c++ Given two sequences with n integers between 0 and 9, interpreted as two n-digit integers, calculate a sequence of numbers that represents the sum of the two integers. Example: n = 8, 1st sequence 8 2 4 3 4 2 5 1 2nd sequence + 3 3 7 5 2 3 3 7 1 1 6 1 8 6 5 8 8
Q: Q1/ Write a program in c++ which read a sequence of 20 numbers and compute their sum when the sum>=…
A: Algorithm: Firstly take 20 input from the user if the sum of number is greater then 500 then print…
Q: Problem 2 Solvo by Jsva not python A number in base 2 (binary) is a number such that each of its…
A: import java.lang.*; import java.util.Scanner; public class Main{ public static void main(String[]…
Q: #include void main() { int i; int number[11]={12,15,17,3,2,7,10,10,15,15,50}; for(i=0;i< 11; i++){…
A: Over here array is given which has 11 elements and we have to find a Maximum, Minimum, and average…
Q: 1.Write all permutation for ABC. (Consider as a letter A, B,C). (PYTHON)
A: Note: Answering the first question as per the guidelines. Input : String: ABC Output : All…
Q: C++ Write a program to overload the operator ‘+’ for complex numbers.
A: #include <iostream>using namespace std; class Complex{public: float r; float i; Complex(){…
Q: Programming Language: Python 4. Write a Python function that takes a positive integer n as an…
A: Python code for the power of two greater than or equal to given number.
Q: short answers : c)Give an example of a common floating point arithmetic error due to the particular…
A: an example of a common floating-point arithmetic error due to the particular way in which…
Q: Q4: Write a C++ program, using function, to see if a number is an integer (odd or even) or not an…
A: Given: Permutation Check whether the given number is positive or Negative by sung odd or even…
Q: Write a program that takes as input an arithmetic expression. The program outputs whether the…
A: Program code: //include the required header files #include<iostream> #include<stack>…
Q: For a quadratic equation ax2+ bx + c = 0 (a#0), discriminant (b2-4ac) decides the nature of roots.…
A: Required:
Q: C++ to Python. How can I rewrite this code, using Python List Comprehension? Please, help! #include…
A: The problem is about converting c++ code into python code.
Q: Write a C program: The use of computers in education is referred to as computer-assisted instruction…
A: Program approach – Include the essential header files. Declare the variables. Define the function…
Q: Q1 )Write a C program using switch. The program reads one character c. Then it reads one integer…
A: 1) Below is C program reads c and m and according to c calculate value of r using switch statement…
Q: Q2/ Write a program in the Fortran language to read 10 numbers and find the sum of the numbers that…
A: Algorithm: 1. Start 2. Read 10 numbers in an array. 3. Declare s 4. Using for loop traverse…
Q: Please Write a program CODE in C 2. Write a function which takes a string of any length and returns…
A: The coding implementation is implemented below:
Q: 1. Write a program in C that reads the radius of a circle and computes: (a) Area of the circle (b)…
A: 1. Area of Circle = pi * r* r 2. Circumference of circle = 2*pi*r 3. Area of Largest square = 1/2 *…
Q: Write a Python function that will take a positive integer n from the user as an argument and returns…
A: GIVEN: 4. Write a Python function that will take a positive integer n from the user as an argument…
Q: in C++, The result of the following statement is : cout<<pow(3,2) + 5; اختر احدى الدجابات O 13 O 11…
A: pow(3,2) = 9
Q: Write another program that generates another table with the same columns and decimals but by using a…
A: Answer in step2
Q: Rewrite the following code using while loop statement instead of for loop statement in C++.…
A: Rewrite the following code using while loop statement instead of for loop statement in C++.
