cb - then output the full name of the file. You should use the boto3 module to solve this challenge. You do not need any access keys to access the bucket because it is public. This post might help you with how to access the bucket. Example Output ob name.txt Browse Resources Search for any help or documentation you might need for this problem. For exampler array indexing, Ruby hash tables, etc.arrow_forward
- Pls help ASAParrow_forwardCOMPLETE TO DO's (do not copy other responses to this question if you do ill downvote) recv.cpp #include <stdio.h>#include <stdlib.h>#include <string.h>#include <fcntl.h>#include <mqueue.h>#include <sys/stat.h>#include <sys/types.h>#include <sys/mman.h>#include <unistd.h>#include <signal.h> // The name of the shared memory segment#define MSQ_NAME "/cpsc351queue" // The name of the file where to save the received file#define RECV_FILE_NAME "file__recv" #define MQ_MSGSIZE 4096 /*** Receive the file from the message queue*/void recvFile(){ // TODO: Define a data structure // of type mq_attr to specify a // queue that can hold up to 10 // messages with the maximum message // size being 4096 bytes // The buffer used to store the message copied // copied from the shared memory char buff[MQ_MSGSIZE]; // The total number of bytes written int totalBytesWritten = 0; // The number of bytes written int…arrow_forwardPlz solve this h.w ASAP C# wimformarrow_forward
- C++arrow_forward# file name: w07_markus.py## Complete the following steps:# (1) Complete the following function according to its docstring.# (2) Save your file after you make changes, and then run the file by# clicking on the green Run button in Wing101. This will let you call your# modified function in the Python shell. An asterisk * on the Wing101# w07_markus.py tab indicates that modifications have NOT been saved.# (3) Test your function in the Wing101 shell by evaluating the examples from# the docstring and confirming that the correct result is displayed.# (4) Test your function using different function arguments.# (5) When you are convinced that your function is correct, submit your# modified file to MarkUs. You can find instructions on submitting a file# to MarkUs in Week *2* Perform -> Accessing Part 2 of the# Week 2 Perform (For Credit) on PCRS.# (6) Verify you have submitted the right file to MarkUs by downloading it# and checking that the…arrow_forwardPlease help with my C++Specifications • For the view and delete commands, display an error message if the user enters an invalid contact number. • Define a structure to store the data for each contact. • When you start the program, it should read the contacts from the tab-delimited text file and store them in a vector of contact objects. •When reading data from the text file, you can read all text up to the next tab by adding a tab character ('\t') as the third argument of the getline() function. •When you add or delete a contact, the change should be saved to the text file immediately. That way, no changes are lost, even if the program crashes laterarrow_forward
- Build Brothers company in C A civil engineering company called Build Brothers has approached you to write a program to help some of their employees with getting their job quicker specially when it comes to calculating different equations in their construction projects. Currently, they use the following PDF to perform all their calculations: http://www.madison-lake.k12.oh.us/userfiles/680/Classes/16192/IED-Review%20Engineering%20Formula%20Sheet.pdf They are willing to develop a small software to calculate all the functions and calculations in the above cheat sheet. They have made this decision to reduce inherent human errors during calculation and improve efficiency. They think that these can be achieved by an “advanced calculator” that allows a user to choose an equation, provide the inputs, and calculate the outputs. Before the company invests on the final product, the have agreed on developing a proof-of-concept first. The idea is that your solution will ‘prove’ that a better…arrow_forwardphp scriptarrow_forwardcreateDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
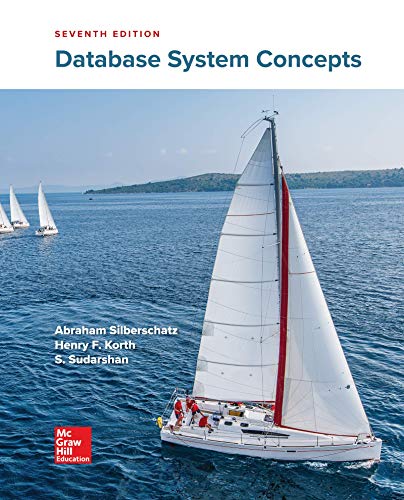
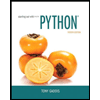
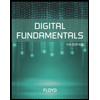
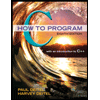
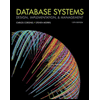
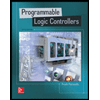