C++ Memory Game Children often play a memory game in which a deck of cards containing matching pairs is used. The cards are shuffled and placed face down on a table. The players take turns and select two cards at a time. If both cards match, they are left face up; otherwise, the cards are placed face down at the same positions. Once the players choose their pair, they can see the board for some time period and attempt to memorize the configuration of cards. They can then use their memory to select the next pair of cards when it is their turn. The game continues until all the cards are face up. Assume that there are two players and to make the game interesting, keep track of how many correct matches each player makes. When all the cards are turned face up, the player with the most matches is the winner. Write a program to play the memory game. Use a two-dimensional array of 4 rows and 4 columns for a deck of 16 cards with 8 matching pairs. You can use numbers 1 to 8 to mark the cards. Use a random number generator to randomly store the pairs in the array. Use appropriate functions in your program, and the main program should be merely a call to functions. Although the game will be written for a 4 x 4 game board and two players, the game may be extended to larger sizes and more players. Write the code in such a way as allow larger boards or more players by changing only a few key constants. For example, a 6 x 6 board would require 18 pairs of card values. Some thought must be given to the data structures needed and how the computer will keep track of the play. The computer must keep track of two pieces of information about each card: the value of the card (an integer between 1 and 8) and its state (face up or face down). In order to keep this information in the 4x4 game board array utilize a struct that has a minimum of two fields, the value of the card and its state. The game must be initialized by distributing 8 values twice in a random fashion in the 4x4 array. There are two approaches to this task. The first approach is to loop twice through the 8 values and generate the row and column indexes randomly. Use a loop that checks to see if the generated location is unoccupied. If it is, it stores the value and the loop ends. Otherwise, the loop continues to generate random rows and columns until an unoccupied location is found. The second approach is to loop through the rows and columns and generate the card values. This approach requires that you keep track of how many times a card value has been generated, if it has been generated and placed twice, continue generating until an unused number has been produced. You will need to write a function to display the game board. If the card is in the face down state, it’s value will be hidden (by showing a default value such as ‘*’ or -1) when the game board is displayed. If the card is in the face up state, the value of the card is displayed. Your display function will also need to display the rows and column values so that the player will know how to enter them on the keyboard. Do not assume that the players will be aware of the offset that programmers use when dealing with arrays To perform the unit test, write a driver that initializes the board and calls the display function to show the values that are stored in the array. Note that for the unit test, all the cards must be in the “face up” state whereas, for the integration test, they will need to be in the “face down” state. It is a good idea to make this initial display configurable so that the integration tester can choose to see the initial configuration before the play begins, but an actual player cannot.
C++ Memory Game
Children often play a memory game in which a deck of cards containing matching pairs is used. The cards are shuffled and placed face down on a table. The players take turns and select two cards at a time. If both cards match, they are left face up; otherwise, the cards are placed face down at the same positions. Once the players choose their pair, they can see the board for some time period and attempt to memorize the configuration of cards. They can then use their memory to select the next pair of cards when it is their turn. The game continues until all the cards are face up. Assume that there are two players and to make the game interesting, keep track of how many correct matches each player makes. When all the cards are turned face up, the player with the most matches is the winner. Write a
Some thought must be given to the data structures needed and how the computer will keep track of the play. The computer must keep track of two pieces of information about each card: the value of the card (an integer between 1 and 8) and its state (face up or face down). In order to keep this information in the 4x4 game board array utilize a struct that has a minimum of two fields, the value of the card and its state. The game must be initialized by distributing 8 values twice in a random fashion in the 4x4 array. There are two approaches to this task. The first approach is to loop twice through the 8 values and generate the row and column indexes randomly. Use a loop that checks to see if the generated location is unoccupied. If it is, it stores the value and the loop ends. Otherwise, the loop continues to generate random rows and columns until an unoccupied location is found. The second approach is to loop through the rows and columns and generate the card values. This approach requires that you keep track of how many times a card value has been generated, if it has been generated and placed twice, continue generating until an unused number has been produced. You will need to write a function to display the game board. If the card is in the face down state, it’s value will be hidden (by showing a default value such as ‘*’ or -1) when the game board is displayed. If the card is in the face up state, the value of the card is displayed. Your display function will also need to display the rows and column values so that the player will know how to enter them on the keyboard. Do not assume that the players will be aware of the offset that programmers use when dealing with arrays
To perform the unit test, write a driver that initializes the board and calls the display function to show the values that are stored in the array. Note that for the unit test, all the cards must be in the “face up” state whereas, for the integration test, they will need to be in the “face down” state. It is a good idea to make this initial display configurable so that the integration tester can choose to see the initial configuration before the play begins, but an actual player cannot.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

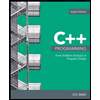
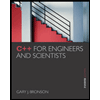
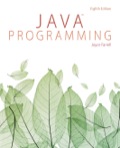
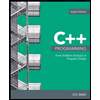
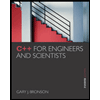
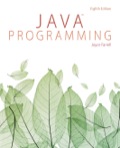