Q: how do you complete a function that returns more than one value? we were kind of taught it but i'm…
A: Given prototype float minMax (float* array, int size, float* min, float* max); Here other than array…
Q: c++ program
A: #include <iostream>#include <bits/stdc++.h>using namespace std; //Array of first 1230…
Q: In python) Skeleton code for the function count_evens is below. The function takes in an array of…
A: def count_evens(n_array): num_events = 0 for number in n_array: if…
Q: Q1. Write a program in C++ language to obtain the equation below, for values of x from (1-20), by…
A: We will run the for loop from x = 1 to x = 20. Then we will calculate Y. And print the value of Y.…
Q: 2. The Fibonacci sequence is defined as numbers in the following integer sequence: 0, 1, 1, 2, 3, 5,…
A: According to the Question below the solution: Output:
Q: Q7: The Fibonacci Series is: 0, 1, 1, 2, 3, 5, 8, 13, 21, .. It begins with the terms 0 and 1 and…
A: According to the Question below the Complete Program: Program Output:
Q: Please write a Python function that checks whether a passed string is palindrome or not. Note: A…
A: Required: Please write a Python function that checks whether a passed string is palindrome or not.…
Q: 6) Write a C program that take integer value and char (A for area rectangle /P for Perimeter…
A: Solution:
Q: 1. Write a complete C++ program that lists all numbers that are perfect squares in a range. •…
A: ALGORITHM:- 1. Generate the value of low between 50 & 200. 2. Generate the value of high between…
Q: n C program Given a set of numbers representing the customers visiting the store and a number n,…
A: ALGORITHM:- 1. Take input from the user. 2. Count the frequency of the elements. 3. Print the…
Q: In C++ using endl; Write a program that can be used to calculate the federal tax. The tax is…
A: Program: #include <iostream> using namespace std; //function getData void getData(char…
Q: Q1 / Write a program in C++ to calculate the value of n!+((n-2)!)³ (n+2)!
A: Use a function to calculate the factorial of a number and in main we print this result
Q: Write a program in C++ for a simple Calculator which perform Addition, Subtraction, Multiplication…
A: Required: create a calculator in c++ that can perform addition, subtraction, multiplication,…
Q: Q6/ write a number anc or nositive h
A: Code: #include<iostream>using namespace std; // function declarationvoid check(double); //…
Q: Part 4: Write an average-of-three-numbers program This program will get three integers from the user…
A: GIVEN:
Q: Q5) Write a C++ program to find the Fibonacci Series up to n number of terms. The Fibonacci sequence…
A: Write a C++ program to find the Fibonacci series up to n number of terms. The Fibonacci is a series…
Q: In multiplication, assume that leading zeroes are not allowed. Write a program in C++ to help Prima…
A: The answer is
Q: 1. Write a program in C that will read a number as input. Check whether the number is prime number…
A: Since you have asked multiple questions we will answer the first one only if you want an specific…
Q: 2. Let x be the first three digits of your matric number, AND y be the last three digits of your…
A: I am considering the matric number as 2210039 Now x = 221 and y - 039 we change 0 in y as 2 so, x =…
Q: C++ Exercise 30 defines the number e and Exercise 31 shows how to approximate the value of e using…
A: #include <iostream> using namespace std; float cal(int i, int n) { if(i == n) return ((i-1) +…
Q: Refer to the statement below, #include void main() { int i; int…
A: Algorithm: for (initialExpression; testExpression; updateExpression){ // body of the…
Q: PYTHON The metric system uses as a basic measure the meter and the imperial system has its basic…
A: "def feet_to_meters(feet)": "return feet * 0.3048" "def meters_to_feet(meters)": "return meters…
Q: 7. Write a function that evaluates the area of a pentagon. Use "math.pi", for pi, and "math.sqrt"…
A: Area of pentagon Pentagons can be convex or concave, regular or irregular. One with equal sides and…
Q: Write a program that simulates a coin-tossing game. At the beginning of the game, each of N players…
A: please cheek next steps for code and output
Q: Programming Language: Python 5. Write a Python function that takes a positive integer n as an…
A: 1) Below is python program that defines function that takes a positive integer n as an argument and…
Q: Write a program in C ++ to generate 35 random numbers between 1 and then print each 7 numbers on one…
A: Please find the answer below :
Q: In multiplication, assume that leading zeroes are not allowed. Write a program in C++ to help Prima…
A: Below is your c++ source code.
Q: Write a C program to calculate the multiplication of two matrices. Two dimensions matrices have to…
A: Actually, program is a executable software that runs on a computer. C is a high level language.
c++
Given two sequences with n integers between 0 and 9, interpreted as two n-digit integers, calculate a sequence of numbers that represents the sum of the two integers. Example: n = 8,
1st sequence 8 2 4 3 4 2 5 1
2nd sequence + 3 3 7 5 2 3 3 7
1 1 6 1 8 6 5 8 8

Step by step
Solved in 2 steps with 1 images

- (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters to 0, and then generate a large number of pseudorandom integers between 0 and 9. Each time a 0 occurs, increment the variable you have designated as the zero counter; when a 1 occurs, increment the counter variable that’s keeping count of the 1s that occur; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of the time they occurred.C Program to convert Decimal to Binary Decimal to binary in C: We can convert any decimal number (base-10 (0 to 9)) into binary number(base-2 (0 or 1)) by c program. Decimal Number Decimal number is a base 10 number because it ranges from 0 to 9, there are total 10 digits between 0 to 9. Any combination of digits is decimal number such as 23, 445, 132, 0, 2 etc. Binary Number Binary number is a base 2 number because it is either 0 or 1. Any combination of 0 and 1 is binary number such as 1001, 101, 11111, 101010 etc.C Programming Write a program that reads an integer from the user (keyboard), namely n. Yourprogram should print all positive numbers that are smaller than n and whosedigits sum up to a perfect square number.(An integer is a perfect square if it hasan integer square-root for example 25) You should print the numbers in ascendingorder and print their digits separated by “:“ symbol from the least significant digitto the most significant digit on the same line as the number. At the end of eachline you should also print the sum of the digits of the number. Example:If the user enters n : 30, then the output should be as follows:1 1 14 4 49 9 910 0:1 113 3:1 418 8:1 922 2:2 427 7:2 9
- c++ Given two sequences with n integers between 0 and 9, interpreted as two n-digit integers, calculate a sequence of numbers that represents the sum of the two integers. Example: n = 8,C++ Given a positive integer, N, the ’3N+1’ sequence starting from N is defined as follows: If N is an even number, then divide N by two to get a new value for N If N is an odd number, then multiply N by 3 and add 1 to get a new value for N. Continue to generate numbers in this way until N becomes equal to 1 For example, starting from N = 3 the complete ’3N+1’ sequence would be: 3, 10, 5, 16, 8, 4, 2, 1 Write code to ask the user to enter a positive integer (N) in the main() function. Write a function sequence() that receives the integer value N and display the ‘3N+1’ sequence starting from the integer value that was received (entered by the user). The function must also count and return the numbers that the sequenceconsists of. The returned value must be displayed from the main() function.#Solve it with C programing Mr. X is a student of Computer Science. He is facing a problem and needs your help to solve it.The problem is, you will be given N integer numbers( 4<n<=100) which are arranged side by side (x1,x2,x3,x4,.....).Now you have to sort those items in ascending order. After doing this , your job is to print the items in a sorted way and find the median.[NB: when N is odd , Median= (N+1)/2; When N is even Median= (N/2)+1 ] Sample Input: 74 7 11 2 9 3 5Sample Output:2 3 4 5 7 9 115
- A Palindromic prime is a prime number that is also a palindromic number. Write a C++ program that displays all the palindromic prime numbers between 100 and 999 without using pointers.For example: These are 14 palindromic prime numbers smaller than 500:2,3,5,7,11,101,131,151,181,191,313,353,373,383.Armstrong(Narcissistic) number is a number that equals to the sum of the digits that powered by to each the number of the digits. For example: 407 is a Narcissistic number because it has three digits and, 407 = 4^3 + 0^3 + 7^3 = 64 + 0 + 343 Find all 3 digits narcissistic numbers by C or Python.CODE USING C++ We've already tried comparing 3 numbers to see the largest among all, so let's try a more complicated mission where we locate where among the 5-digit integer is the largest one. There are only 4 possible cases for this mission: if the largest digit is the first digit, print "Leftmost" if the largest digit is the third digit, print "Middle" if the largest digit is the last digit, print "Rightmost" if none of the above is correct, print "Unknown" Now, show me how far you've understood your lessons! Input A line containing a five-digit integer. 14632 Output A line containing a string. Middle
- Java LAB #4 One of your colleagues wanted to generate an List of strings using a random function, and since you are an expert in programming, your colleague asked you to create a program for him that generates random letters to make this desired string (i am a programmer) how can you help him to achieve this task ? Notes: • string length must be lesser than 100 ascii code allowed range from 32 to 140 demonstrate a condition that fix the correct letter for each generation Ex: 1* gen is a profssmagdgaer, 2st gen in a progsanger -- Final gen (Im a programmez) use Arrayl.ist to make the random permutations print number of generation that makes the correct sentencePython code Write a function that, given two integers, return the product of every integer between them, inclusive. #Example function code: #def circle_area(radius): #area = math.pi * radius ** 2 #return areaC PROGRAM Write a program that asks the user values for the coefficient A,B, and C in the quadratic equation Ax^2 + Bx + C= 0, and then prints the solution/s of the equation (if there is/are any) For this problem, you need to include the following header file: math.h So that you can use the C library function sqrt() The function sqrt() computes the square root of a value (in double) Use #include <stdio.h>
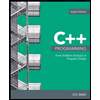
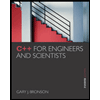
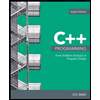
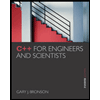